Drizzle/better-auth + Local PSQL
I have the biggest issue trying to setup drizzle with my project and I don't believe it should be this complicated as I am facing currently. Someone with more experience please help me out and explain to me what I am doing wrong.
So I am trying to setup better-auth with Drizzle + my local PostgreSQL and I am using the latest version of Next js for my project.
I basically start of my initialising my next js project by running the regular command
npx create-next-app@latest
(app router no src-folder) and go then install drizzle following the docs and run the commands that are shown in the docs/guide. I create my .env
file and setup my DATABASE_URL="postgres://postgres:mypassword@localhost:5432/postgres"
and move on to creating my db
directory and place the index.ts
file inside of it which holds this code.
And I then create my schema.ts
file. This is basically just copied over from the docs. Afterwards I move on to create my drizzle.config.ts
file but I slightly modify it.
What I change here is that I change the schema: './src/db/schema.ts',
to schema: './db/schema.ts',
for app directory in next js.21 Replies
From this point on I sort of move into the better-auth installation part of their docs and do the steps that are. I run the command of
npx @better-auth/cli generate
which gives me the back a auth-schema.ts
file. That sort of looks like this
I then try to run this npx drizzle-kit migrate
to migrate things over and I get this error.
did you reference this new schema file in your drizzle config, and did you run npx drizzle-kit generate first, to generate the migration sql, before running drizzle-kit migrate
what you saw isnt any error, it just didnt migrate anything
I bascially took what ever was in the
auth-schema.ts
and and placed it inside of the schema.ts
file. I don't really use the other file. It's only usecase was to generate the info shown above.
Yes I did run those commands and when I run the npx drizzle-kit generate
it says No schema changes, nothing to migrate because I have already ran it before. This only changes in case I delete the drizzle
directory inside of my project.Also if I open up pgadmin to see what's inside the db I get 2 schemas. One named public (which I'm used to seeing from other projects) and a schema named "drizzle". Should this be the case?
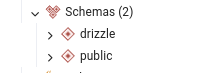
yes, drizzle keeps track of migrations
so whe nu ran generate it didnt generate a sql file in drizzle/migrations?
Do you mean inside pgadmin itself?
no in your project folder
Oh yeah. As I said earlier it does generate that directory
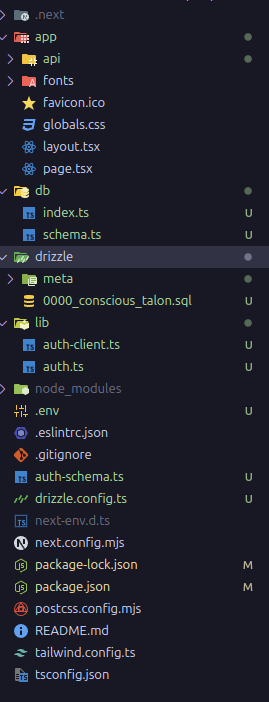
ok and whats in that sql file
ok now in your db whats in the drizzle migrations table
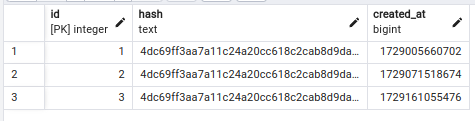
so you are saying that none of these tables are created in public
No they are created. I can see the tables. I am curious to why it's giving me the error in my terminal when I run the command
npx drizzle-kit migrate
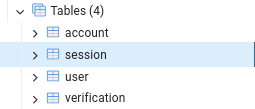
thats not an error
its telling you you didnt pass in a config file so its using default one it can find
What does that mean exactly.
the config file is how to connect to your database
the one you made at the top
in teh command line you didnt pass it as an argument so it searched your project for one and used the one it found
So where can I find more info about how to do that exactly because I mostly just copied over code from the docs. I now need to understand exactly how it works
Basically what your saying is that my
index.ts
file should be passed into my drizzle.config.ts
file right?no
you do not need to do anything
everything worked as it should
Okej then
Thanks Darren 😄