class questions
Hey, i have a few questions about classes given this code:
1) why does the
this
variable need to have a different identifer (in this case _) to prevent it from giving this error? "Uncaught TypeError: Cannot set property message of #<Example> which has only a getter
at new Example"
2) if i try to use a function that isn't a getter to return a this
value, like below, it returns "ƒ getMessage() { return this._message; }"
rather than "example message". Why is this? Is there anything like this with setters too?
3) why may you want to store a class in a variable? e.g. const x = class Message {...}
Thanks in advance.10 Replies
for your 2nd question, it does return the msg if u call the getMessage function tho
it returns a function if u dont call the method i.e u dont add
()
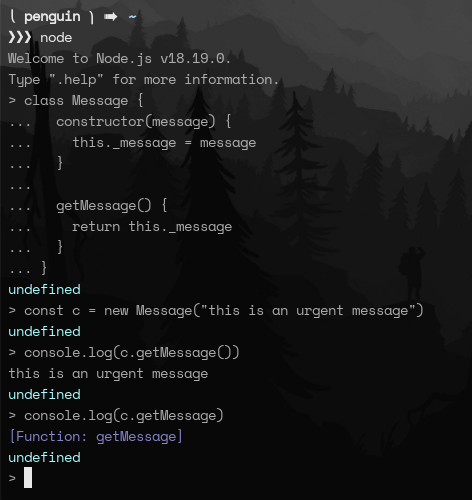
doing
console.log(c._message)
also works
btw, getter and setters r normally used for private props
private props r properties that are not accessible outside the class itself
so u provide with a getter and setter method
for your 3rd question,
when u construct a class, it basically creates an object so like
{key: value}
which would be the copy of the base class except with its own set of values and returns u the reference to that object, u basically store the reference to that object in the variabke
otherwise how would u refer to that objectFor the first question:
Using the getter syntax there, you defined a property called
message
, that doesn't store its own value but instead returns the result of running the getter whenever you try to access it. It's kind of a different way of writing it as a function, except you don't call it yourself, the code does it for you.
Because it still has to store the value somewhere, you need to use a different name because message
is already taken. The underscore is a pretty common convention to indicate that a property should be treated as private, and not accessed directly, so the example used an underscore to differentiate the actual stored value of the message and the getter function message
It could just have been called "myMessage" or "text" or "Jeremiah".
usually you'd use a getter or a getter/setter combo if you wanted to do some logic, or use some kind of caching. Say it's an expensive fetch command to actually get the message, and it's not used very often, you'd use a getter to check if _message
is undefined, and then fetch the result if it's not, store it, and on subsequent uses, just return the cached value
they're also used for computed values, so like... lets say you have a class that stores someone's height, and you store that height in centimeters. You may want to have a heightInInches
or something, because you have a multi-region piece of software, but you wouldn't want to store a value that's dependent on a different value, because then you might forget to update one when you update the other, so you'd put some logic in to turn centimeters into inches. Very quick and dirty:
Thank you both for the info, it’s super useful.
So essentially you can create an instance of the class by either doing
let x = new Message()
and x = class Message {…}
?
Also with private properties, I thought that’s what static
was for in classes? Or is that different?private properties are properties that are only used internally in the class, static means the class doesn't need to be instantiated to be used
You don't get to call the constructor if you use x = Message. But I'm also not super familiar with defining classes in Javascript, I don't do it that often
Oh okay I see, what do you mean by instantiated? Does that mean that things with
static
written infront of them don’t pass down to a class that extends the original?in most languages, when you write a class, you can have static and dynamic methods. Static methods can be called without having a class instance, where dynamic ones need a class instance (so an object, rather than a class)
like I said though, I'm not super familiar with how JavaScript does it, because Javascript OOP is a nightmare compared to other languages
I mostly just consume objects and classes from libraries
I really struggle with classes and OOP in general myself. Just wanted to add on , a class instance is when you use it eg
So the instance is now
user
, it is the first instance and you can have as many as you want.from your pattern, you seem to be trying to create a private variable
dont do it this way: add
#message = null;
before the constructor, and then access to the value with this.#message
its fugly, but thats how you make a private variable
and these are really private, while what you have is just a cheeto lock: hopes and dreams are all that keep it "private"Ah okay I see, thank you all