Improving performance / caching
Hello, Im coming to an end with my database software. I filled the database with around 150 rows, but now I got the problem that performance is really trash with everything it does, even if it only does single row querys. So I thought maybe it´s possible to create a cache which stores all data once they are loaded which then is being used by all the other methods making a query.
This is the code where all aircrafts are being collected, at the moment the data is loaded asynchron to not block the UI.
How can I build such a cache with is used by every method?
36 Replies
Oof, doing some manual ADO stuff, my sincere condolences
For caching, there's https://www.nuget.org/packages/Microsoft.Extensions.Caching.Memory
I believe that's what ASP Core uses under the hood for it's caching
All that said, though, you should really not be having any performance issues with a database this small
each row got a picture in it with 5mb
Why the hell are you storing images in the database
where else xD
In the file system on disk?
Or in a CDN?
Then you just save the path in the database and you're good
but its on a local server
How does that change anything?
yeah, good question
I thought this would be the best solution :harold:
So shall I scrap it or does the caching also works with the pictures?
Caching is a solution for a problem that isn't there
what would you do?
I would store the images on disk or in a CDN and store just paths to them in the database
Or, in case of a CDN, whatever additional ID or whatever is necessary
okay
Thanks
I approve of what Z said
Huge amounts of data (images, arbitrary binary data, big amounts of texts, etc etc) make databases slow
And relational databases are very very expensive to host
So it's just not a good practice
https://www.microsoft.com/en-us/research/publication/to-blob-or-not-to-blob-large-object-storage-in-a-database-or-a-filesystem/?from=https://research.microsoft.com/apps/pubs/default.aspx?id=64525&type=exact
Is a good research paper talking about disk (blob storage being just one way of saving a file on a disk) vs database storage of files
Can you help in me in doing this, I literally builded the whole thing around storing the pictures in the db and dont know how to start now over with this again :sadge:
and what if the path changes, eg i buy a new PC
You use relative paths
Paths starting with
./
for example, will be relative to where the .exe
isSo, I Change the datatype in The DB to varchar and create The Method above and just call it whenever I need to interact with a Picture?
In the db you change the column from
byte[]
type you were storing it as
To an int Foreign Key, that points to a File objectSo shall i create a table and link it to The main table with a foreign Key, The Table then only Stores a path
yes
The file class i gave as an example of what the table would probably look like
Why not use the current table, instead of The Picture use a string
With a separate table for files, you'd have a centralized management point
Of course, you can scatter information about the existence of a file around the table, but it can and will in the long run hurt integrity
Things will get lost, files will be deleted, and various tables need to be updated extensively
A good database design will teach you a thing or two about good practice :catlaugh:
So this is my current database
aircraft:
airline:
picture:
You got any further improvments?



I usually don't prefix/suffix the primary key with table name
It's useless information IMO
But besides that, LGTM
So thats basically the new table I created now

Okay, so im now copying the file to a directory in the project folder and the database is looking like this now


Is this correct (Besides the suffix)
So its saving the picture files, but I just cant get it to work so it shows the picture again in the pictureBox, it just shows an empty box without any error
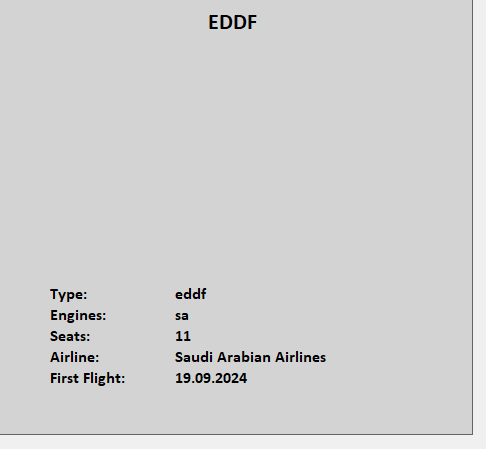
Thaat is up to you to fix now
Okay, so im pretty much done now, I got one last problem
Basically when Im deleting a picture it says that its being used by another thread and so it cant delete the picture
I already set the pictureBox NULL where the picture is in but it still says that its being used
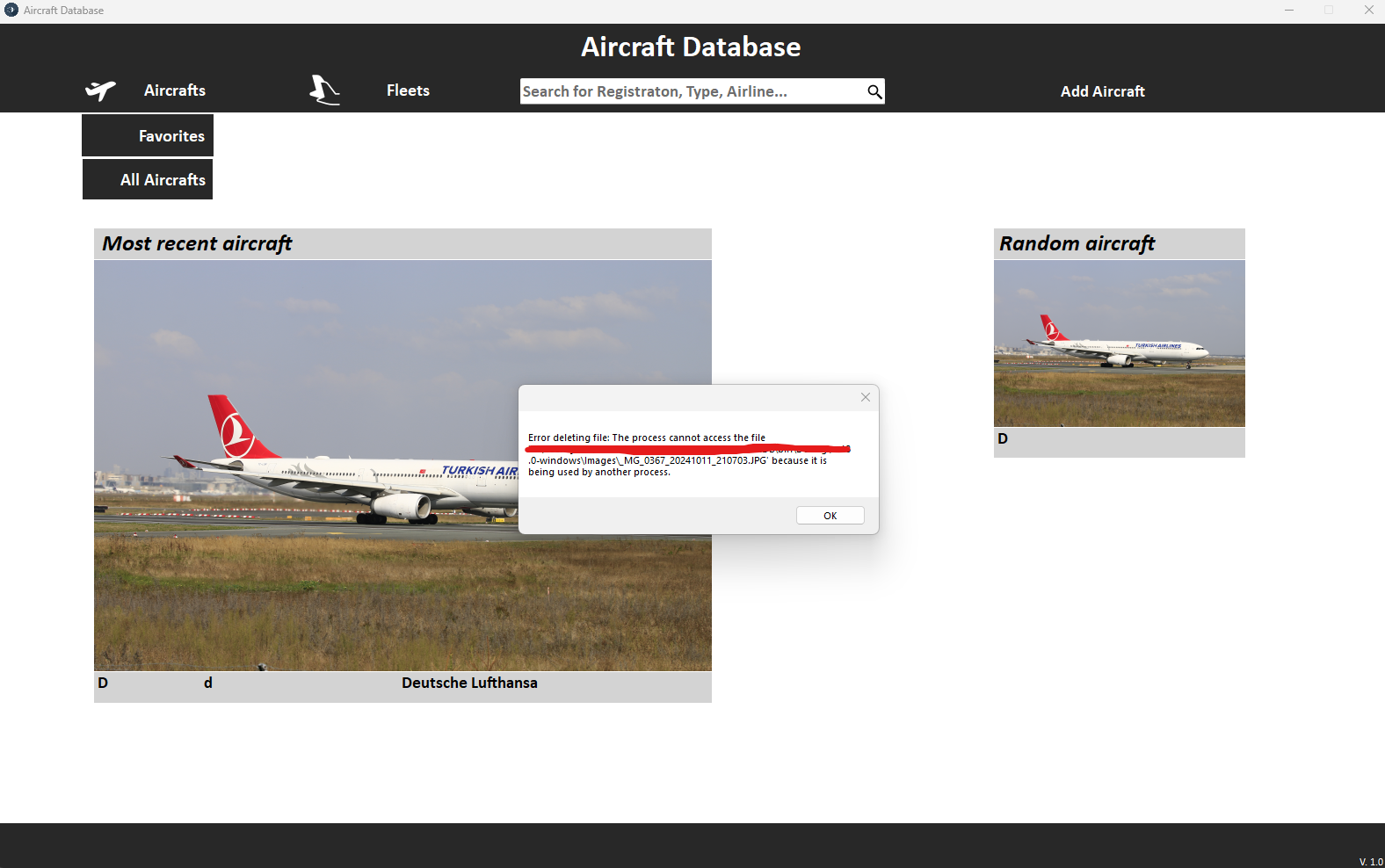