use strict & data storage
Hey, i have a couple of questions:
1) I understand
"use-strict";
is used to prevent mistakes and stop bad code from having a chance of running. Does anyone have any examples of something that is considered bad code running without the use of use-strict
and then it not working when you add it?
2) let's say you have a tab system (see image for example). When you click on a different tab, the html changes to content relevant to the tab. Where is an appropriate place to store the data (text, image etc.) before inserting it to the page? I'm aware you may do this by changing .innerText/.innerHTML
values and the value of the src
attribute for the image, but storing them in variables or something doesn't quite feel right, though i may be wrong.
I'd appreciate any insight. Thanks in advance.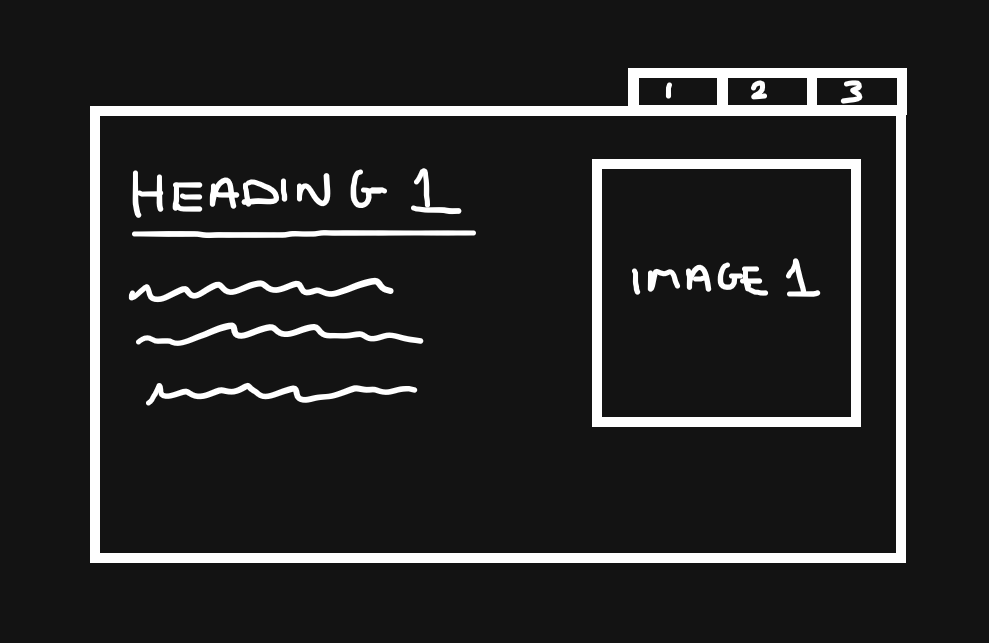
22 Replies
Ello. I'll try to give you some answers.
1. The use of use-strict will prevent you from doing silly things like having multiple variables declared with the same name. An example is
Javascript allows you to do this but with strict mode an error will be thrown. https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Strict_mode
2. The data for the different tabs should be in the html using css/js to hide show the content. If the data is consistent and known beforehand this is the best for accessibility reasons.
If you're fetching the tabs dynamically then you could use the innerText/innerHTML to swap out the contents however there is additional overhead on the server and client to make this happen.
MDN Web Docs
Strict mode - JavaScript | MDN
JavaScript's strict mode is a way to opt in to a restricted variant of JavaScript, thereby implicitly opting-out of "sloppy mode". Strict mode isn't just a subset: it intentionally has different semantics from normal code. Browsers not supporting strict mode will run strict mode code with different behavior from browsers that do, so don't rely o...
"use-strict"
doesn't do anything
"use strict"
prevents you from suffering from surprise mistakes by throwing more strict exceptions when you do something dummy
the difference is extremelly important
another example of strict mode working: assign a value to NaN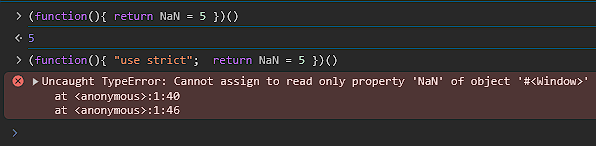
for #2 ...
do not use
innerText
/innerHTML
for that
both properties are a performance nightmare
if you need to fetch content dynamically, use either:
- the dom parser api (recommended)
- use a <template> and add the dom parsed elements into the correct element (recommended)
- use a <template> and set the innerHTML
of the correct element
- create a document fragment or an element, and feed it the html in the same way
the difference between using innerHTML
and the dom parser is that innerHTML
will execute scripts and download images imediatelly when added to an element, while the dom parser lets you manipulate the elements safely before you add them into the document
also, an important difference: innerText and innerHTML can cause performance issues, specially if you change it a lotah okay i see, all of this helps, thanks a lot 🙂
with this, let's say i wanted to switch a heading from "heading 1" to "heading 2" with the click of a button, you'd recommend something like this rather than swapping the
innerText
of the h1?
if you need to set text, use
textContent
https://developer.mozilla.org/en-US/docs/Web/API/Node/textContentWith templates you don't need to create anything, you clone them and then define the content
So you would have
when you clone the content of a <template>, you get a copy of the elements
you then can use the clone as if it was the
document
, in most instancesah right i see, i'll look into that, thanks you both
you will also then need to append it to the DOM once you have updated it's content with your values.
alright, that makes sense
this is why i love templates
Me too!
I much prefer them to generating all the HTML in the JS file.
same, it's so practical
and all the html stays in html
Me three but I need a post-it on my forehead that it’s
not
I always make that typo and waste two minutes debugging why it’s not working. So dense sometimes 🤦🏻♀️
🤣
i know the pain
doesnt iframe use content?
Glad to hear I’m not alone ; I literally facepalm every time. Not sure as I’ve used an iframe maybe twice but have a project coming up where I do have to use one so I’m sure I’ll find out! 😆
🤣 you will suffer
but you know what helps a lot?
jsdoc or typescript
Never heard of jsdoc but I am learning ts and it will be one of the reqs on the upcoming project as will react
you will need jsdoc anyways, to document the code
it's just comments, but with specific "tags"
if you google it, you will find the documentation about it really easily
Yes I’m looking it up, very interesting!
automatic documentation and all you need to do is write comments