Pipe chains don't work in rc13
I'm trying to make a logging wrapper to help me debug my Types
but pipes don't seem to chain
18 Replies
@ArkDavid
What version of this? I fixed something similar in the last release
It says 13
How can I know version in runtime?
Is there
type._arktypeVersion
or whatever?
Serious question
Would use it all the bugs
Anyways add this test pleaseNah it fails on rc14
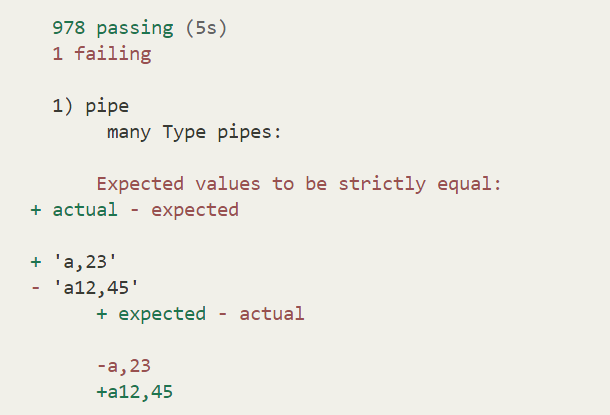
Please ping me when you fix this, I think I'll pull the fix
Since this works:
It looks like an incorrect optimization where we notice that last morph and the next pipe are
===
and ignore it or something
May also have to do with the input being validated over and over I guess
Yeah I guess it is the validated input
This works:
Yeah I'm on it like I said it has to do with piping to another node incorrectly ignoring repeated pipes
Hmm, interesting, only repeated ones?
Okay now I understand nothing atall
anyways, my LogWrapper doesn't work so (╯°□°)╯︵ ┻━┻
I think so, like I said it has to do with normalization because there's a bunch of logic so that e.g. the second
to
can be ignored in type("string.json.parse").to({name: "string"}).to("object")
I'll fix thisBut with
(v) => T(v)
it does work so ┬─┬ノ( º _ ºノ)There's a lot of complex logic around pipes and tons of edge cases hopefully at some point I will cover all these
That's because AT doesn't understand what that does
It's just like any other function
Whereas if it's another type, we can reason about it and reduce things
Yeh lol I think you don't even have a test for multiple pipe args
Shouldn't matter really as long as it'
s right for the base case
Internally there is just a
pipeOnce
function that gets reducedThe base case with multiple arguments should be checked anyways
But there are still a lot of edge cases with morph nodes, unions, metadata etc.;
These are the cases I'm adding + fixing (both broken now both in terms of structure and result):
You shouldn't test as it's a whitebox
Thanks for the 101 lol
There's a reason everything is tested through the top-level type API
But you have to reason to some extent about what logic actually needs to be tested
E.g. if you are a library that wraps arktype, not testing the entire parser requires the white box knowledge that you didn't implement it
These are fixed in
2.0.0-rc.14