Weird errors when using useQuery
Maximum update depth exceeded. This can happen when a component repeatedly calls setState inside componentWillUpdate or componentDidUpdate. React limits the number of nested updates to prevent infinite loops.
13 Replies
cc @dan
first snippet gives me a wrapper Providers which I wrap
children
in layout.tsx
this looks fine. can you send a compoentn that infa rerenders
this? https://discord.com/channels/966627436387266600/966629731086774302/1289596654235684967
also, using
useQuery
now doesn't fetch my data at all for some reason
no fetch request is being sent
oh it fetched now, after a long time
I think that was because it got set as stale
ah, it's the initialData
>:(@dan I think setting the default value like this is causing the error
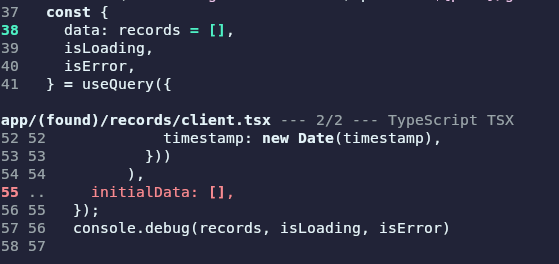
yea because you're ssring and not throwing it doesnt refetch on the client after since you're hydrating with the servers cache on the client
once its stale it'll then refetch
it was because of the
initialData
Maximum update depth exceeded. This can happen when a component repeatedly calls setState inside componentWillUpdate or componentDidUpdate. React limits the number of nested updates to prevent infinite loops.this is still happening :(
and removing the useQuery stops that from happening?
yeah, I don't see that error with
useSuspenseQuery
The diff between the two is quite minimal
me setting a default here seems to be the most relevantfull diff
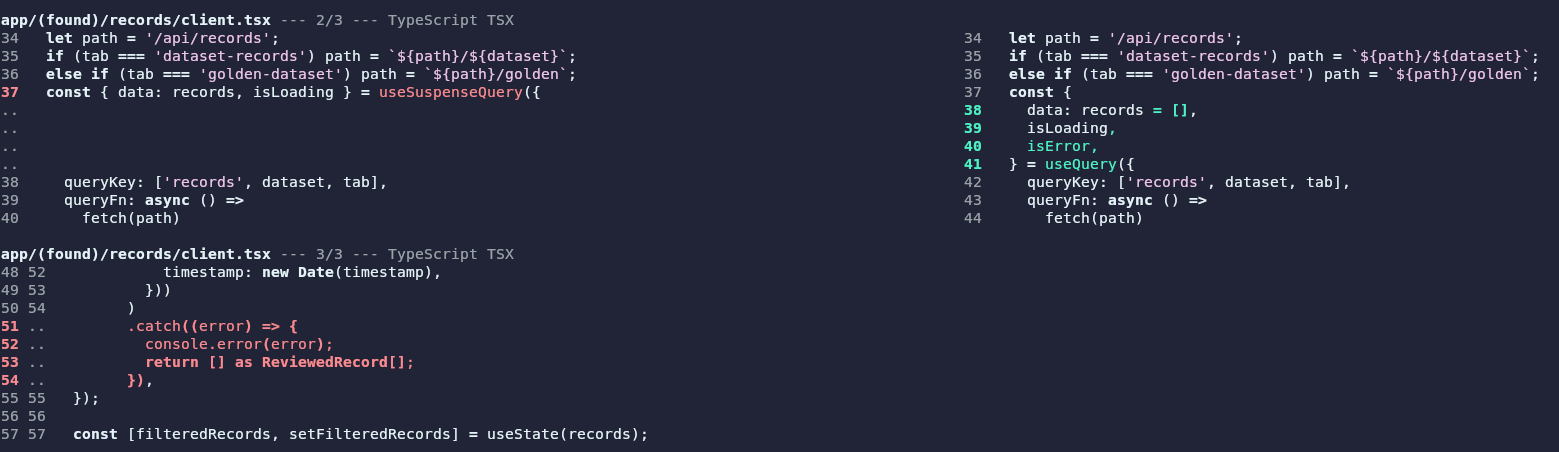
setting the default on the useQuery like that wont work afaik, make another variable lile
im not sure if that would cause the rerender issue though :Thonkang:
this fixed it lol
so weird
You can ask on the Tanstack discord and see if dominick or tanner has a good answer
Right! Thanks :)