Add roles / permissions per team for user
Hello,
I have a case where I use spatie permissions, and I want to be able to give user roles and permissions on a team, can someone help with with the set of relations I have to do?
I have:
User - model
Team - Model
TeamUser - Model
But I get an error when I try to save the team, when I select user roles
This is how I was thinking to make it using repeater,
Any help would be really appriciated.
Thanks
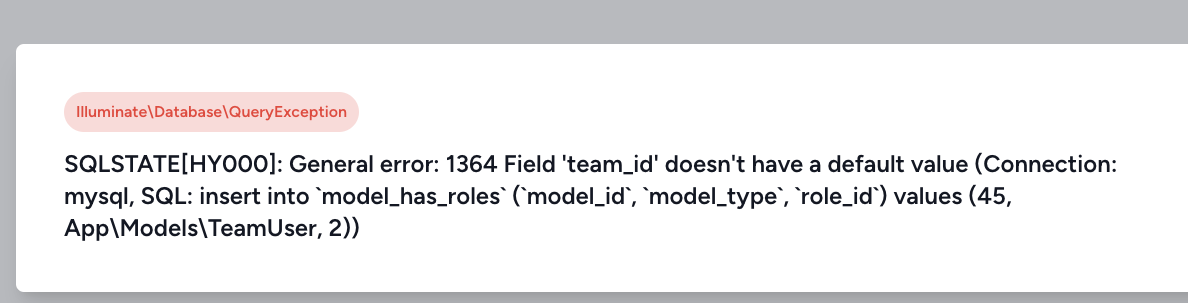
26 Replies
try to make the column
team_id
nullable in the db
and see if that fix it
for more:
https://filamentphp.com/docs/3.x/forms/advanced#saving-data-to-a-belongsto-relationshipDo I also need to remove team_id from primary I suppose also right ?
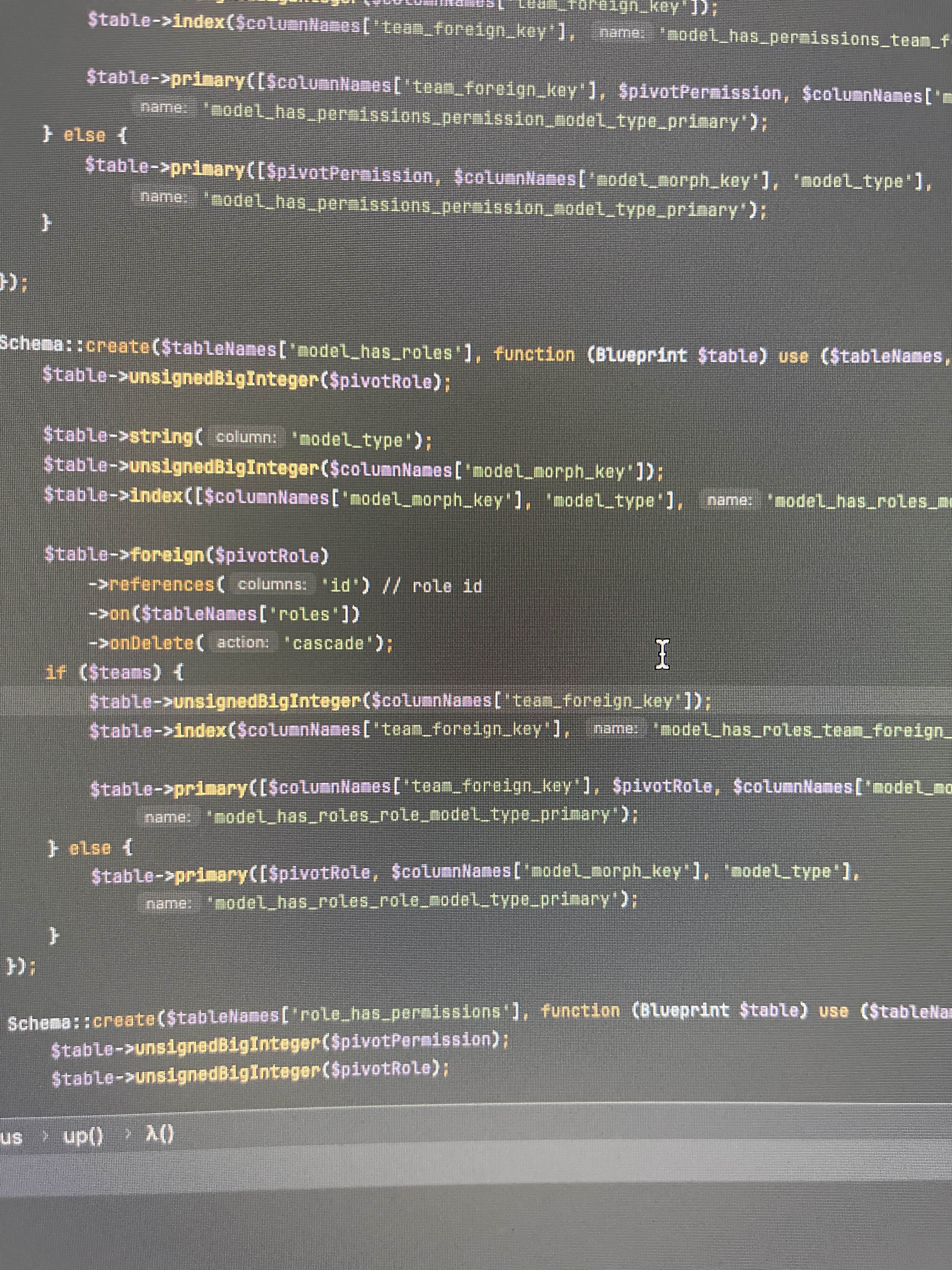
yes, I add a new migration to do so
but you can test and edit it directly on the db see if this solve the issue
The sql error is solved but team_id field remains empty
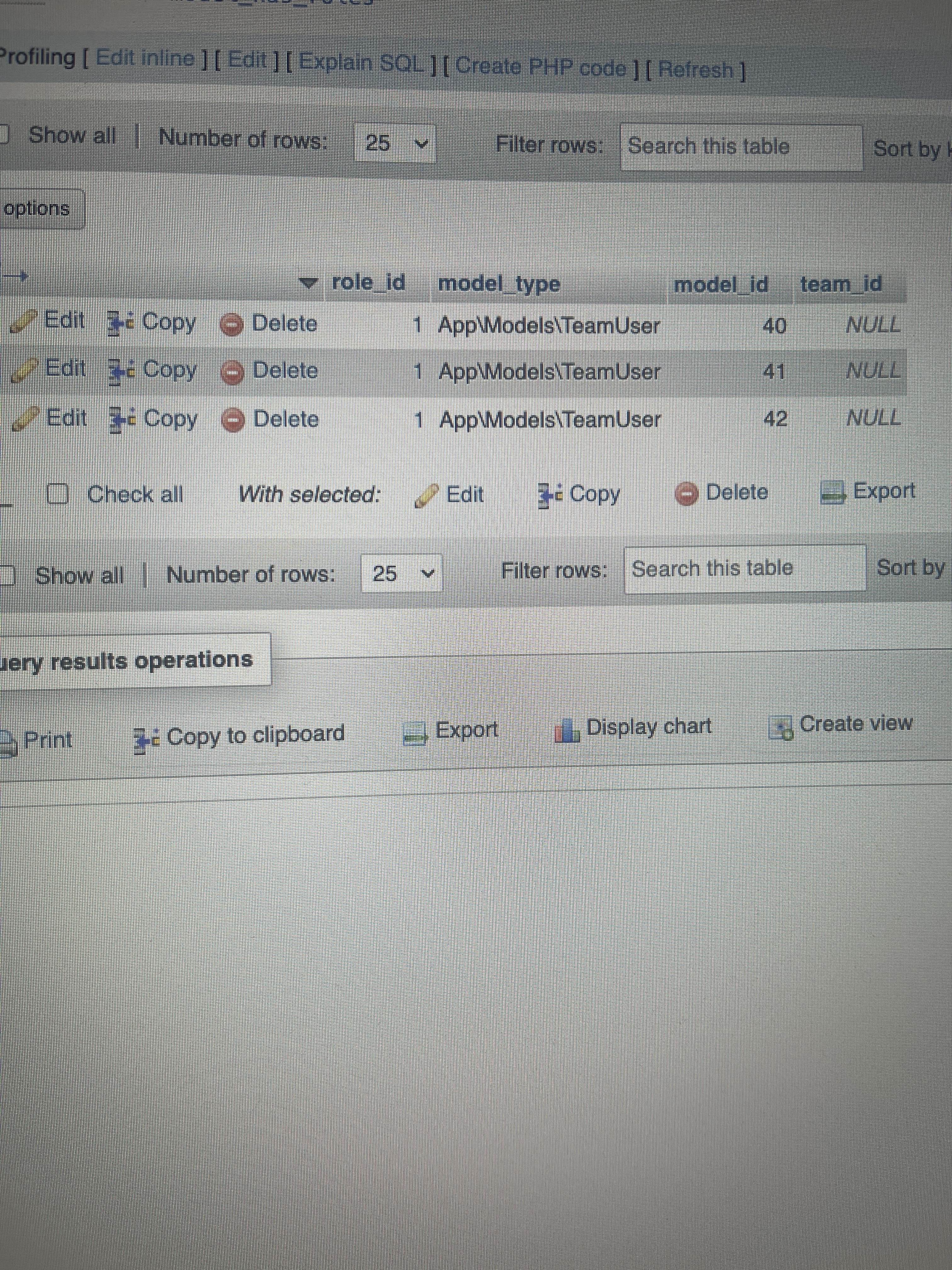
this from my user resource, so adjust the code as needed
but this to give you an idea
you can
dd(getPermissionsTeamId())
to check if the team id is set correctly also you can use Filament::getTenant()->id
@Lara Zeus i get no luck I get this error when trying to save
SQLSTATE[23000]: Integrity constraint violation: 1062 Duplicate entry '1-52-App\Models\TeamUser' for key 'PRIMARY' (Connection: mysql, SQL: insert into
model_has_roles
(model_id
, model_type
, role_id
) values (52, App\Models\TeamUser, 1))
this is my form
As long as you have the right relations set up, like users being part of a company and a company having multiple users, you should be good.
I use #bezhansalleh-shield with multi-tenancy works pretty good.
Thye state multi-tenancy isn't supported...
Yea mean spatie?
GitHub
Support Multitenancy · Issue #382 · bezhanSalleh/filament-shield
Any chance we add multitenancy near future ?
Im using it on the "employee" panel, but on my "customer" panel it also works? It all depends on your policies, right?
do you guys think is oky this relation I have in my User mode?
do I really need that TeamUser ?
from what I see the problem starts here:
i saves me the roles in the model_has_roles table, but it doesn't insert the team_id value, which is actually the record->id that i'm currently editing..
That makes sense, right? Since it's a pivot table, they just assign the role to the user, correct? Now you need to figure out if you can add another value to a column before saving it.
saveRelationshipsUsing
@Lara Zeus using saveRelationshipsUsing on the repeater, does nothing
trying to place a dd right after
it goes by and inserts a value in model_has_roles with team_id missing
GitHub
Mutate data before saving to a pivot table · filamentphp filament ·...
I have 3 tables: client, contact and client_contact, client_contact being a pivot table. Alongside the ids of client and contact it should save additional data like the current user's id. But I...
Is this something that can help?
managed to catch something when I did
i tried this also
but no joy, somehow the model_has_roles is getting the values inserted without team_id before even to reach my each statement and I get sql duplicat id error
@CodeWithDennis @Lara Zeus any idea ? 😢
have you tried setting up setPermissionTeamId($team_id) before setting roles?
yeeye i think i sorted it, now it saves correctly my
replaced saveRelationshipsUsing with saveRelationshipsBeforeChildrenUsing
@CodeWithDennis @Lara Zeus i've managed to eliminate that TeamUser, the question i have is,
I want to have a super admin panel, where you can see resources from all Teams, the problem I see rising is this Spatie Permission relation
as you se it relies on getPermissionsTeamId() which you normally set when you switch the team, any idea how I can make it work in super admin panel so it brings the correct things?
No sorry. 😅
managed to sort it in the end, even simpler :))
@jamesro do share the solution here please, I might need it to make it simple as well.
this is what I've ended doing:
Thank you so much.