Design Patterns / Factory Method
I'm having some serious issues understanding what the Factory method does. We were given an exercise to learn it but i'm completely lost on how / what it is that i have to do.
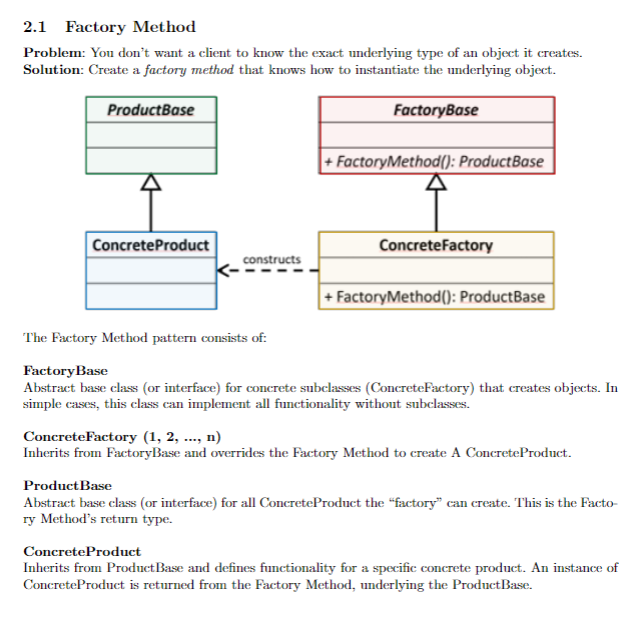
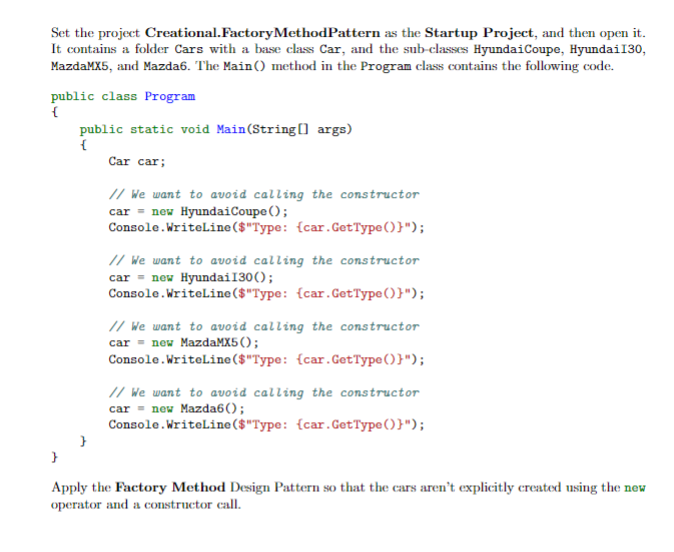
71 Replies
Like specifically the picture they provide doesn't really make sense to me.
So far the only thing i know is that we use the "Factory Method" such that we don't instantiate a constructor directly into main. But we let the "factory" (w/e that is) do it for us.
So what exactly are we meant to do in main? Call an interface/abstract class which in turn calls something?
in main, you call your factory method. This gives you the object the factory created.
There was a pretty good example at the end of your last thread
I sadly don't have my own thread open
Or can't find it rather
ok, here is an example
we have a
Product
class that has 3 properties: Id, Name and PriceAnd this is all inside main?
no, just the stuff before
public class ProductFactory
we want Ids to be assigned automatically, so we make a factory that keeps track of IDs
we then create instances of products via the factory, and they automatically get their IDs assigned and incrementedOk. So we create an object from
ProductFactoru()
.
I'm not sure what this does thoit creates a list
it makes 3 products via the factory and adds them all to the list
I see. Is the list an array?
its a List.
Never seen
List<Product>
Syntax beforeI know of all the basic ones like array, linked list, stack, heap etc..
now its an array.
happy? π
Yeah that makes sense
Just wasn't aware c# had it's own type of list
a
List<T>
is a smart wrapper around an array, and is used for when you dont know the size of the list ahead of time
it uses an array internally, but has a lot of helper methods to automatically resize the array when neededI see. Couldn't we just define the array like you did
Product[]
. This makes the Array scale with input?
If the purpose of List<T>
is used for when we don't know the size of the array. Shouldn't Product[]
do the same thing?
Also where is the abstract class / interface in this example?there is none.
So the factory method doesn't always have an interface/abstract class?
no.
you are probably mixing the two patterns up again
:harold:
there is a pattenr called ABSTRACT FACTORY
and one called FACTORY
:p
Oh. I had no idea that was the case
But it seems this exercise is asking to use the Factory Method
So what are the differences between these classes?
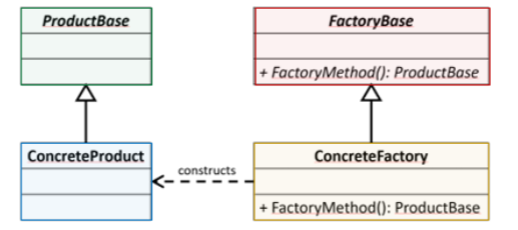
that picture is for the abstract factory
Because in my exercise we have two type of brands of cars. Hyundai and Mazda. And these two brands have 2 types each. HyundaiCoupe, Hyundail30 and Mazda6, MazdaMX5.
So would i need two types of factories one for Hyundai and one for Mazda?
yeah
welllll
it depends on several things
Really? The exercise is described as "Factory Method" and it says
FactoryBase
Should be an interface/abstract methodyou could in theory just have a single factory with 4 methods, but thats not very scaleable
FactoryBase is literally an abstract class, so yes.
Ok. And this abstract class should contain the two Concrete Factories? Which is Mazda and Hyundai
no
it doesnt contain them
they are subclasses of it
Yeah sorry meant subclass
And these subclasses are the one that makes the cars?
yes
And that is essentially it?
they are the
ConcreteFactories
Aah i see!
And they make the
ConcreteProduct
?yes
What is the
ProductBase
For?
for the abstract factory to know what to make
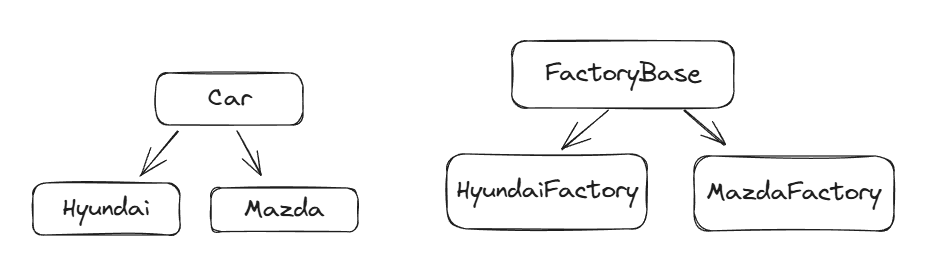
you can see how the two structures mirror eachother
Ah yeah
So the right one is An abstract Factory and left one is a normal Factory?
>_>
the left ones are the products
the cars
the right ones are the factories
the top one is the factoryBase, the abstract factory
the bottom ones are the concrete factories
This is so abstract, it's extremely tough to understand
does this help?
we have 2 concrete factories, that make specific cars
we have the abstract factory that they both inherit from
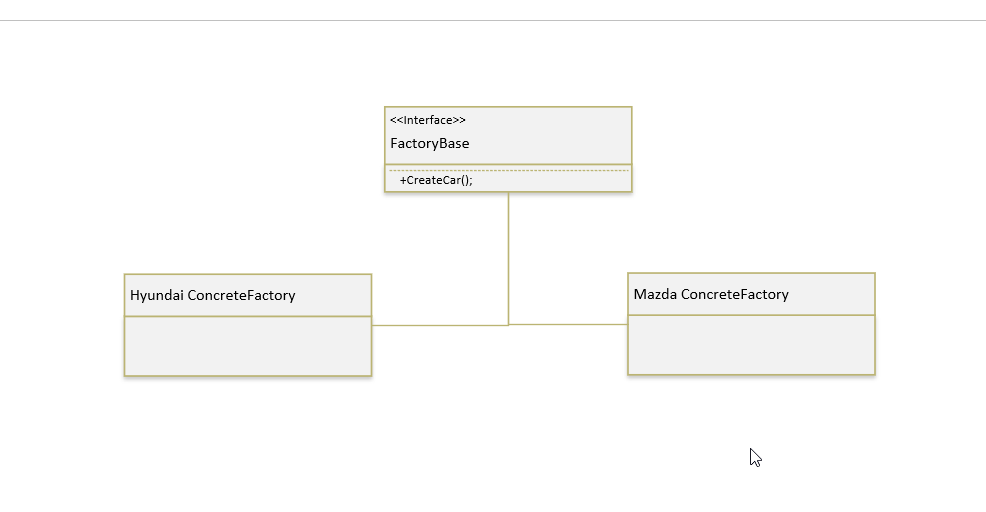
yeah thats exactly what we have here
And the stringname is the parameter which decides which car to make? Or rather which concrete Factory to enter?
yes to the first one, no to the second one
Just to go through it, making sure i get it right.
So we call the
Create
method with the argument string name
? How does it know which one concrete factory to enter?it doesnt
you cant have an instance of
FactoryBase
its impossibleRight, i'm onboard with that. I'm just curious to what happens when we call the method
Create
. Since Create
is defined in both factories.well, Create is an instance method
its called on the instance of the factory
and since we cant make instances of
FactoryBase
, its only called on a concrete factory
so we already know if its a mazda or a hyundai factoryI'm not sure i understand that part.
I was taught that when we call on a method such as car
Create()
it will call that specific method and apply everything inside the {}
. However Create()
is defined inside both factories. So how does C# know which factory to enter? Does it enter both of them? Or does it go to the appropriate one based on the argument inside create()
?Show me the code you are thinking about
alternatively, do you have a headset at hand and can jump into a VC? I could show you via screenshare exactly how this works.
This is what i'm basing it of.
When we call the method
create
from public abstract Car Create(string name);
. Both MazdaFactory
and HyundaiFactory
has the method Create
. So which one will it enter?neither
it cant
you cant do what you are saying
methods work differently inside abstract classes?
Essentially what i'm asking is:
What happens when
public abstract Car Create(string name);
is calledno, but in that code you just pasted, there is no method call
can you write out the method call
show me HOW you would invoke that method
public abstract Car Create(string name);
public abstract Car Create(string name);
this is a method?yes
but its an abstract method
that particular method will never be called.
Oh so we aren't actually invoking it inside the abstract class?
there are no invocations anywhere here
Shit
its just declarations
Okay that's why i'm confused lmao
invocations are the stuff you'd see in "main"
I assumed it was an invocation of it
So if i were to invoke it inside main
look, can you jump on a screenshare call right now? it'd be easier to show
Sure
#vc-3