β Factory Method Pattern
Does someone have a good explanation or perhaps a link to somewhere where i can learn this? Been trying to find a video or similar but it just doesn't stick :(
81 Replies
so you know how constructors work right?
I think soooo
var x = new Product("Apple", 15, 3.5);
etcWe store an instance of Product inside var x ?
sure, but the "constructor" here is the
new Product("Apple", 15, 3.5)
partSo the constructor is the one that instanciates the object?
yes
Oki, then yeah i'm with you :cathype:
a "factory" in OOP is a class/method that knows how to create something else. it knows the details needed to call the constructor properly, and maybe set some values on the object before returning it
in this case, maybe you dont know what the
15
and 3.5
should be
lets assume 15
is the "Product ID", and that should be an incremeneted number. It would be silly to expect the programmer to constantly keep track of the current ID and manually give itOki
so we can make a "ProductFactory" instead that keeps track of it for us
Would that be an
abstract class/interface
?uh... probably not
Weird, i think our teacher specifies it as such π
abstract things cant be created, so how would you create the factory?
Ah, are you refering specifically to the "abstract factory" pattern?
I think so? GoF ?
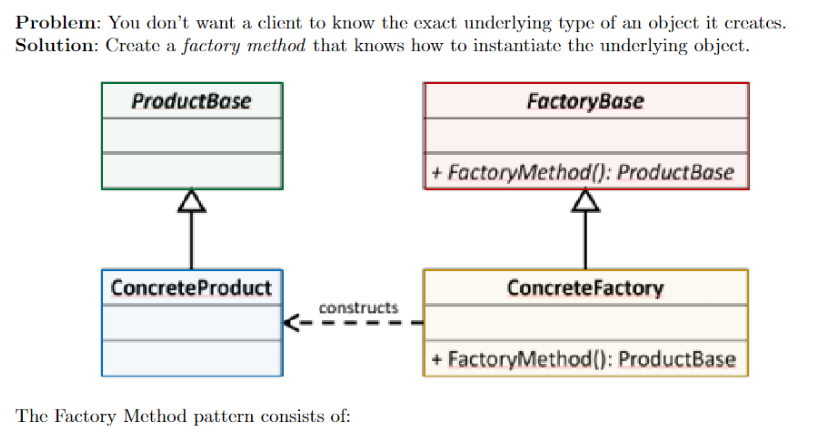
yeah
This is what she explains it as
yeah GoF has an "abstract factory" in it
FactoryBase
here is your abstract factory
check this out: https://refactoring.guru/design-patterns/abstract-factoryHow do you know that is abstract
their explanations are usually pretty good
well it has a
Base
suffix and the title is in italics
and there are other classes called ConcreteSomething
concrete is the opposite of abstract...Ooh
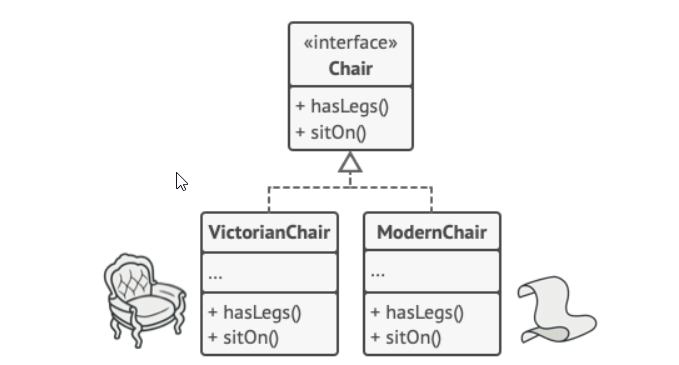
So in my case the interface here would be Carfactory?
?
this is the first time you mention
Car
Oh wait i might not have included everything
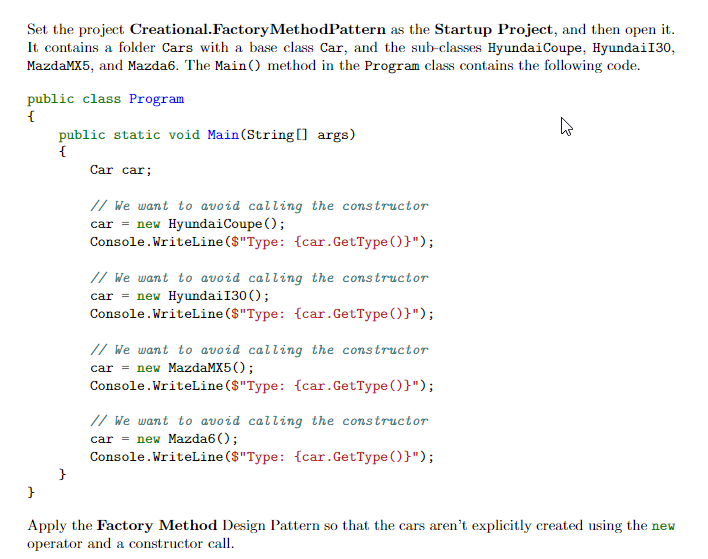
This is what i'm working on atm
And i'm trying to implement the Factory method (which i'm currently learning)
right
In your case
Car
would be an abstract base class, not an interface, but otherwise it would fulfill the same role as "Chair" does in the above imageI see
We were provided an abstract class for
Car
in the project
Hmm
iβll finish reading the page, one moment ^^I can't see the resemblance of this ^ and https://discord.com/channels/143867839282020352/1288142980603052105/1288148258987708447
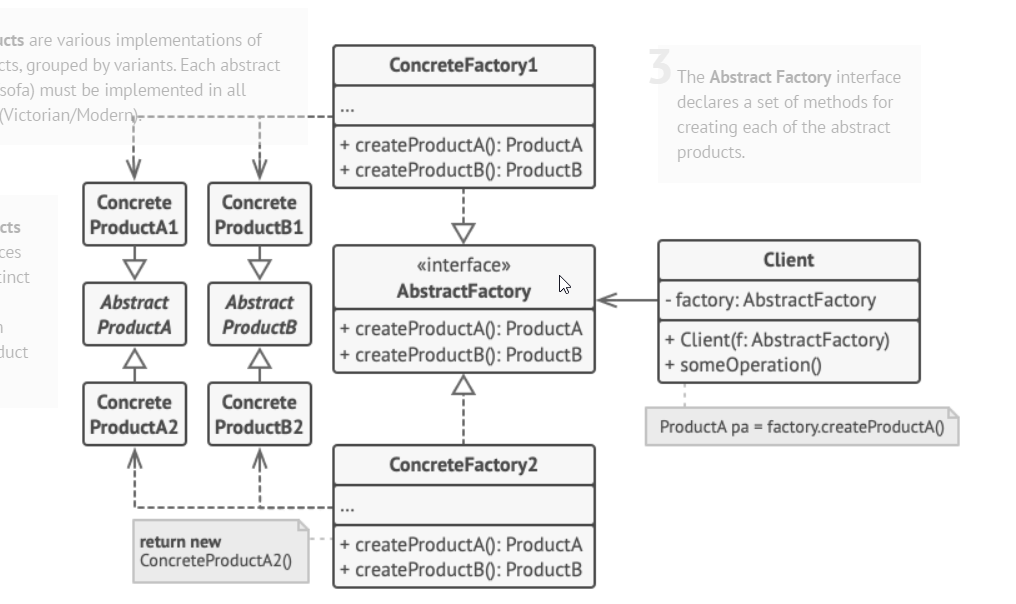
Merineth
Quoted by
<@577931037393420298> from #Factory Method Pattern (click here)

React with β to remove this embed.
Would the "Client" be my main method?
yeah
Client is missing in this?
Would FactoryBase be a subclass interface of the client?
you dont specifically need a client
FactoryBase is
AbstractFactory
they do the same thingI see
So this Interface would be called from main in order to make the car?
And then depending on what type of car we want different Concrete Factories are called?
π this is so confusing
I don't even see the benefit of using this Factory method
Not even gpt can explain it to me :I
Well in this case there is no benefit
Like i fail to see what's wrong with this
This is just a stupid example
:(
10/10 teacher
eh, more like bad... kursplaner
(: yup
its hard to understand why you'd need an abstract factory until you do
So they dont want me to instanciate cars with constructors in the main program
and its not a pattern you use for simple apps traditionally
They want me to use a factory to do it for me
So i instanciate them inside the factory instead
I mean, the simplest implementation of a factory literally just calls the constructor... which is pretty silly
Welp i've been trying to figure out what it even is for a few hours now
I'm surprised there aren't videos covering it under 20 min
the link I gave above is... pretty good.
:{
Yeah for someone who already knows it π
The first thing the Abstract Factory pattern suggests is to explicitly declare interfaces for each distinct product of the product family
So i create an interface for car?
or rather an abstract classWell, you already have that, no?
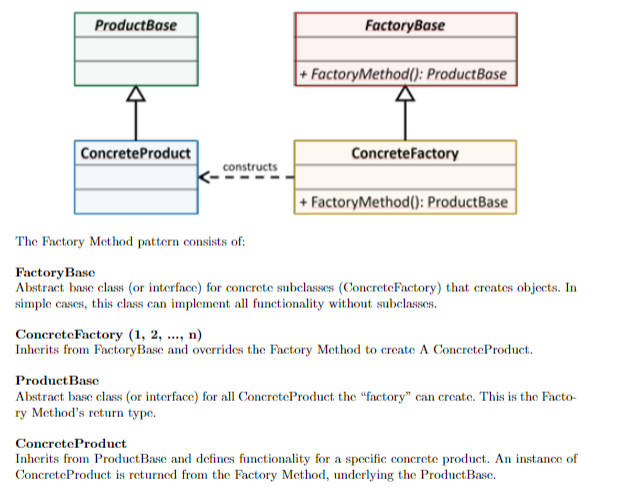
Would that be the FactoryBase?
Or do i need another abstract class for a total of two?
you would indeed have two
one for Car, one for AbstractCarFactory
or CarFactoryBase
whatever
then you make one concrete factory for each car variant
Confusing considering there aren't two here
you can make your factories in different ways
that one has its example of the three kinds of furniture
each factory knows how to make a chair, a sofa and a table
the difference there is the style of the factory. In your case, you only have one "dimension" of difference
So in the example from the site we'd need 3 interfaces for each chair, sofa and table?
yeah
a baseclass for each type
either interface or abstract class
Ok so why do iu need two interfaces when there is only one object, car
(i just assume interface and abstract class are the same)
because you have the BaseThing (car) and the FactoryBase
thats two things
but none are concrete
And what's the difference between those two iabstract classes?
what
one is a car
the other is a factory
... .d
hahahah
but i don't get it
I don't even understand what they are mean to do
in this case, NOTHING
I have 4 different types of cars,
Oh
they are literally just to tie the hierarchies together
I found the solution they provided
As you said the
Car
abstract class is completely empty
However the CarFactory
is not
well, it provides the base implementation for
CreateCar
yeah
however, no body
so its effectively an interfaceno body?
yeah no body
notice how the method has... no body
Oh, right
iirc abstract classes can't have a body to their methods
only the definitions
abstract classes can
abstract methods cant
abstract classes can have non-abstract methods
So what's the difference between this ^and that?
welllllll
this uses the factories
so uh.. yeah, as said
this example sucks π
this example very much just shows you what the code looks like, it doesnt show you why or when this pattern makes sense
haha i juat have a really hard time understanding or relating to stuff when iβm not given concrete examples :(
okay i took a quick nap D:
If anyone sees this and wouldn't mind explaining Factory Method to me :sadcat:
Anyone ? :/
This is the provided solution
However it doesn't really bring me any closer in understand what factories do.
i would use a factory for creating clients, for example
How would that work?
The only rough idea i have around factories are that they are using to encapsulate outside of the main method? As in the constructor isn't done in the main?
static factory method <T> where T : WhatEverClassThisIs, new() π
i would use for example as a helper to have some logic when instantiating a class
and then there could be other
CreateClient
methods with different parameters