null check raising errors
i come from python and my first interpretation was that they can work like decorators, but that's clearly not the case.
my question is how do i modify class data with an attribute?
like how in python you can decorate a class like this:
how would i do this in C#?
53 Replies
Attributes do nothing
They are just markers
They can be used by reflections or source generators, and those can provide additional functionality
Is there anything in particular you need to do, or is it just a theoretical question?
Or you can go offline, sure, that's also fine
oh sorry, i shut my laptop to go make breakfast. im back now
i want to set a docstring on a command class that says how the user explains the command to work
No need for attributes there
Just use documentation comments
Just type
///
before whatever you want to document, and your IDE will automatically generate a doc string, with all the parameters, return, and so oni was making my own CLI so code comments wouldnt work
You'll just have to fill it in
Ah, well, attributes in this case, sure
Probably used with reflections
Or with source generators
how would i do those then
what are source generators
Both are fairly advanced topics
Source generators more so than reflections
Source generators let you... generate source code. They allow you to write code that inspects your existing code and generates new code
As for how, Microsoft's docs are fairly good, at least on the topic of reflections, so I'd start there
ah ok will definitely look at it
by the way, do you know how to do this?
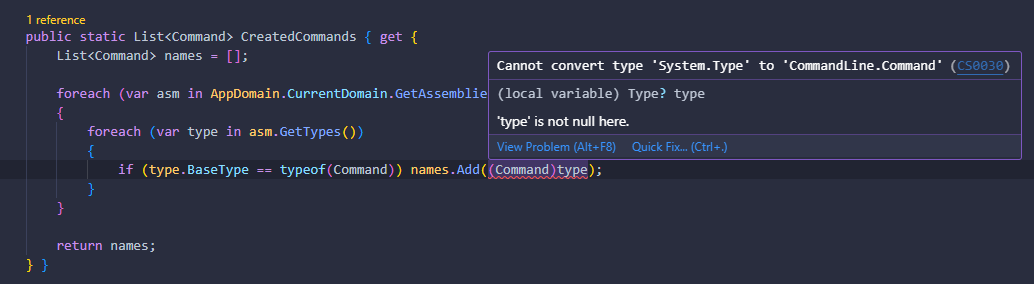
basically i have a property called
CalledCommands
that gets all the subclasses of the Command
class
but of course, it's just a generic Type
typeYou want an instance of
Command
?not an instance of
Command
, all the subclasses of Command
it works fine and gets the correct names, but i dont get how i convert from Type
to Command
Activator.CreateInstance()
method will be useful
It lets you create an instance from a Type
ohh
i dont have an empty constructor tho
It lets you pass parameters too
Just look up the docs
how does that help?
sorry, i really just dont get it
how does creating an instance work? do i not already have the instances?
oh wait
yep, still dont get it
If I may suggest some other, simpler project for learning purposes?
uh sure
That said, to do anything, you need an instance of a given class, an object
ah ok makes sense
A
Type
instance that stores type info of Command
is not an instance of Command
If you want to list all subclasses of Command
based on the Type
you'll need to use reflections to get them
Then, from what I gather from the code, you'll want to instantiate each of them, and store them in a list?yep
Ah, wait, scratch that, you are getting all subclasses of command already
So, yeah, only instantiation is left
the help command gathers all the created commands and returns the docstring the user applies to them
for some reason, this is the console output
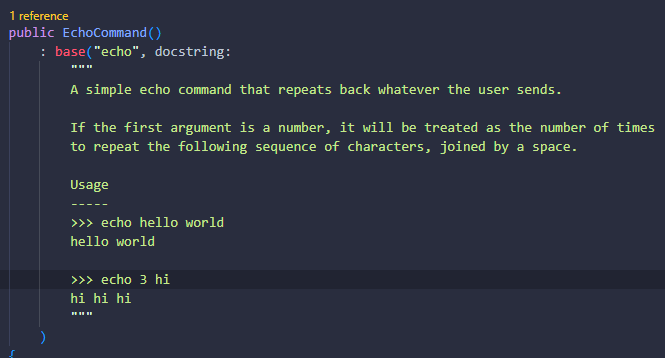
when printing it in
dotnetfiddle.net
, it works fineUh
No idea how would you ever get this output lmao
(sorry for light mode users)
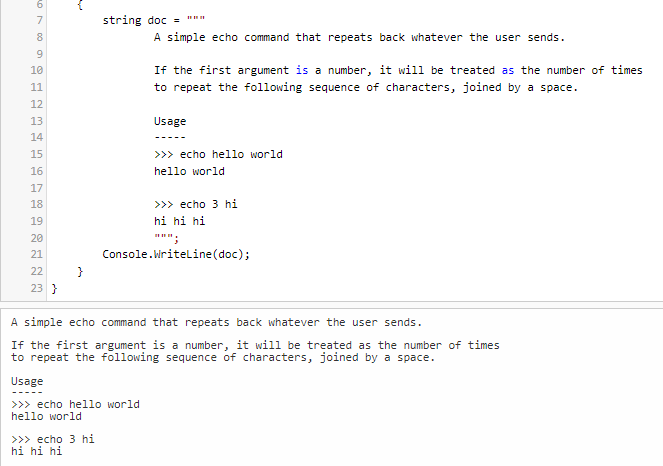
new bug found
Do you use any async code at all?
That's about the only thing that comes to mind
nope
fully sync
Something being printed, while something else moves the cursor position
I'm at a loss, then
I'd probably just opt for an existing CLI library lol
lemme check my
WriteLine
funcs
boringSee if any of your code sets cursor position
Comment those bits out and try again
Also, make sure everything actually is fully sync, and not some shenanigans like calling an async method in a synchronous context
Post the whole repo, maybe the error is somewhere else
Everything here looks just fine
I'll take a look tomorrow, gotta go to sleep now
https://mystb.in/5b803b98869adcf3ca - here's the full thing
I guess you could also try stepping through the code with the debugger, idk
found the error
i did
.Replace("\n", "").Replace("\n\n", "\n")
to turn two new lines into one and one new line into nothing
but i think i fucked something up thereregarding using attributes for command documentation, you can look at one of the many other CLI frameworks that already do this. https://github.com/Tyrrrz/CliFx comes to mind as my personal favorite
back from the gym
oh that looks pretty cool. i'll do my best to adopt a few concepts from there
i get this error even though (i think) i've correctly overloaded
==
. heres my code - https://mystb.in/2c965b9e95b80075f2 - and let me know what i did wrong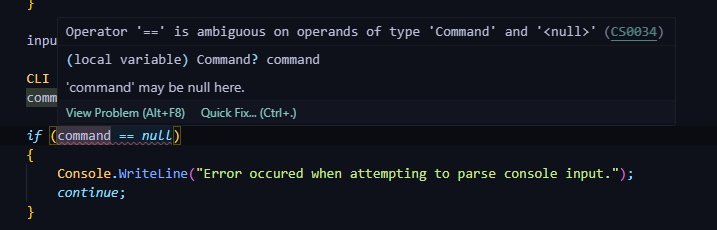
Can you show the definition of
Command
, is that defined by you?its in the pastebin
Yeah so you define an equality with string and command
null
could be eitherohh
Hence the ambuiguity error, doesn't know which == to invoke here
You can use
ReferenceEquals(command, null)
If you wanna circumvent this
(Even better if you remove the string versions of == IMO)well i want to be able to compare a string with an argument
so if the argument name is the same as the string or the string is the alias of an argument
wait
should i just add a cast?
What's wrong with typing
cmd.Name == ...
You can have null as Command
or null as string
yes
That will disambiguate it
But ReferenceEquals
works tooi meant explicitly comparing with a string, eg.
whats that
ReferenceEquals
literally checks, if the two references are the same. Similar to pointer equality in C or something, checks if the two things are literally the same instance, disregarding any other equality defined on the types.