✅ null reference
How do I fix possible null reference return do I add = null! after the errormessage or why does that come up as a warning?
```cs
using System;
using System.IO;
namespace Calculator.View
{
public class CalculatorView
{
public string ErrorMessage { get; set; }
public double ResultString { get; set; }
public string InputString { get; set; }
public string GetRPNString()
{
Console.WriteLine();
return Console.ReadLine();
}
public void DisplayResult(double result)
{
Console.WriteLine("Result: " + result);
}
public void DisplayError(string errorMessage)
{
Console.WriteLine("Error: " + errorMessage);
}
public string ReadInputFromFile(string filePath)
{
using (StreamReader reader = new StreamReader(filePath))
{
return reader.ReadLine();
}
}
public void WriteOutputToFile(string filePath, double result)
{
try
{
using (StreamWriter writer = new StreamWriter(filePath, false))
{
writer.WriteLine("Result: " + result);
}
Console.WriteLine($"Wrote result to file: {filePath}");
}
catch (Exception ex)
{
Console.WriteLine($"Error writing to file: {ex.Message}");
}
}
}
}
```
19 Replies
$code
To post C# code type the following:
```cs
// code here
```
Get an example by typing
$codegif
in chat
For longer snippets, use: https://paste.mod.gg/And please post the error message (including line numbers etc).
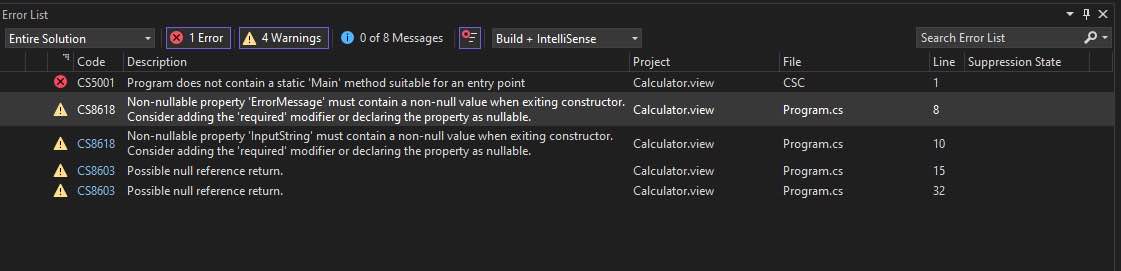
(and please format your code as C#, by editing your previous messages and surrounding your code with backticks, see the message above)
To be honest, if you're just starting out, I'd probably recommend turning off the nullable reference types warnings. Edit your .csproj, and change
<Nullable>enable</Nullable>
to <Nullable>disable</Nullable>
$codegifI am trying but it’s not working
Okay but I can’t have any warnings when I turn it in
One way would be to make your properties
required
The error happens because the properties might be uninitialized when you access themYes okay so do you mean by doing InputString = inputstring and the same with the other ones inside the calculatorview class?
Did you do this?
No I did not but I want to be able to see the warnings that my teacher will get as well
They will have to be set in the constructor specifically
Or set to default values
Or made
required
Huh? I'm assuming your teacher is going to be opening your solution? Or are they going to be copy/pasting code code into their own solution?
Warning:
Good:
Good:
Bad, but fixes the warning:
Good:
Good:
Good:
Good:
Ohh okay I see what you mean
using System;
using System.IO;
namespace Calculator.View
{
public class CalculatorView
{
public string ErrorMessage { get; set; }
public double ResultString { get; set; }
public string InputString { get; set; }
public CalculatorView(string errormessage, string inputstring)
{
ErrorMessage = errormessage;
InputMessage = inputmessage;
}
public string GetRPNString()
{
Console.WriteLine();
return Console.ReadLine();
}
public void DisplayResult(double result)
{
Console.WriteLine("Result: " + result);
}
public void DisplayError(string errorMessage)
{
Console.WriteLine("Error: " + errorMessage);
}
public string ReadInputFromFile(string filePath)
{
using (StreamReader reader = new StreamReader(filePath))
{
return reader.ReadLine();
}
}
public void WriteOutputToFile(string filePath, double result)
{
try
{
using (StreamWriter writer = new StreamWriter(filePath, false))
{
writer.WriteLine("Result: " + result);
}
Console.WriteLine($"Wrote result to file: {filePath}");
}
catch (Exception ex)
{
Console.WriteLine($"Error writing to file: {ex.Message}");
}
}
}
}
```
I added that now as it got rid of two warnings but there are still two warnings in GrtRPNString and ReadFromInput
It’s the return Console.ReadLine() that has a possible null reference return
You need the ```cs at the start of your code
Yep,
ReadLine()
returns string?
So a possible null
You'll have to handle that, for example with a null-coalescing operator
Hmm yes okay
Can I not just add a ? after public string?
Cuz that removes the warnings
It seems to work now I just need to make sure that I am writing to a file correctly
Actually I ran into another problem when writing to a file it only writes the latest value so do I need like a loop to fix that?
!close
Closed!