How can i avoid that a user can "signup" twice with the same email address?
At the moment, it's possible for users to sign up with the same email address and still get this confirmation message on the signup page:
You've signed up successfully! Check your email for the confirmation link.
In the database, nothing changes if the email address already exists but how can I check upfront as part of the sign up process if the email address already exists?
12 Replies
Hi @mb23. I'm kapa.ai, your friendly support bot. I will try to answer your question by searching through the knowledge base. :wave:
----------------------
In Wasp, you can customize the sign-up action to check if an email already exists in the database before proceeding with the sign-up process. This can be done by using the
findAuthIdentity
function which checks if an authentication identity already exists for a given provider ID.
Here is a sample code snippet from the Wasp documentation that shows how to do this:
In the above code, findAuthIdentity
is used to check if an authentication identity already exists for the provided email. If it does (existingAuthIdentity
is not null), you can return a custom message indicating that the email is already in use. If it doesn't, the code proceeds to create a new user.
Please note that this is just a basic example. You might need to adjust the code to fit your specific needs. For more information, you can refer to the Wasp documentation on creating a custom sign-up action (https://wasp-lang.dev/docs/auth/email#creating-a-custom-sign-up-action).
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------
Sorry @mb23, I seem to be having a bit of trouble. Don't worry though, I've pinged my support and they will try to fix me up! :technologist:
Perhaps try again in 15mins?We consider the user registered only after they confirm their email. Until then, the email is considered free. Imagine if somebody signed up with someone's email and now that person can no longer create an account.
To perform some extra logic before the signup (throw an error to stop the signup from happening if you want) - you can always use the
onBeforeSignup
hook: https://wasp-lang.dev/docs/auth/auth-hooks#executing-code-before-the-user-signs-upAuth Hooks | Wasp
Auth hooks allow you to "hook into" the auth process at various stages and run your custom code. For example, if you want to forbid certain emails from signing up, or if you wish to send a welcome email to the user after they sign up, auth hooks are the way to go.
What Miho said and also, this is kind of a security feature - you don't want to tell users that an account with an email exists to prevent enumeration attacks (i.e., finding out who uses certain apps)
@Filip @mb23 @kapa.ai How can we implement custom error-handling logic for signup in Wasp version 0.11.8?
Let me check the knowledge base for you again. One moment! :books:
----------------------
To implement custom error-handling logic for signup in Wasp version 0.11.8, you can create a custom sign-up action. This allows you to define your own error handling and return custom error messages. Here's how you can do it:
1. First, define a custom sign-up action in your
main.wasp
file:
2. Then, create a signup.js
(or signup.ts
if you're using TypeScript) file in your src/auth/
directory. In this file, you can implement your custom sign-up logic with error handling:
This approach allows you to catch any errors that occur during the sign-up process and return custom error messages. You can modify the error handling in the catch
block to suit your specific needs.
For more detailed information, you can refer to the Wasp documentation on creating a custom sign-up action (https://wasp-lang.dev/docs/auth/username-and-pass#2-creating-your-custom-sign-up-action).
Remember that this custom sign-up action will replace the default Wasp sign-up logic, so you'll need to implement all necessary checks and validations yourself.
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------
Sorry @john, I seem to be having a bit of trouble. Don't worry though, I've pinged my support and they will try to fix me up! :technologist:
Perhaps try again in 15mins?@kapa.ai I want the above implementation for version 0.11.8 not for 0.15.0
Let me check the knowledge base for you again. One moment! :books:
----------------------
I apologize for the confusion in my previous response. You're right to point out the version discrepancy. Let me provide you with the correct implementation for Wasp version 0.11.8.
For Wasp version 0.11.8, the implementation of custom error-handling logic for signup is slightly different. Here's how you can do it:
1. First, define a custom signup action in your
main.wasp
file:
2. Then, create a signup.js
(or signup.ts
if you're using TypeScript) file in your src/auth/
directory. Implement your custom signup logic with error handling:
This implementation is specific to Wasp version 0.11.8, as shown in the Wasp documentation for customizing the auth flow (https://wasp-lang.dev/docs/auth/username-and-pass#2-creating-your-custom-sign-up-action).
In this version, you define the custom signup action in the main.wasp
file and implement the logic in a separate JavaScript file. The error handling is done within the try-catch
block, allowing you to return custom error messages when exceptions occur during the signup process.
Remember that this custom signup action will replace the default Wasp signup logic, so you'll need to implement all necessary checks and validations yourself.
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------
Sorry @john, I seem to be having a bit of trouble. Don't worry though, I've pinged my support and they will try to fix me up! :technologist:
Perhaps try again in 15mins?@kapa.ai Cannot find module '@wasp/server/auth' or its corresponding type declarations.ts(2307)
getting these error in version 0.11.8
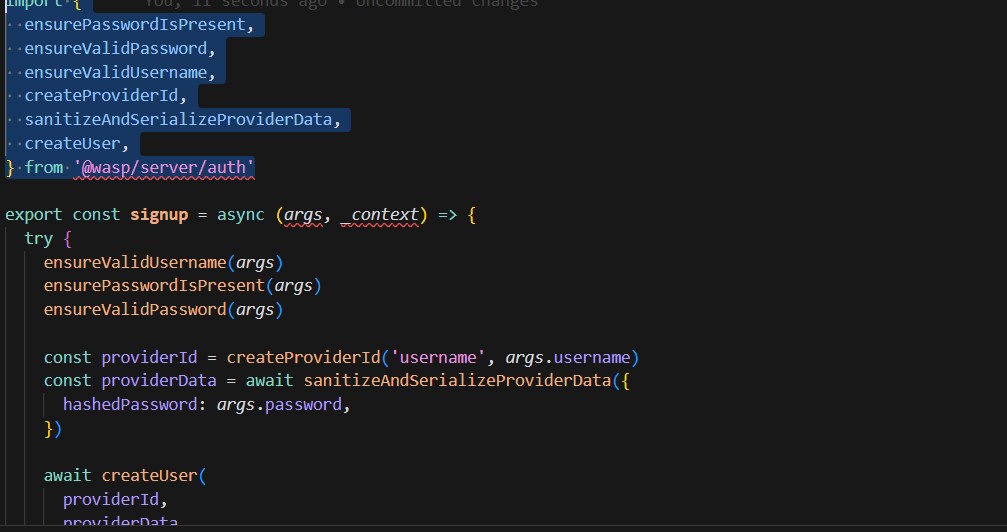
Let me check the knowledge base for you again. One moment! :books:
----------------------
This error typically occurs when the Wasp compiler hasn't generated the necessary files or when there's an issue with the project setup. For Wasp version 0.11.8, here are a few steps you can try to resolve this issue:
1. First, make sure you're importing from 'wasp/server/auth' instead of '@wasp/server/auth'. In version 0.11.8, the import should look like this:
2. If you're still getting the error, try cleaning and recompiling your Wasp project. Run the following commands in your terminal:
This will delete the generated files and rebuild them, which often resolves issues with missing modules Discord support thread (https://discord.com/channels/686873244791210014/1215750573278629958).
3. If the problem persists, check if you're running the Wasp compiler. The compiler needs to run to generate the SDK for you to have all the
wasp/*
packages Discord support thread (https://discord.com/channels/686873244791210014/1215750573278629958).
4. Make sure you're not modifying any files under the .wasp
directory. These are generated files and modifying them can cause problems Discord support thread (https://discord.com/channels/686873244791210014/1204832621176160286).
5. If you're using an IDE like VS Code, try restarting it. Sometimes the IDE's TypeScript server needs to be restarted to recognize the newly generated files.
If none of these steps resolve the issue, it might be worth checking if there's a problem with your Wasp installation or if there's a known issue with version 0.11.8. You could consider updating to a newer version of Wasp if possible, as newer versions might have resolved this issue.
Remember, always create your custom files within the src
directory, not in the .wasp
directory, as the latter is where Wasp outputs generated files Discord support thread (https://discord.com/channels/686873244791210014/1204832621176160286).
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------
Sorry @john, I seem to be having a bit of trouble. Don't worry though, I've pinged my support and they will try to fix me up! :technologist:
Perhaps try again in 15mins?@kapa.ai tried above steps but not working
Let me check the knowledge base for you again. One moment! :books:
----------------------
I apologize that the previous steps didn't resolve your issue. Since you're still experiencing problems with Wasp version 0.11.8, let's try a few more troubleshooting steps:
1. First, make sure you're using the correct import statement for version 0.11.8:
Note that in this version, it's
@wasp/auth
instead of wasp/server/auth
.
2. If you're still getting errors, try running wasp clean
followed by wasp start
again. Sometimes, multiple cleanups are necessary Discord support thread (https://discord.com/channels/686873244791210014/1213958217537814640).
3. Check if there are any issues with your project's .wasp
folder. As mentioned in a Discord support thread, sometimes problems can occur in this folder. You might try deleting the .wasp
folder and all node_modules
folders, then running wasp start
to reinstall everything Discord support thread (https://discord.com/channels/686873244791210014/1213958217537814640).
4. If you're using VS Code, try opening your project directly from Ubuntu (if you're using WSL) rather than through Windows. There have been cases where this has resolved similar issues Discord support thread (https://discord.com/channels/686873244791210014/1213958217537814640).
5. If none of these steps work, it might be worth considering upgrading to a newer version of Wasp, as many issues have been resolved in later versions.
If you're still encountering problems after trying these steps, it would be helpful to get more detailed information about the error messages you're seeing. With more specifics, we might be able to provide a more targeted solution.
Remember, if you continue to face difficulties, the Wasp community on Discord is often very helpful for troubleshooting specific issues Discord support thread (https://discord.com/channels/686873244791210014/1215750573278629958).
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------
Sorry @john, I seem to be having a bit of trouble. Don't worry though, I've pinged my support and they will try to fix me up! :technologist:
Perhaps try again in 15mins?@john I believe that this import is too new for your wasp version, and that is why it doesn't work. You should check the older verison of our docs, that is the same as your wasp version, or upgrade to newer version of the wasp.