Backend public?
Is there a way that I can make my api accessible from localhost while its on prod?
The current frontend and api are hosted already and working, but I want to also access it from localhost(for now) and other domains later on.
23 Replies
Hi @fustuk.. I'm kapa.ai, your friendly support bot. I will try to answer your question by searching through the knowledge base. :wave:
----------------------
Yes, you can make your API accessible from localhost and other domains by configuring Cross-Origin Resource Sharing (CORS) settings.
In the context of Wasp, there's an open discussion on GitHub about making CORS more restrictive. The idea is to pull origins from a
CORS_ORIGINS
environment variable. When running in development mode, localhost is also added. Users are advised to set CORS_ORIGINS
to the correct value on the hosting provider when deploying source (https://github.com/wasp-lang/wasp/issues/94).
There's also a suggestion to add a global property inside an App element to specify the domain and allowed origins. This would automatically allow localhost and the specified domain. For more advanced users, there's a suggestion to allow more domains simultaneously by specifying domains in an array or parsing an array from an environment variable source (https://github.com/wasp-lang/wasp/issues/94).
Please note that these are still open discussions and may not be implemented yet. You may want to follow the discussion or contribute to it to stay updated on the progress.
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------
Sorry @fustuk., I seem to be having a bit of trouble. Don't worry though, I've pinged my support and they will try to fix me up! :technologist:
Perhaps try again in 15mins?@kapa.ai
Let me check the knowledge base for you. One moment! :books:
Sorry @fustuk., I seem to be having a bit of trouble. Don't worry though, I've pinged my support and they will try to fix me up! :technologist:
Perhaps try again in 15mins?
@kapa.ai
Let me check the knowledge base for you. One moment! :books:
Sorry @fustuk., I seem to be having a bit of trouble. Don't worry though, I've pinged my support and they will try to fix me up! :technologist:
Perhaps try again in 15mins?
export const apiMiddleware: MiddlewareConfigFn = (middlewareConfig) => {
const customMiddleware: express.RequestHandler = (_req, _res, next) => {
next()
}
middlewareConfig.set('custom.middleware', customMiddleware)
return middlewareConfig
}
This is my middleware can I add it here somehow? @kapa.ai
Let me check the knowledge base for you. One moment! :books:
----------------------
Yes, you can add your custom middleware to the middleware configuration in Wasp. Here's how you can do it:
1. Global Middleware: If you want to apply your custom middleware to all operations and APIs, you can modify the global middleware. You can do this by defining a
middlewareConfigFn
in the server
field of your app
declaration. Here's an example:
In your myServerSetupCode.js
file, you can define your myMiddlewareConfigFn
like this:
This will apply your custom middleware to all operations and APIs source (https://wasp-lang.dev/docs/advanced/middleware-config#1-customize-global-middleware).
2. API-specific Middleware: If you want to apply your custom middleware to a specific API, you can do this by defining a middlewareConfigFn
in your api
declaration. Here's an example:
In your apis.js
file, you can define your myApiMiddlewareFn
like this:
This will apply your custom middleware to the specified API source (https://wasp-lang.dev/docs/advanced/middleware-config#2-customize-api-specific-middleware).
3. Per-Path Middleware: If you want to apply your custom middleware to all APIs under a common path, you can do this by defining a middlewareConfigFn
in your apiNamespace
declaration. Here's an example:
In your apis.js
file, you can define your myApiNamespaceMiddlewareFn
like this:
This will apply your custom middleware to all APIs under the specified path source (https://wasp-lang.dev/docs/advanced/middleware-config#3-customize-per-path-middleware).
Please replace myApp
, myServerSetup
, myMiddlewareConfigFn
, myApi
, myApiFn
, myApiMiddlewareFn
, myApiNamespace
, and myApiNamespaceMiddlewareFn
with the actual names in your project.
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------
Sorry @fustuk., I seem to be having a bit of trouble. Don't worry though, I've pinged my support and they will try to fix me up! :technologist:
Perhaps try again in 15mins?I found this, so I guess the github issues 2 years ago are not implemented.Is there any way I can make this accessible from local :? @Wasp Team
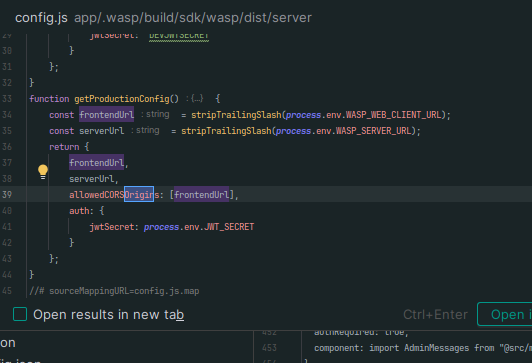
So the issue is that you can't hit your external api due to cors errors? If so, then you'd need to redeploy and set cors origins to all, i.e.
*
but where do I set it
in this function ? getProductionConfig
that would modify ALL operations and api endpoints though
if you want to only modify specific enddpoints, follow this: https://wasp-lang.dev/docs/advanced/middleware-config#2-customize-api-specific-middleware
Configuring Middleware | Wasp
Wasp comes with a minimal set of useful Express middleware in every application. While this is good for most users, we realize some may wish to add, modify, or remove some of these choices both globally, or on a per-api/path basis.
which one, would this work getProductionConfig or do I have to modify serverMiddlewareFn
Im fine with allowing all endpoints
I'm not away or the
getProductionConfig
function. What/where is that?hmm its in the build dir
app/.wasp/out/sdk/wasp/dist/server/config.js
I guess I cant edit that, nvm will use the approach you suggested thank you