How to render SVG file on HTMLString
class LeaveApprovalStatsOverview extends BaseWidget
{
....
private function createDescription(LeaveStatus $firstStatus, LeaveStatus $secondStatus): HtmlString
{
return new HtmlString(
view('components.leave-status-description', compact('firstStatus', 'secondStatus'))->render()
);
}
private function createLabel(int $first, int $second): HtmlString
{
return new HtmlString(
view('components.leave-count-label', compact('first', 'second'))->render()
);
}
}
class LeaveApprovalStatsOverview extends BaseWidget
{
....
private function createDescription(LeaveStatus $firstStatus, LeaveStatus $secondStatus): HtmlString
{
return new HtmlString(
view('components.leave-status-description', compact('firstStatus', 'secondStatus'))->render()
);
}
private function createLabel(int $first, int $second): HtmlString
{
return new HtmlString(
view('components.leave-count-label', compact('first', 'second'))->render()
);
}
}
<div class='flex'>
<div class='flex justify-center' style='color: {{ $firstStatus->getColorHex() }};'>
<span>
{{ $firstStatus->getLabel() }}
</span>
<span>
{{ $firstStatus->getIconSvg() }}
</span>
</div>
<span class='px-1'>/</span>
<div class='flex justify-center' style='color: {{ $secondStatus->getColorHex() }};'>
<span>
{{ $secondStatus->getLabel() }}
</span>
<span>
{{ $secondStatus->getIconSvg() }}
</span>
</div>
</div>
<div class='flex'>
<div class='flex justify-center' style='color: {{ $firstStatus->getColorHex() }};'>
<span>
{{ $firstStatus->getLabel() }}
</span>
<span>
{{ $firstStatus->getIconSvg() }}
</span>
</div>
<span class='px-1'>/</span>
<div class='flex justify-center' style='color: {{ $secondStatus->getColorHex() }};'>
<span>
{{ $secondStatus->getLabel() }}
</span>
<span>
{{ $secondStatus->getIconSvg() }}
</span>
</div>
</div>
<div class='flex space-x-2'>
<span>{{ $first }}</span>
<span class='px-1'>/</span>
<span>{{ $second }}</span>
</div>
<div class='flex space-x-2'>
<span>{{ $first }}</span>
<span class='px-1'>/</span>
<span>{{ $second }}</span>
</div>
4 Replies
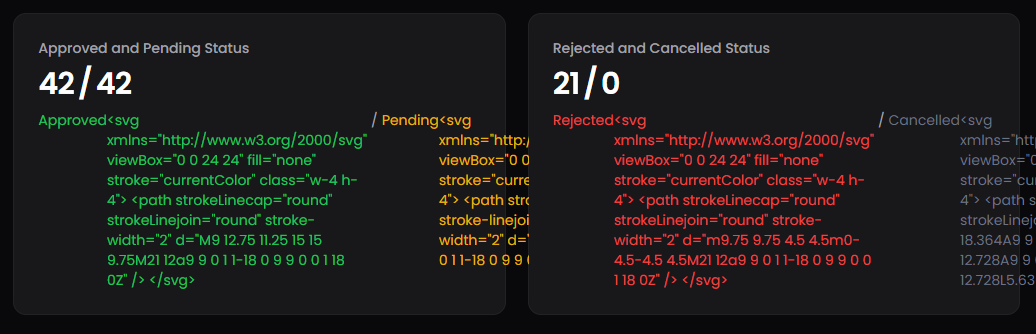
<?php
namespace App\Enums\HR;
use App\Concerns\EnumOptions;
use Filament\Support\Colors\Color;
use Filament\Support\Contracts\HasColor;
use Filament\Support\Contracts\HasIcon;
use Filament\Support\Contracts\HasLabel;
enum LeaveStatus: string implements HasColor, HasIcon, HasLabel
{
use EnumOptions;
case PENDING = 'pending';
case APPROVED = 'approved';
case REJECTED = 'rejected';
case CANCELLED = 'cancelled';
public function getLabel(): ?string
{
return ...;
}
public function getColor(): string|array|null
{
return match ($this) ...;
}
public function getColorHex(): ?string
{
return match ($this) ...;
}
public function getIcon(): ?string
{
return match ($this) ...;
}
public function getIconSvg(): ?string
{
return match ($this) {
self::PENDING => '<svg xmlns="http://www.w3.org/2000/svg" viewBox="0 0 24 24" fill="none" stroke="currentColor" class="w-4 h-4">
<path stroke-linecap="round" stroke-linejoin="round" stroke-width="2" d="M12 6v6h4.5m4.5 0a9 9 0 1 1-18 0 9 9 0 0 1 18 0Z" />
</svg>',
self::APPROVED => '<svg xmlns="http://www.w3.org/2000/svg" viewBox="0 0 24 24" fill="none" stroke="currentColor" class="w-4 h-4">
<path strokeLinecap="round" strokeLinejoin="round" stroke-width="2" d="M9 12.75 11.25 15 15 9.75M21 12a9 9 0 1 1-18 0 9 9 0 0 1 18 0Z" />
</svg>',
self::REJECTED => '<svg xmlns="http://www.w3.org/2000/svg" viewBox="0 0 24 24" fill="none" stroke="currentColor" class="w-4 h-4">
<path strokeLinecap="round" strokeLinejoin="round" stroke-width="2" d="m9.75 9.75 4.5 4.5m0-4.5-4.5 4.5M21 12a9 9 0 1 1-18 0 9 9 0 0 1 18 0Z" />
</svg>',
...
};
}
}
<?php
namespace App\Enums\HR;
use App\Concerns\EnumOptions;
use Filament\Support\Colors\Color;
use Filament\Support\Contracts\HasColor;
use Filament\Support\Contracts\HasIcon;
use Filament\Support\Contracts\HasLabel;
enum LeaveStatus: string implements HasColor, HasIcon, HasLabel
{
use EnumOptions;
case PENDING = 'pending';
case APPROVED = 'approved';
case REJECTED = 'rejected';
case CANCELLED = 'cancelled';
public function getLabel(): ?string
{
return ...;
}
public function getColor(): string|array|null
{
return match ($this) ...;
}
public function getColorHex(): ?string
{
return match ($this) ...;
}
public function getIcon(): ?string
{
return match ($this) ...;
}
public function getIconSvg(): ?string
{
return match ($this) {
self::PENDING => '<svg xmlns="http://www.w3.org/2000/svg" viewBox="0 0 24 24" fill="none" stroke="currentColor" class="w-4 h-4">
<path stroke-linecap="round" stroke-linejoin="round" stroke-width="2" d="M12 6v6h4.5m4.5 0a9 9 0 1 1-18 0 9 9 0 0 1 18 0Z" />
</svg>',
self::APPROVED => '<svg xmlns="http://www.w3.org/2000/svg" viewBox="0 0 24 24" fill="none" stroke="currentColor" class="w-4 h-4">
<path strokeLinecap="round" strokeLinejoin="round" stroke-width="2" d="M9 12.75 11.25 15 15 9.75M21 12a9 9 0 1 1-18 0 9 9 0 0 1 18 0Z" />
</svg>',
self::REJECTED => '<svg xmlns="http://www.w3.org/2000/svg" viewBox="0 0 24 24" fill="none" stroke="currentColor" class="w-4 h-4">
<path strokeLinecap="round" strokeLinejoin="round" stroke-width="2" d="m9.75 9.75 4.5 4.5m0-4.5-4.5 4.5M21 12a9 9 0 1 1-18 0 9 9 0 0 1 18 0Z" />
</svg>',
...
};
}
}
Solution
{!! $firstStatus->getIconSvg() !!}
?I realize that was the mistake after sending this post. my bad and thank you, you good sire.