β Exceptions
Are exceptions essentially if statements that are global across the entire program?
14 Replies
Such as if Iβm dividing by 0 somewhere, I can make an exception that handles that specific problem across my entire program?
uh
Not as such, no
an exception is an object that represents an error of some sort, and they "bubble up" until caught
if you never catch it, it will terminate your program with an error
are you familar with whats called "the call stack"?
Exceptions are ββtryββ and ββcatchββ right?
no those are keywords that relate to exceptions
exceptions are C# objects that inherit from the
Exception
classOk so an exception class is a class thatβs meant to catch possible problems?
no, it represents an instance of the problem
hang on, let me write some demo code
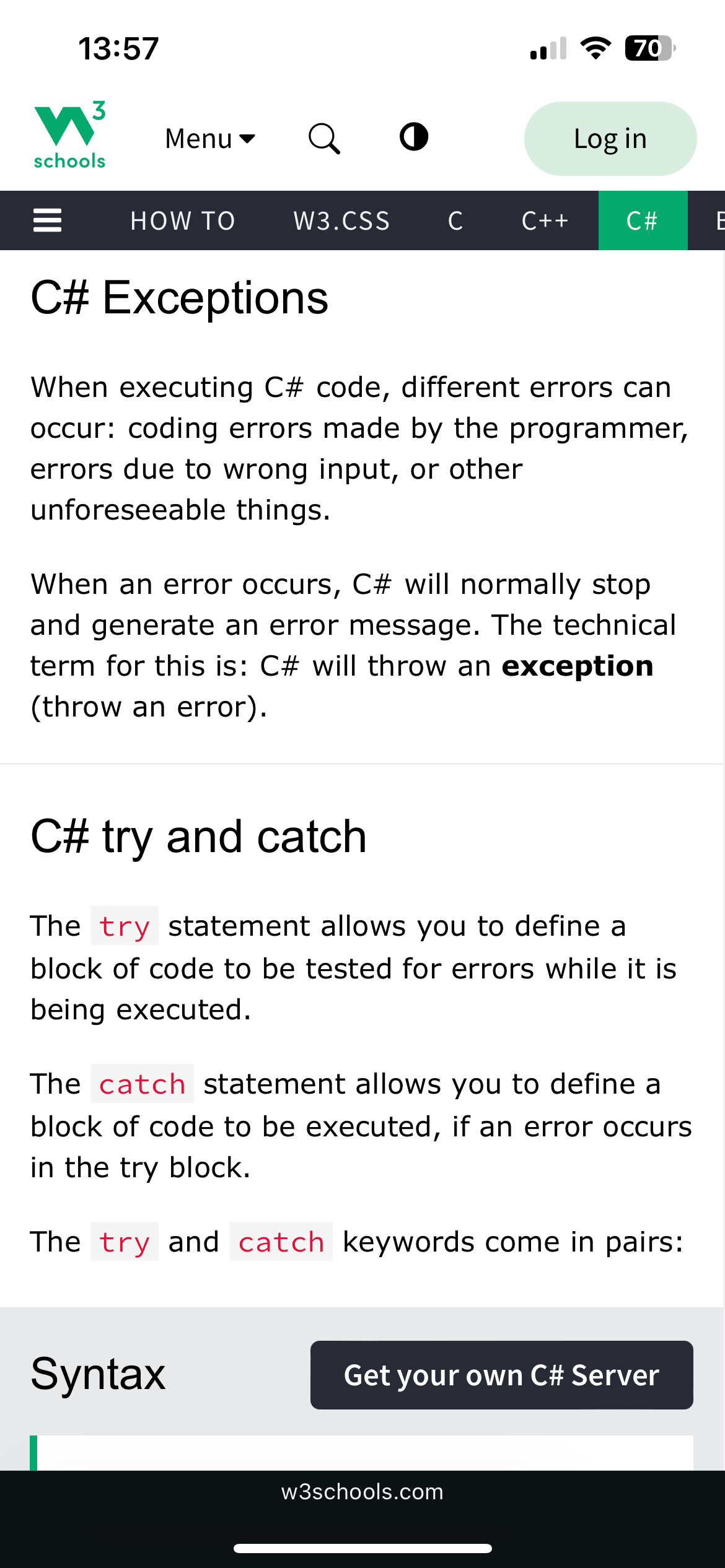
So instead of crashing the entire program it will give an exception instead with try and catch?
So we have to know beforehand what kind of error will occur to catch it?
I mean you can use
Which catches all exceptions, but generally not recommended. If you can try to avoid methods that can throw exceptions all-together
This does make senses tho
Ok so itβs generally better to know what problem could occur to catch that specific problem?
What would code look like if I want to catch multiple errors that could occur?
Like this?
like this
its pretty rare that you catch two or more exceptions with the exact same fix
and if you do, you can use...
but this is very rare, as said
Ok thanks I think I understand it now :)