How to change styles for login/signup page
I have looked through about every file and mass searched "yellow" and I cannot find the hex or any styling to change the login page. If anyone could point me in the right direction that would be great! Thank you!
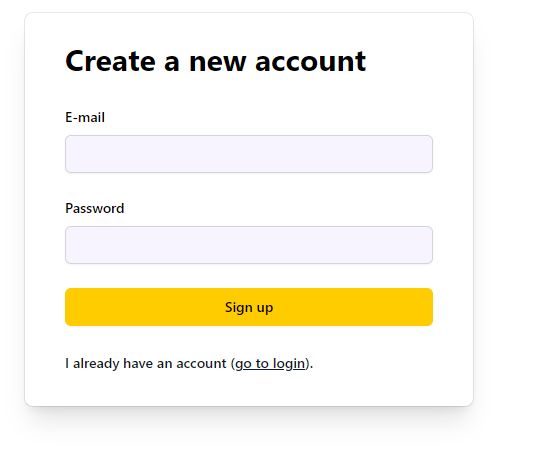
14 Replies
Hi @Sherm. I'm kapa.ai, your friendly support bot. I will try to answer your question by searching through the knowledge base. :wave:
----------------------
To customize the styles for the login/signup page in Wasp, you can pass props to the form components. Here are the steps:
1. Customize the form colors: You can customize the form colors by passing an
appearance
prop to the form. This prop should be an object that specifies the colors for different parts of the form. Here's an example:
Then, in your LoginPage component, pass the appearance
object to the form:
2. Customize the logo: You can also customize the logo by passing a logo
prop to the form. This prop should be the path to your logo. Here's an example:
3. Customize the layout of the social buttons: You can change the layout of the social buttons by passing the socialLayout
prop to any of the components. It can be either vertical
or horizontal
(default). Here's an example:
For more details, you can refer to the Wasp documentation on Auth UI Customization (https://wasp-lang.dev/docs/auth/ui#customization-).
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------
Sorry @Sherm, I seem to be having a bit of trouble. Don't worry though, I've pinged my support and they will try to fix me up! :technologist:
Perhaps try again in 15mins?which file would the appearance be in @kapa.ai
Let me check the knowledge base for you. One moment! :books:
----------------------
The
appearance
object can be defined in a separate JavaScript or TypeScript file. In the examples provided in the Wasp documentation, it is defined in a file named appearance.js
or appearance.ts
.
Here's an example of how you might define it in appearance.js
:
And here's how you might import it in your LoginPage.jsx
or LoginPage.tsx
:
You can place the appearance.js
or appearance.ts
file in the same directory as your LoginPage.jsx
or LoginPage.tsx
file for simplicity. However, you can also place it in a different directory if you prefer, as long as you adjust the import statement in LoginPage.jsx
or LoginPage.tsx
accordingly.
For more details, you can refer to the Wasp documentation on Auth UI Customization (https://wasp-lang.dev/docs/auth/ui#customization-).
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------
Sorry @Sherm, I seem to be having a bit of trouble. Don't worry though, I've pinged my support and they will try to fix me up! :technologist:
Perhaps try again in 15mins?@kapa.ai
import { Link } from 'react-router-dom';
import { LoginForm } from 'wasp/client/auth';
import { AuthPageLayout } from './AuthPageLayout';
export default function Login() {
return (
<AuthPageLayout>
<LoginForm />
<br />
<span className='text-sm font-medium text-gray-900 dark:text-gray-900'>
Don't have an account yet?{' '}
<Link to='/signup' className='underline'>
go to signup
</Link>
.
</span>
<br />
<span className='text-sm font-medium text-gray-900'>
Forgot your password?{' '}
<Link to='/request-password-reset' className='underline'>
reset it
</Link>
.
</span>
</AuthPageLayout>
);
}
here is my current LoginPage.tsx, how do I pass the appearance through?
Let me check the knowledge base for you. One moment! :books:
----------------------
To pass the
appearance
object to the LoginForm
in your LoginPage.tsx
, you first need to import the appearance
object. Then, you can pass it as a prop to the LoginForm
component. Here's how you can do it:
In this code, appearance
is an object that you define to customize the colors of your form. You would define it in the appearance.js
or appearance.ts
file like this:
For more details, you can refer to the Wasp documentation on Auth UI Customization (https://wasp-lang.dev/docs/auth/ui#customization-).
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------
Sorry @Sherm, I seem to be having a bit of trouble. Don't worry though, I've pinged my support and they will try to fix me up! :technologist:
Perhaps try again in 15mins?@kapa.ai
here is my css for my app
could you make a login page for me that looks nice using these same colors? As well add a logo area for me to add a logo
Let me check the knowledge base for you. One moment! :books:
----------------------
I'm sorry, but as an AI, I can't create a new design for you. However, I can guide you on how to customize your login page using Wasp's Auth UI and your existing CSS.
First, you need to define your
appearance
object with your desired colors. Here's an example:
Then, in your LoginPage.tsx
, you can pass the appearance
object to the LoginForm
component:
This will apply your custom colors to the login form. For the logo, you just need to pass the logo
prop to the LoginForm
component. The logo
prop should be the path to your logo.
Please note that the actual CSS styling (like layout, spacing, typography, etc.) would need to be done separately, and Wasp's Auth UI might not cover all aspects of it. You might need to write additional CSS or use a CSS framework to achieve your desired look.
For more details, you can refer to the Wasp documentation on Auth UI Customization (https://wasp-lang.dev/docs/auth/ui#customization-).
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------
Sorry @Sherm, I seem to be having a bit of trouble. Don't worry though, I've pinged my support and they will try to fix me up! :technologist:
Perhaps try again in 15mins?src/client/static/logo.png
here is my relative path for logo but its still saying it cant find it
@kapa.ai
Let me check the knowledge base for you. One moment! :books:
Wohooo @Sherm, you just became a Waspeteer level 1!
----------------------
If you're having trouble importing your logo, it might be due to the way static assets are handled in Wasp. When you import a static asset like an image, it should return its URL. Here's an example:
In this case,
logo
will be /logo.png
during development, and become /assets/logo.2d8efhg.png
in the production build. This ensures that the asset file exists and is included in the bundle.
If you're still having trouble, make sure the path to your logo is correct. The path should be relative to the file where you're importing the logo. For example, if your LoginPage.tsx
and logo.png
are both in the src/client
directory, you would import the logo like this:
If this doesn't solve your problem, it might be a good idea to check if there are any open issues related to this on the Wasp GitHub page (https://github.com/wasp-lang/wasp/issues).
For more details, you can refer to the Wasp documentation on static asset handling (https://wasp-lang.dev/docs/project/static-assets#importing-an-asset-as-url).
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------
Sorry @Sherm, I seem to be having a bit of trouble. Don't worry though, I've pinged my support and they will try to fix me up! :technologist:
Perhaps try again in 15mins?export const appearance = {
colors: {
brand: '#007bff', // blue
brandAccent: '#de5998', // pink
submitButtonText: 'white',
},
} can you make the background of the login page dark mode colors? @kapa.ai
colors: {
brand: '#007bff', // blue
brandAccent: '#de5998', // pink
submitButtonText: 'white',
},
} can you make the background of the login page dark mode colors? @kapa.ai
Let me check the knowledge base for you. One moment! :books:
----------------------
To change the background color of the login page, you would typically do this in the CSS or styling of the parent component that contains the
LoginForm
. However, the appearance
prop in Wasp's Auth UI currently doesn't support changing the background color directly.
Here's an example of how you might do it:
In this example, replace #yourDarkModeColor
with the hex code of your desired dark mode background color.
Please note that this will only change the background color of the div
containing the LoginForm
. If you want to change the background color of the entire page, you might need to do this in a higher-level component or in your global CSS.
For more details, you can refer to the Wasp documentation on Auth UI Customization (https://wasp-lang.dev/docs/auth/ui#customization-).
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------
Sorry @Sherm, I seem to be having a bit of trouble. Don't worry though, I've pinged my support and they will try to fix me up! :technologist:
Perhaps try again in 15mins?Hey @Sherm, did you see the docs here: https://github.com/wasp-lang/wasp/issues/1980#issue-2255149259
They show how to customise the login page and should cover your questions
GitHub
Fix error during
wasp start
after wasp start
and failing `wasp ...Version: 0.13.2. (probably post-0.12.0 and caused by #1769 (comment), doesn't happen with 0.11.8). OS: Linux How to reproduce: Run wasp new something && cd something. Run wasp start and...