Issues with Email Verification Redirect in Filament/Laravel Setup
Hi everyone,
I'm facing an issue in my Filament + Laravel project related to email verification. After verifying an email, the user is redirected to the login page instead of the intended success page. I've tried several approaches, including Laravel's default verification, a custom VerificationController, and Filament's built-in methods, but nothing has worked.
Key Details:
Custom Controller: Handles verification but fails to redirect correctly.
Filament Setup: Tried reverting to Filament's ->emailVerification() method, no change.
Simplified Routes: Used basic Laravel routes, issue persists.
Problem:
Users are redirected to the login page instead of the success page (/verification-success) after verifying their email.
Code Fragments:
VerificationController:
Email Verification Class:
Has anyone experienced something similar? Any suggestions would be greatly appreciated!
Thanks!
25 Replies
Remember that I use the super duper filament kit template.
Filament supports email verification. You need to add this in your panel
$panel->emailVerification()
and implement MustVerifyEmail contract
https://laravel.com/docs/11.x/verification#model-preparationLaravel - The PHP Framework For Web Artisans
Laravel is a PHP web application framework with expressive, elegant syntax. We’ve already laid the foundation — freeing you to create without sweating the small things.
thank you very much for replying but yes I have tried that too, :
])
->path('admin')
->login(Login::class)
->passwordReset(RequestPasswordReset::class)
->emailVerification(EmailVerification::class)
and class User extends Authenticatable implements FilamentUser, MustVerifyEmail, HasAvatar, HasName, HasMedia
same issue?
php artisan about --only=Filament
What is the Filament version?
Could you try this in a Filament fresh install?
I tried to install filament super duper kit in a clean way without any configuration but I didn't get it either, I tried in the verificationController class to define where it will be redirected to but neither, in spite of all my attempts I don't know which part forces to send to the login of the panel:
but I didn't get it either
what is the issue?
ok as I said above whatever changes you make or I can think of a user after pressing the email verification is directed directly to the login and I was hoping to put after the user presses the verification button some blade.
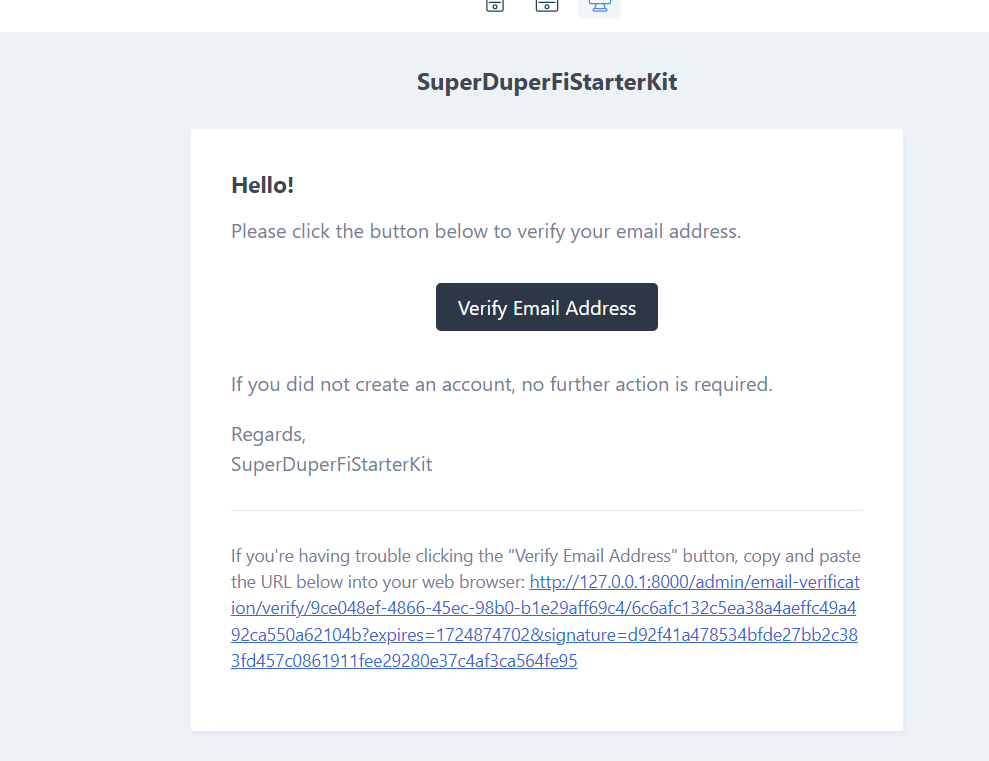
I have found some posts that talk about this in older versions of laravel, but it hasn't worked for me I don't know if my template I use super duperkit in some plugin or some file defines this I have searched but I haven't had much luck with the things I have modified.
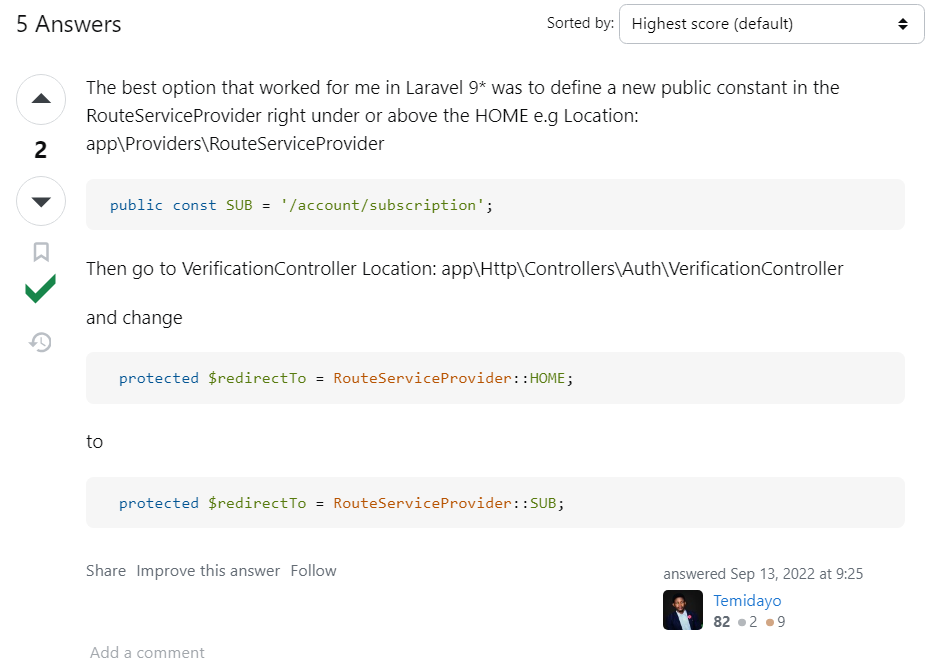
if you want , you can create a minimal repo on github to reproduce this issue, I can have a look after
of course I want it hehe I'm intrigued to know what my error or what happened to the redirection after verification I will upload immediately the minimum version you recommended to isolate the problem.
GitHub
GitHub - StikAlexander/route-pos-verification-email-try-change: ple...
please help me to find out who is in charge of redirecting the newly verified user to the login. - StikAlexander/route-pos-verification-email-try-change
🙏🏻
thanks
It looks like you’ve made some customizations to your project. I’ve enabled the registration form and noticed a few bugs. These issues don’t appear to be related to Filament itself but rather to the custom changes you’ve implemented.
If you’d like some help, please provide a minimal repository that replicates this specific issue
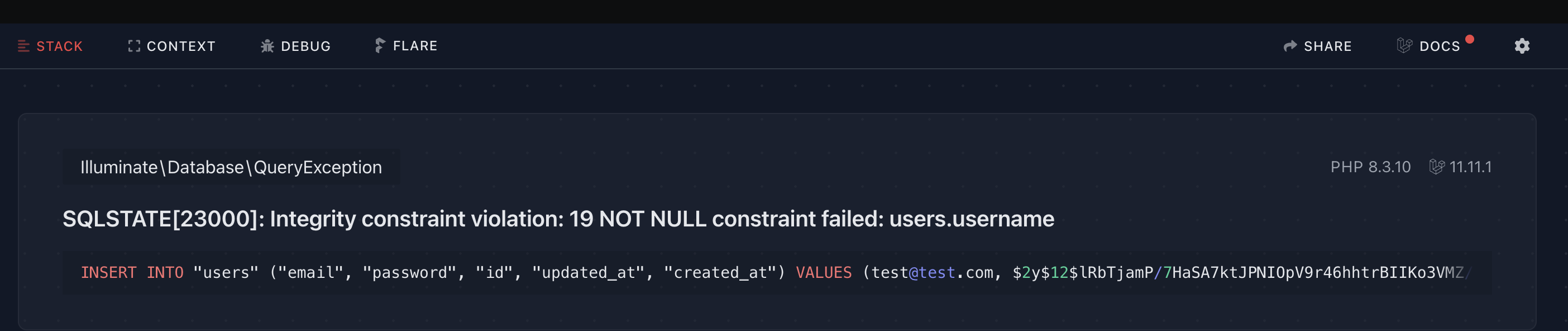
The changes I made to the repository I gave you were very minimal. Look, let's do the following: if you're okay with it, I'll send you the link to the Super Duper Starter Kit directly. If it's possible to implement the function of redirecting the user after verification, I'm sure that if I see an example, I'll learn how to edit it in my more advanced project in the future. Does that sound good, or does it go against the chat rules? Let me know, thanks a lot.
🫶🏻
Filament
SuperDuper Starter Kit by Rio Dewanto P - Filament
A start point to kickstart your next app. Features a Custom Theme, Mail Configuration, Shield, Exception, and more!
Shouldn’t the redirect just be handled in a middleware?
That’s the only guaranteed place to know anything about the authenticated user.
hello awcode the truth I have tried so many things and tips and none of them work for me haha it is very simple I am looking for the user after pressing the verification email to be taken to a blade where it says verification successful login, I feel that the default verification is functional but crude ie you press verify and it sends you immediately to the login
😅
`<?php
namespace App\Http\Responses\Auth;
use Filament\Http\Responses\Auth\EmailVerificationResponse as BaseEmailVerificationResponse;
use Illuminate\Http\RedirectResponse;
use Livewire\Features\SupportRedirects\Redirector;
class CustomEmailVerificationResponse extends BaseEmailVerificationResponse
{
public function toResponse($request): RedirectResponse | Redirector
{
return redirect()->route('custom.verification.success');
` the last thing they recommended me was to try with the EmailVerificationResponse file, but as it always gives the same result nothing happens the user is sent to loginThen something else is happening in your app. The verification should redirect to a login with a message that an email has been sent. So, yes they can’t log in at that point but the login screen is less important than the message saying they need to check their email. And when they click the link in the email, they get verified and can then login or be logging automatically by the verification token in the link.
Umm, as far as I know, it works fine. Let me quickly explain the flow: a user is created, they receive the verification email, click on verify, and are sent to the login page. They log in, and that's it. At verification, it stops being null. If this behavior is wrong, please let me know, as this is how it comes by default in the Super Duper Starter Kit template 😦
Ok, but I don’t know what the super duper starter kit is. If you are having issues it sounds like an issue with that.
It's a plugin or template maybe ?, and I understand. I don't want to waste much of your time. If it's a plugin error, it's best to migrate my project away from that template. I'll try that eventually, thanks. 🙏🏻
Reach out to the plugin author.
yes i already tried, sorry i will close the post thanks for the advice
You don’t have to close it. Some one might have an answer. But it’s hard to help in the general help thread when any plugin is involved.