hot swapping auth
Background: I've got a wasp app running username and password
-NOTE: the app has actions and queries that refer to the username (provideUserId) in order to display it in the ui
Wasp version: 0.14.0
Platform: MacOS Sonoma 14.1.1
Problem:
-I want to change the auth so that it's email + password BUT also includes a username field on signup so we can keep using this in the app
What's the best way to do this?
Possible Solution:
Should I change the main.wasp to email+pass then add username to user model in prisma? I've heard if you do custom auth correctly, wasp will push out custom fields to your auth forms
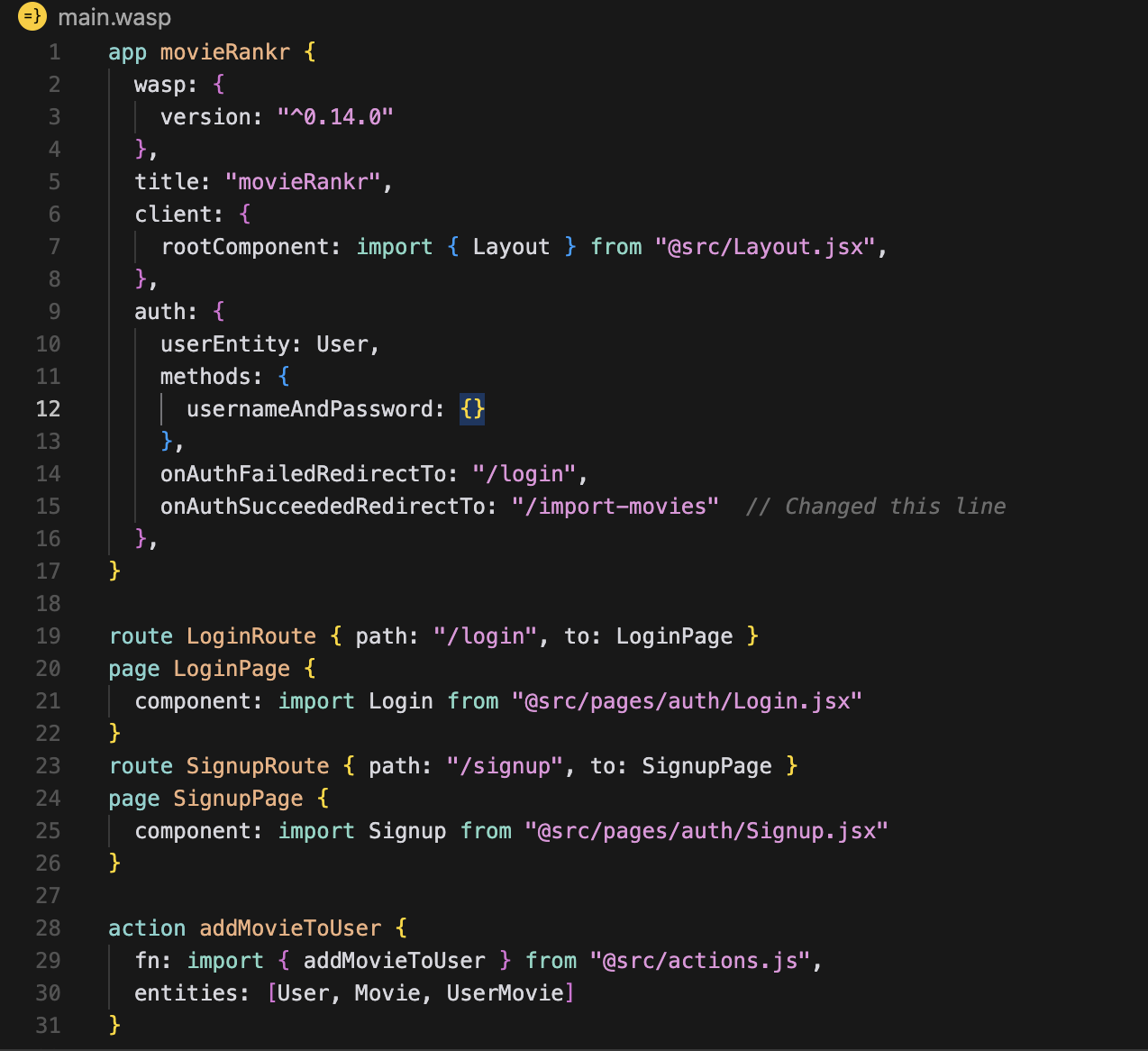
7 Replies
Hi @Billy Howell. I'm kapa.ai, your friendly support bot. I will try to answer your question by searching through the knowledge base. :wave:
----------------------
Yes, you're on the right track. Here's a step-by-step guide on how to achieve this:
1. Change the auth method to email and password in your
main.wasp
file. You can do this by modifying the auth
field in your app
declaration. Here's an example:
(Source: Wasp Docs - Setting Up Username & Password Authentication (https://wasp-lang.dev/docs/auth/username-and-pass))
2. Add a username
field to your User
entity in your Prisma schema. This will allow you to store the username for each user.
3. Define extra fields for the signup process. You can do this by defining a userSignupFields
object in your src/auth/signup.js
file. Here's an example:
(Source: Wasp Docs - Defining Extra Fields (https://wasp-lang.dev/docs/auth/overview#1-defining-extra-fields))
4. Update your main.wasp
file to use the userSignupFields
. You can do this by modifying the auth
field in your app
declaration. Here's an example:
(Source: Wasp Docs - Defining Extra Fields (https://wasp-lang.dev/docs/auth/overview#1-defining-extra-fields))
5. Update your actions and queries to use the email
field instead of the username
field. Since you're switching to email and password authentication, you'll need to update any actions or queries that refer to the username
field to use the email
field instead.
6. Test your changes. Make sure to thoroughly test your changes to ensure that everything is working as expected.
Remember, Wasp will automatically include any extra fields you define in the signup form. So, if you define a username
field as an extra field, Wasp will include it in the signup form.
Also, keep in mind that Wasp recommends using email and password authentication for production use, as username and password authentication is very basic (source (https://discord.com/channels/686873244791210014/1193648181465071616)).
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------
Sorry @Billy Howell, I seem to be having a bit of trouble. Don't worry though, I've pinged my support and they will try to fix me up! :technologist:
Perhaps try again in 15mins?after i've made these updates to my code, how to i push the changes? i got this error when i ran wasp start
Wohooo @Billy Howell, you just became a Waspeteer level 1!
@kapa.ai after i've made these updates to my code, how to i push the changes? i got this error when i ran wasp start
Let me check the knowledge base for you. One moment! :books:
Kapa gets things wrong,
emailAndPassword
doesn't exist, but the method is called email
: https://wasp-lang.dev/docs/auth/email#1-enable-email-authentication-in-mainwasp
Consult the docs for the specifics but here how I'd do it:
1. Add the email
login method and remove the usernameAndPassword
2. Add the username
field on the User
entity
3. Run some code that copies the username
from the AuthIdentity
to the User
's username
property (some useful code: https://wasp-lang.dev/docs/auth/email#creating-a-custom-sign-up-action and https://wasp-lang.dev/docs/data-model/backends#seeding-the-database)
4. Add the custom field on the signup form called username
: https://wasp-lang.dev/docs/auth/overview#customizing-the-signup-processDatabases | Wasp
Entities, Operations and Automatic CRUD together make a high-level interface for working with your app's data. Still, all that data has to live somewhere, so let's see how Wasp deals with databases.
Overview | Wasp
Auth is an essential piece of any serious application. That's why Wasp provides authentication and authorization support out of the box.
Email | Wasp
Wasp supports e-mail authentication out of the box, along with email verification and "forgot your password?" flows. It provides you with the server-side implementation and email templates for all of these flows.
thanks beast. I got it done! Couldn't get new DB migrated in Fly so I had to destroy it and create a new one lol