Accept number in string type
Hello! I'm parsing each value in a form with
JSON.parse
to convert string values like false
to actual booleans. Unfortunately, this introduces the issue, that if someone inputs 123
into a text field, it gets parsed into a number type by JSON.parse
. Then if the field is type string
. An error is thrown, since number is not assignable to string.
How should a type look like, if it should handle such a situation?120 Replies
Could you write a code snippet showing showing this?
Result:
What's this doing?
generateFormData
runs JSON.parse
on every value. The left-hand site is what it would return given the request
So.... just change
generateFormData
to not JSON.parse when the text field type is "string"?
This doesn't seem like an AT issueIt's not an AT issue, I am asking advice how it could be handled
Right, then this is how ig ^
I am converting it in such a way, since
multipart/form-data
has to send everything as stringsYou presumably have a way of knowing which input fields are going to be a text type
Yes
So just have your
generateFormData
function take that into account
In a very verbose way, something like
Or as one line, something likeI guess this could be use for the comparison
But will this also work when the type has contraints? (
string>0
)Possibly. I'm not familiar with the library you're using for forms
Yeah; why wouldn't it? This is all before ArkType
formType
is an Arktype type
It means that having a name of
"123"
is valid (which arguably shouldn't be a valid name, but I don't have the context etc.) but it will still apply the constraint of the length being >0
Oh I think I see what you mean now
Hmm
Let me get an ArkType environment openAnother issue is I can't use
formType.get('name')
, since not all types in the union have that key:
So I'd need to know the intent
in advanceI meant the actual form things
Not the keys within the ArkType type
Stuff from the
request
So like if you had the htmlthen stringTextFields
would be ["firstName", "lastName"]
I am using the
generateFormData
from here:
https://github.com/forge42dev/remix-hook-form/blob/main/src/utilities/index.tsGitHub
remix-hook-form/src/utilities/index.ts at main Β· forge42dev/remix-h...
Open source wrapper for react-hook-form aimed at Remix.run - forge42dev/remix-hook-form
Because (I assume)
formData
would be {firstName: "bob", lastName: "doe"}
Yes
Basically,
stringTextFields
is whatever the keys are in formData
that you know should remain a string typeBut that's a little redundant, when I have already created an Arktype type which says it should be string
It's not... because you're blindly doing parsing before AT
So your parsing has to handle stuff that otherwise AT would do
Or you don't blindly parse stuff and just leave everything to AT, which arguably makes more sense
You've effectively got
input -> parse to json -> ArkType
ArkType is designed to have no middle stepThat would be perfect...
Yes, it all feels wrong
And unsafe
One option is a morph, but there may be a better way
I'm not up-to-date on the latest types and unfortunately the docs don't list them (yet) π
And for some reason I can't get an ArkType environment working
Are you using
moduleResolution: "node"
? It was broken in last release
But I also released 2.0.0-rc.0
this morningI'm using the actual AT repo, so it's on whatever that uses
What issue are you having in the repo/

Yeah, I wouldn't know how to convert
multipart/form-data
inside of Arktype to handle booleans and arrays. Does parse.formData
handle booleans?(I'm on my json schema branch fwiw, but have merged main into it)
It's probably because I switched from paths to customConditions, how are you running the file
tsx ark/jsonschema/del.ts
tsx
was previously working iircYeah if you look at the scripts in package.json you will see how you have to run it now
You should just be able to use
pnpm ts
instead
And
pnpm tsc
fails with a whole load of errorsMaybe you have to build first I guess to get that
You shouldn't have to build to not see errors though
I think I may have just fked the branch :Shrug:
Let me try recloning the repo
Oof, big repo

It's really not big at all lol
Your internet is just being slow
A few months ago it was actually like 10x bigger I removed some GIFs from the history
My internet is the same speed as normal (just over 100mbps) :Shrug:
Lol
That doesn't mean it's downloading it at 100mbps
Maybe it's the server, my point is it's not the repo
Yeah, fair enough
Oh yeah

Yeah it helped a lot when I went through the history and removed all the files over a certain size
How do I get it to import arktype? π
have got this but
pnpm i
isn't working and if I just run it says it can't find the arktype
moduleIt's probably a corepack thing?
Ughh stupid corepack
(This is the
pnpm i
error)You can add this to scripts:
"ts": "node ./ark/repo/ts.js",
You need to update pnpmDo I need to be using.node 22.6+?
Only if you want to be able to run TS directly from node
I have tehe scripts set up to work with 20 and 18 also
Well it tried to fall back to tsx but that wasn't installed
tsx is installed globally
Pnpm doesn't respect that I guess you need to pnpm i
But I can't
pnpm i
Oh yeah, update pnpm
Damn
Node actually had a solution to this BS but they just killed it https://www.youtube.com/watch?v=I7qMwaxNNOc
Theo - t3β€gg
YouTube
Corepack is dead, and I'm scared
The removal of Corepack from Node is effectively a death sentence for the project, and I'm not pumped about it.
SOURCE https://socket.dev/blog/node-js-takes-steps-towards-removing-corepack
Check out my Twitch, Twitter, Discord more at https://t3.gg
S/O Ph4se0n3 for the awesome edit π
Yeah, I saw that video π
I'm kinda lost π
Like this would 100% solve your problem if it was enforced by default
But you should just be able to
pnpm upgrade && pnpm i
Okay I might have lied about what upgrade didIt failed anyway :KEKW:
Maybe just try pnpm add -g pnpm
I'm using corepack to manage it now but I guess I'll have to switch off
pnpm add -g pnpm
is what I always did in the past when I wanted to upgradeDoesn't look like it works :Shrug:
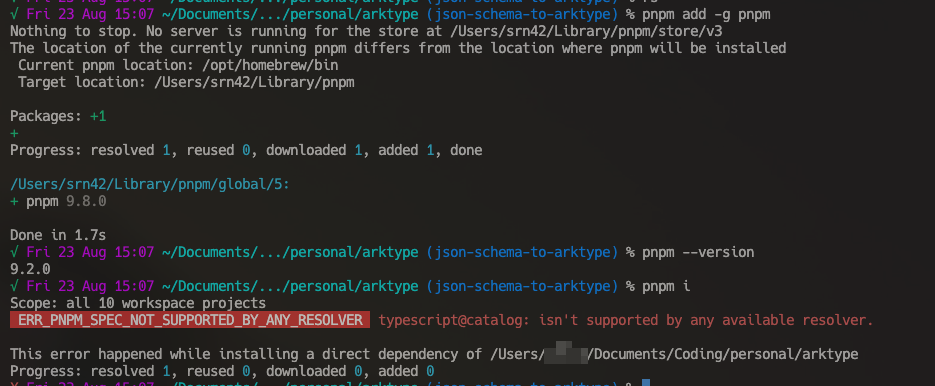
Wait what???? πππππ
Can I ditch tsx now?
Oh you're using homebrew and you still have an old version
Lol I can't debug your env
Theo - t3β€gg
YouTube
Node FINALLY Supports TypeScript
Huge! Can't wait for the new Node release to enable type stripping with --experimental-strip-types
SOURCES
https://github.com/nodejs/node/pull/53725
https://github.com/tc39/proposal-type-annotations?tab=readme-ov-file
Check out my Twitch, Twitter, Discord more at https://t3.gg
S/O Ph4se0n3 for the awesome edit π
Woooooooooooooaaaaaaaaahhh π€©π€©π€©π€©π€©
I would keep it around a bit longer but I was able to get AT's unit tests running in ~30 minutes
I am tired of registering ts-node and how workers run when they are written in TS
I mean
tsx
is pretty great as far as an external solution goes
But yeah very cool to be able to run it natively
It's so weird in the debugger it literally just displays the file with all the type annotations replaced with whitespace lolThanks; I really should pay more attention to terminal output π
Looks like it worked
Why is Theo respected?
(Sorry @PIat, I totally took over this channel π
)
From what I've seen he has pretty nuanced takes on a lot of topics, I like him. He even did a video on attest
I'm sorry you guys have to go through all this because of me π¬
I don't think that's what happening I think was inevitable based on Tizzy's env clusterfuck
So he's purely a YouTuber?
Not exactly
But I mean yeah he's generally a content creator, but I think if done well it serves a really valuable role in the ecosystem
Yeah, it would've happened anyway when I continued working on https://github.com/arktypeio/arktype/issues/729 π
GitHub
Support JSON-schema as an input format Β· Issue #729 Β· arktypeio/ark...
This would be a large feature allowing JSON schemas as an alternative definition format, that would be statically validated and inferred, similarly to the primary TS-based syntax. Some additional i...
Yeah will be interesting to revisit that now that I have some of the infra setup for the output types
I see, thank you
GitHub
Incorrect discriminated union processing Β· Issue #1033 Β· arktypeio/...
Report a bug π Search Terms boolean unioned with a recursive discriminated union, recursion, recursive discriminated union π§© Context ArkType version: 2.0-dev.26 TypeScript version (5.1+): 5.5 Other...
Just find a workaround haa
If you want to work on it
There are other ways
Yeah, my time is better spent elsewhere atm anyway tbf
As in, I'm too busy to work on it atm anyway
More like worse spent :kappa:
@PIat To finally answer your question, this seems to work:
You just need a way of determining what
keepAsString
should be, which from the sounds of it you know so isn't an issueWould it somehow be possible to extract keepAsString from the
formType
? I've been looking into that for a while nowSort of. You could look at the internal schema structure
See where the
domain
is "string"
, or it's a unit of type string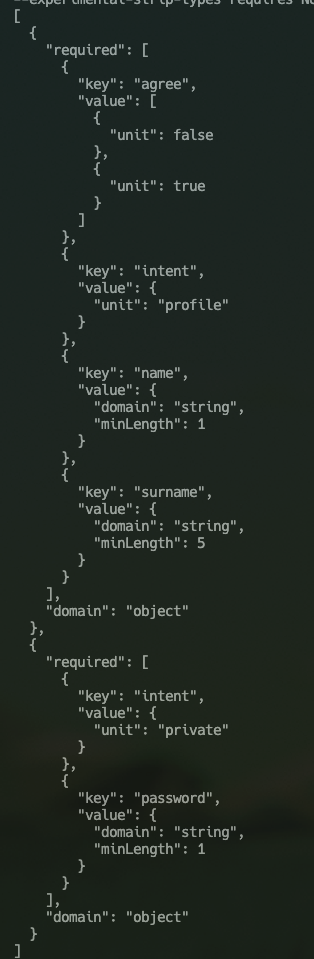
(That json being
formType.json
)
@PIatThank you! This is what I ended up with:
Will see what kinds of issues this will yield in the future π¬
This is so sketchy haha I wouldn't rely on this. You can use
node.internal
to get access to a bunch of APIs that are useful for this kind of manipulation including .distribute
which maps over the branches of a typeYeah I tried to understand those methods but failed miserably
A lot of them just mirror the TS APIs
Oh, I didn't think of it that way
keyof
pick
omit
required
and partial
all of which are available on object types are just like TS
And then extract
and exclude
are on every type, which is very useful for filteringWhat is
BaseRoot
?
BaseRoot is what type is internally
It's kind of like type without baked-in inference and with a bunch more powerful transformation methods, compilation etc.
I see! But why is it returned from
keyof
?Because that's what
type.internal is
Once you're in internal
, you're not going back to type
I'm adding .distribute
to Type in the next release though so you won't have to lose inference to use itOh wow
Does using
node.children
make sense with distribute
?Not really, distribute is for iterating over branches ofa union
If it's not a union, the root node itself would be the branch
And each branch is a BaseType
Yeah each branch is more specifically any BaseRoot that is not a union
This is so cool
If you want to see a summary of each node you're looking at
.expression
will be usefulI'm actually trying to understand typescript for the first type, and it feels useful now, since I'm using the same knowledge in the runtime as well
Yeah that is a really unique new capability now that ArkType exists
It's insane
A real bridge between static and runtime
That's what the whole branding on the homepage is about! An ark that saves your types from the flood of compilation haha
I do see it give such a value, but have trouble finding out how to check if password is string. I don't see the way to check the keys
I can't wait till I understand it fully βΊοΈ
.get
is like index accessIt'll be wroom wroom
Even now it's the backbone of my whole job messaging system
Well you should definitely never need to use try catch haha
I don't know how much worse this is than the json solution π
It's to prevent
What about this:
Rigggghhhhtt π«
It makes sense
Careful though a lot of expressions will throw by default if they create an unsatisfiable value so you don't accidentally create validators that will never pass
Thank you! I still have a lot to learn
Well I appreciate you delving into the type system aspect of things. I'm still really the only one with much context on that stuff! Luckily working on docs now βοΈ
Already? π΅
I'm living in the best timeline π
Once they're published that will be true haha