query with
I'm trying to do this query, but "with" doesn't recommend anything, did I link my schema wrong?
8 Replies
You need to define relations for “query” api. Did you?
https://orm.drizzle.team/docs/rqb
Drizzle ORM - Query
Drizzle ORM is a lightweight and performant TypeScript ORM with developer experience in mind.
mmm let me check
It is not enough to establish the relationship in the schema? Do I need to do something else?
haha
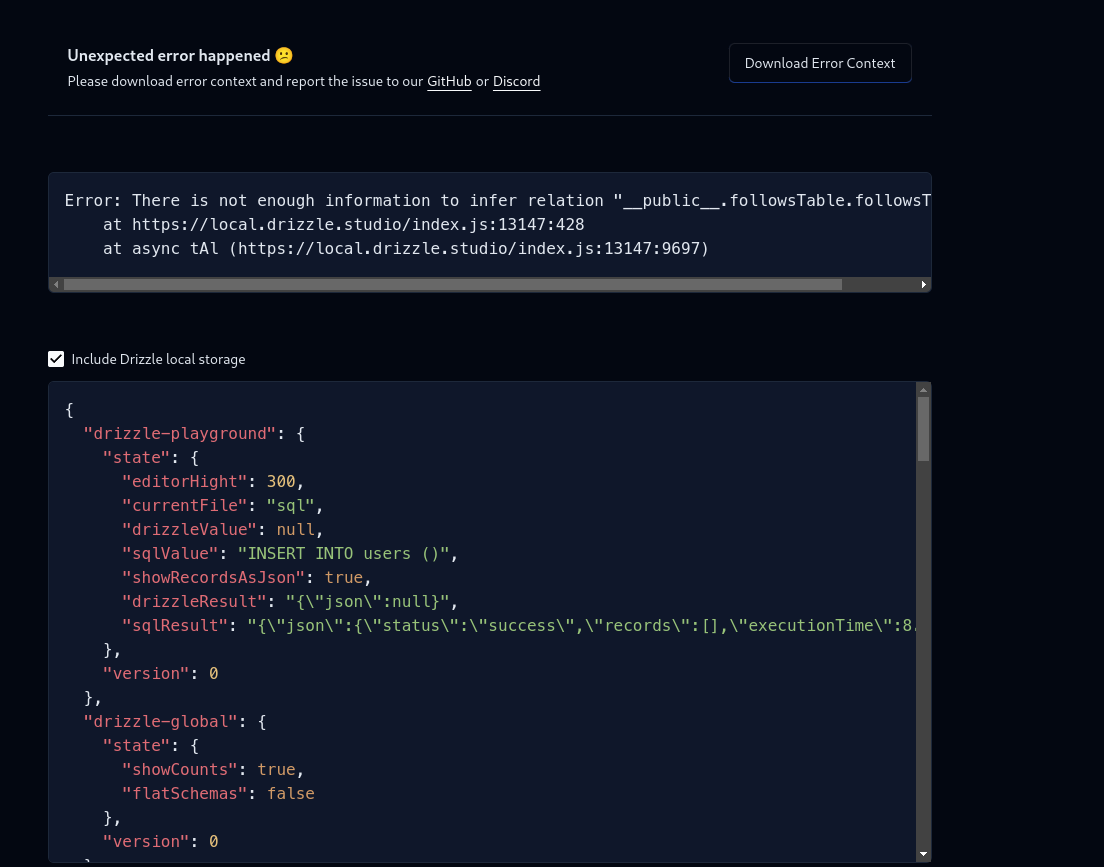
after
but
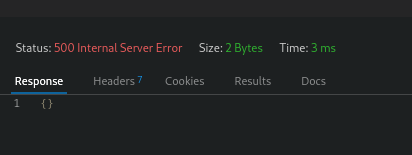
If I remove with it works, if I put with it doesn't work
@edarcode Hey! Are you still having this error in Drizzle Studio?
That same day I closed the browser, relog server and it returned to normal