Getting a 404 on our test API route
Trying adding an api route like so:
api inference {
fn: import { inferenceHandler } from "@src/server/api/inference.js",
middlewareConfigFn: import { inferenceMiddlewareFn } from "@src/server/api/inferenceMiddleware",
httpRoute: (POST, "/api/inference"),
auth: true
}
No errors are given in the output of "wasp start" however the route is 404 when we try to access it.
17 Replies
Hi @Conspyre. I'm kapa.ai, your friendly support bot. I will try to answer your question by searching through the knowledge base. :wave:
----------------------
Based on the information provided, it's hard to pinpoint the exact issue as there could be several reasons why you're getting a 404 error. However, here are a few things you could check:
1. File Existence: Ensure that the files
inference.js
and inferenceMiddleware.js
exist in the @src/server/api/
directory and that they are correctly exporting inferenceHandler
and inferenceMiddlewareFn
respectively.
2. Middleware Function: The middlewareConfigFn
should be a function that configures Express middleware for this API. Make sure it's correctly implemented. You can find more information about it in the Wasp documentation (https://wasp-lang.dev/docs/project/server-config#middlewareconfigfn-extimport).
3. Route Order: If you have a wildcard route (a route that catches all paths), make sure it's the last route in your main.wasp
file. This is because routes are matched in the order they are defined and the wildcard route could be catching requests before they reach your inference
API route.
4. Authentication: You've set auth: true
which means Wasp will attempt to parse the JWT in the Authorization Header and provide a context.user
object. If the JWT is not present or invalid, it could lead to a 404 error. You could try setting auth: false
temporarily to see if authentication is causing the issue.
If none of these suggestions help, it would be beneficial to check the server logs for any error messages or warnings, and consider opening an issue in the Wasp Github repository (https://github.com/wasp-lang/wasp/issues) for further assistance.
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------
Sorry @Conspyre, I seem to be having a bit of trouble. Don't worry though, I've pinged my support and they will try to fix me up! :technologist:
Perhaps try again in 15mins?@kapa.ai Update: we are trying to use "await axios.post(...)" to access our API route. This seems to be the issue, but I'm not sure how we actually call the API route. We've gotten another API route which can be accessed externally, given as a webhook to another app - its working just fine - I am talking about the Stripe webhook API in the SaaS sample app.
We've tried building an api endpoint, which we want to call from the React side of the app. How do we do that?
Let me check the knowledge base for you. One moment! :books:
----------------------
To call an API from the client side in a Wasp application, you can use the Axios wrapper provided by Wasp. Here's how you can do it:
In the above code, replace
{ /* your data here */ }
with the actual data you want to send to the server. This data will be sent as a JSON object in the body of the POST request.
This method can be used to call any custom API endpoint from the client side of your Wasp application. The api
object is an instance of Axios that's pre-configured to make requests to your server, so you can use any Axios method on it (like get
, post
, put
, delete
, etc.).
For more information, you can refer to the Wasp documentation on using the API from the client (https://wasp-lang.dev/docs/advanced/apis#using-the-api-from-the-client).
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------
Sorry @Conspyre, I seem to be having a bit of trouble. Don't worry though, I've pinged my support and they will try to fix me up! :technologist:
Perhaps try again in 15mins?Update: @kapa.ai you solved it. U dat bot! Here, have some 🍪🍪🍪
Wohooo @Conspyre, you just became a Waspeteer level 1!
Let me check the knowledge base for you. One moment! :books:
----------------------
I'm glad to hear that your issue is resolved! Enjoy developing with Wasp and don't hesitate to ask if you have more questions. And thank you for the cookies! 🍪🍪🍪
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------
Sorry @Conspyre, I seem to be having a bit of trouble. Don't worry though, I've pinged my support and they will try to fix me up! :technologist:
Perhaps try again in 15mins?
Hey. Is this API route that you're calling from your client also need to be exposed externally?
I'm just wondering why a Wasp Operation wouldn't suffice here.
Awesome! @Conspyre could we have done something to make this easier for you? Maybe improve docs somehow, or improve our SDK/API somehow?
@Vinny (@Wasp) it does not need to be exposed externally, I probably just need more understanding what a Wasp Operation is.
@martinsos I'm just learning this with the LLM, and the bot insisted on using an axios.post to hit the api endpoint so that threw me into a ditch for a couple of days… I don't think this bit (import { api } .....) was in the docs, if it is I missed it. 😦
@Vinny (@Wasp) @martinsos I just had a thought, if there were a condensed version of the docs (text only) that were in one file, we could one shot that into the LLM's context. That would be dope.
@kapa.ai how could I turn this API endpoint into a Wasp Operation?
Let me check the knowledge base for you. One moment! :books:
----------------------
To turn your API endpoint into a Wasp operation, you would need to declare an action or a query in your Wasp code. Here's an example of how you can do it:
In the above code,
inferenceHandler
is a function that you would define in your @src/actions
directory. This function should be a Node.js function that takes two arguments: args
and context
. The args
object contains any data you pass to the action from the client side, and the context
object contains information about the current user session and entities.
Here's an example of what the inferenceHandler
function could look like:
Once you've declared and defined your action, you can call it from the client side like this:
In the above code, replace { /* your data here */ }
with the actual data you want to send to the server.
For more information, you can refer to the Wasp documentation on actions (https://wasp-lang.dev/docs/data-model/operations/actions).
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------
Sorry @Vinny (@Wasp), I seem to be having a bit of trouble. Don't worry though, I've pinged my support and they will try to fix me up! :technologist:
Perhaps try again in 15mins?you can also use Cursor, and then add the URL to the wasp docs. Then in the chat, you can do
@Docs
-> Wasp
and include the docs in the chat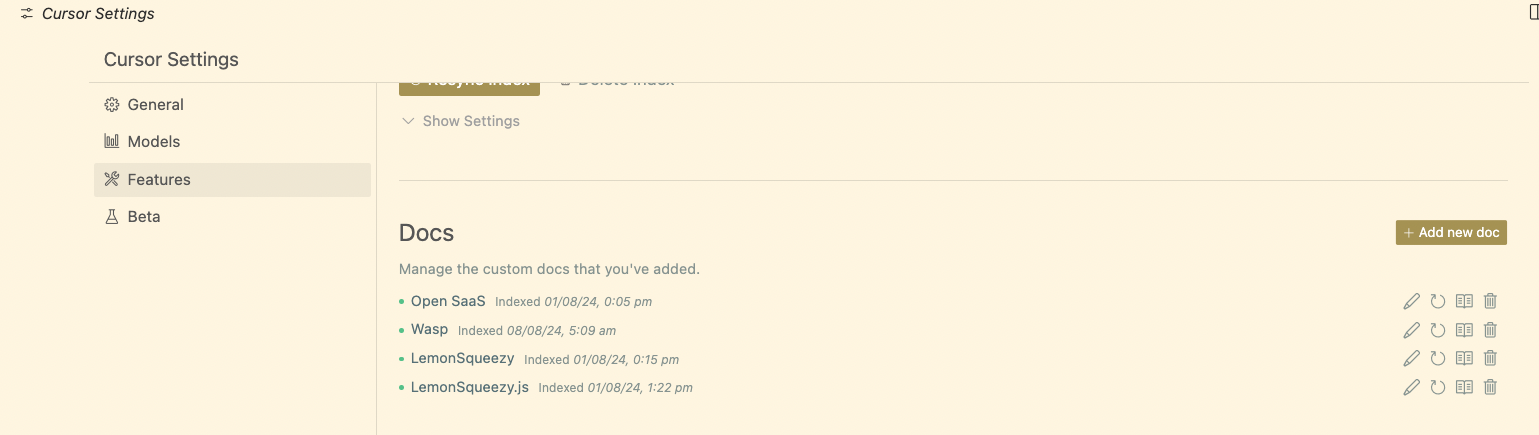
Otherwise Kapa does a pretty good job
Yeah it could be fun to have whole docs in a format that is good for LLMs. Ideallky already chunked so it can be nicely used in RAG. Or maybe already as a RAG database.
I just checked, we do have section in docs on how to use API from client: https://wasp-lang.dev/docs/advanced/apis#using-the-api-from-the-client -> maybe it was a bit low in the doc? I but get if you used the bot that you probably didnn't read that part.\
Custom HTTP API Endpoints | Wasp
In Wasp, the default client-server interaction mechanism is through Operations. However, if you need a specific URL method/path, or a specific response, Operations may not be suitable for you. For these cases, you can use an api. Best of all, they should look and feel very familiar.
Tnx for the feedback in any case!
I can see I need to give Cursor a spin (and stop letting the Bot read everything for me - but - that's where speed comes from lol). Thanks for the followups! 🙂