Using a FileUpload input in a Custom Page
Hello everyone!
I have created a custom page with several forms, all of which work perfectly, except for one.
What doesn't work is a form with two FileUploads, one for an article cover, the other for multiple other images.
Doing a
dd
of the getState()
I get this result, but honestly I was expecting an instance of FileUploaded (or whatever it's called), whereas now it's just returning strings.
Is this normal? On the page I called the WithFileUploads
trait.
My code so far:
Thanks everyone!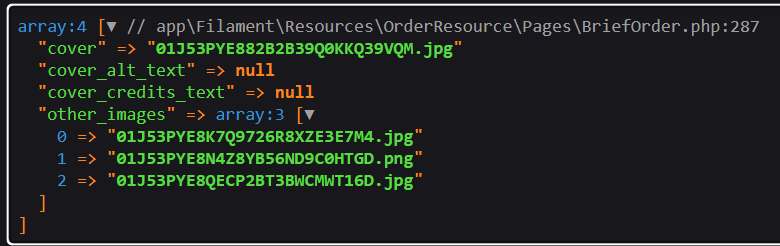
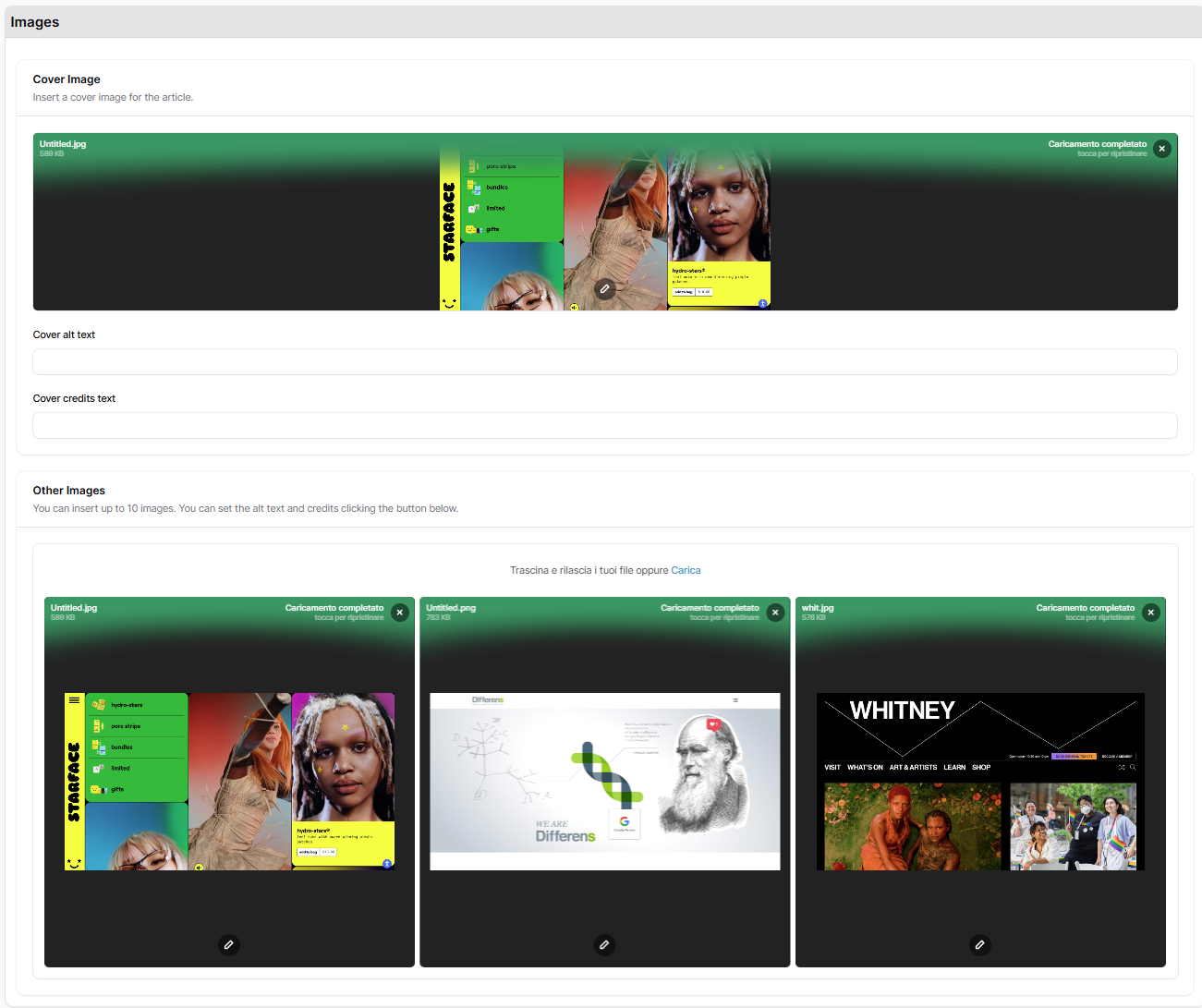
Solution:Jump to solution
Ok, found the problem 😄
I had to use the
imagesData
array, and not the getState()
method....14 Replies
Solution
Ok, found the problem 😄
I had to use the
imagesData
array, and not the getState()
method.But now I have another question. How can I fill the
imagesForm
?
In my mount()
method I have:
and the array is correct. But the FileUpload inputs remain blankNot an expert at filament, but happen to run across to your issue.
Does this return you a path or a URL?
'cover' => $this->brief->briefImages->where('is_cover', true)->pluck('path')->first()
Hi Ed, thank you for your reply.
It returns a path:
If I use the
Storage:url()
method, which returns:
I have the same results.
The other fields (cover_alt_text and credits_text) work as a charmWhat if you try removing
public
from the value like so:
"cover" => "briefImage/KyzIWU8hnDQQdrlWGqI9PMAwFGh9oiCd4TVhSJqj.jpg"
Does that then display anything in the FileUpload
comopnent?Yes, but no 🤣
It recognized the image, but in my console:

Haha! Okay, step closer.
My next question comes to mind is, if your image is stored locally or on cloud?
Locally, in Storage
In your
livewire.php
, can you set your APP_URL to your localhost port number e.g.:
'app_url' => ':8000',
Then stop your sever, run the following command:
php artisan storage:link
And try again 🙂It's already like that. Other fileupload inputs work as intended 🤔
Instead this one is in a custom page
Hmm, I'm not sure on this one sorry!
I had a similar issue as you, by only showing the grey box with no preview image.
I then added my port number to
app_url
, ran the command, and it finally showed the image.
I was navigating on 127.0.0.1:0000 and not in localhost:8000 as my APP_URL...
Well, at least now it works 😄
Thank you so much for your help Ed!
Oh very glad you managed to sort your issue! 🙂
Thanks for helping me, I had the same Problem :lecker: