✅ Custom `IComparer` adds so much overhead
Is there a way I can avoid having to make a whole new class for the
IComparer
interface here?
https://dotnetfiddle.net/yRh9YN
C# Online Compiler | .NET Fiddle
Test your C# code online with .NET Fiddle code editor.
16 Replies
I have a
SortedList
of roman numerals where I want lower value numeral to appear earlier in the sorted order.
I do not want to insert based on their numeric value, but rather via a custom IComparer
interface.
just for the fun of iti don't see where the overhead is, there is a big method body which is more or less irreducible and there are 4 lines of extra code for the class
you can use
Comparer.Create
to save a couple of those lines i guessIt's a little neater @reflectronic
But I think it gets put into a class anyway
I wish the IL viewer in rider wasn't so dumb, I am trying to see if the compiler is putting it into a hidden class
I guess it does not create a hidden class.
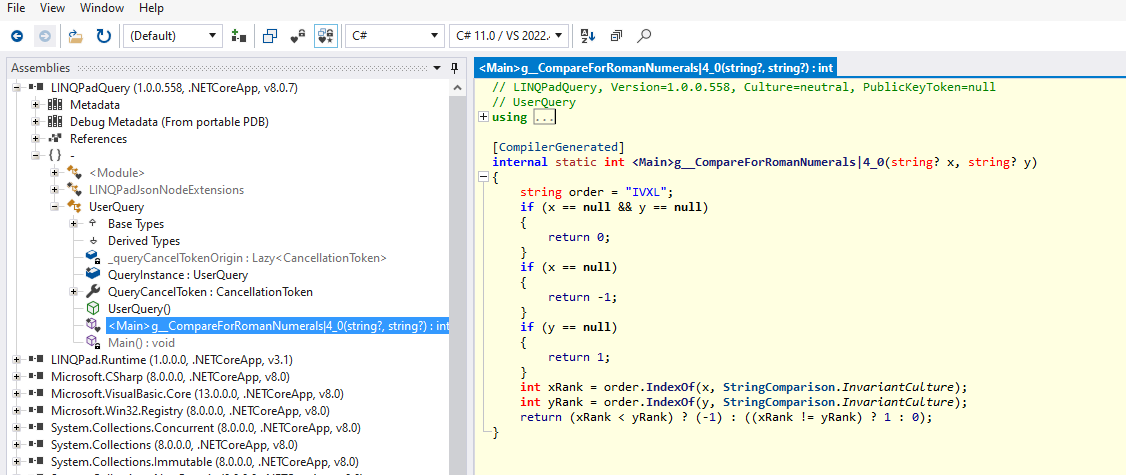
I thought everything was in a class in c#
a method is not a class
I know, but I thought it would put it in a hidden class.
Or maybe I am thinking of java
yes, maybe in java this is how functional interfaces are implemented
it is not how delegates are implemented in C#
a delegate object's constructor accepts the method directly
thank you @reflectronic
Now I gotta try to use a switch pattern instead of these old school
if
statements
Is there a way I can avoid having two var (xRank, yRank)
s here?
Can I someone name the expession preceeding the 2nd switch and use those names in the cases that follow?this doesn't work
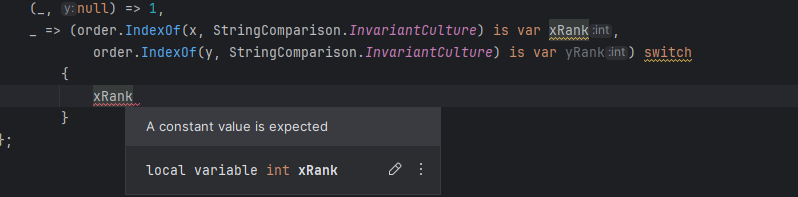
I know this is a crazy scenario but I want to avoid accidently flipping the names
(yRank, xRank)
i would just do
_ => order.IndexOf(x, StringComparison.InvariantCulture) - order.IndexOf(y, StringComparison.InvariantCulture)
That's clever and I thought about doing that but I don't know if it is readable at a glance. What do you think @reflectronic
I know you said that that is what you would do. How readable do you think it is?
It's one of those "tricks". Once you've seen it, you recognise it in future
Just be careful with it in general. It won't happen with
IndexOf
, but you can end up overflowing if you're dealing with large intsor
CompareTo
the indices, since int
is IComparable
thank you. I will use it
that would work too, thanks
Is there any overhead here that warrants switching from
CompareTo
to just subtracting the two values?