Default options of checkbox list
I have this section :
How can I compare the current checkbox id with the User resource role id to make it checked ?
34 Replies
Why do you need too? Your relationship should be pre-checked from the data that's being loaded?
can not use relationship hasMany on checkbox list
so you probably want getStateUsing opposed to formatStateUsing
I am in User resource, and use has Many roles and belongsToMany academies , I just want to display all roles of the academy and default select the user roles that he have
I solved it now by using
but IDK why the data is not sending to method
mutateFormDataBeforeSave
?Because your are not hydrating it
You set it but it's not be hydrated which is why I suggested getStateUsing
You could try using $set in afterStateHydrated?
Method Filament\Forms\Components\CheckboxList::getStateUsing does not exist.
@toeknee still can not sending dataWhat code are you using
Are you sure it is not sending the data? Have you got the model fillable filled for the column
this is not column , I just need to send the Ids of the rols then sync the Ids for thre User roles (Spaite roles)
roles not sending to the $data
@toeknee
are you using
relationship()
in roles?no
The idea that :
Academy has many roles,
And User belongs to many academies , Also user has many roles
I need to group be academy and for each academy display the roles of it . when in the edit page of user I can edit the roles of users for each academy that he belongs to.
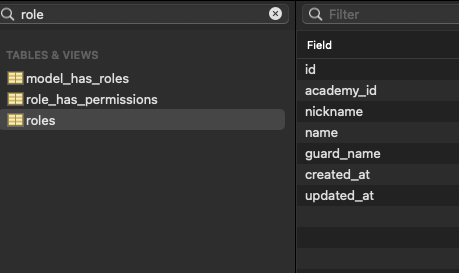
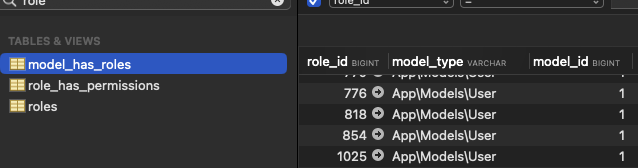
got it ? @Leandro Ferreira @toeknee
please read #✅┊rules

sorry
use
dehydrated()
in the academies repeater if you want to access the data in $data
arrayThank you ❤️
is there other simple idea to make this approach ?
using relation manager I think
https://filamentphp.com/docs/3.x/panels/resources/relation-managers
every thins is working now but data is not refreshed after save (its saved in DB):
try
$this->refreshFormData(['field'])
after savingok thanks but
afterStateHydrated
still have problem on it, I need to set the default checkboxes checked IIF ID of the checkbox in the
$roleIds = $form->model->roles->pluck('id')->toArray();
->default()
is not working with CheckboxList
default only works in creation not edit
so, how to auto select ?
you pass them in on mount, you shouldn't be maniplating data in and edit form really
I just need edit form only
how can I fill the data in mounted ?
Is this on a resource or a custom form
in resource
->dehydrated()
it will never save with this on it.
but the data(Roles) is not sending when saving
It won't because you have set: ->dehydrated() which means it will send nothing.
So what should I do ? any recommendation
remove ->dehydrated() and it should be set
set in which method ? also I need the adding the default values which is ($form->model->roles->pluck('id')->toArray();)
to clarify the idea
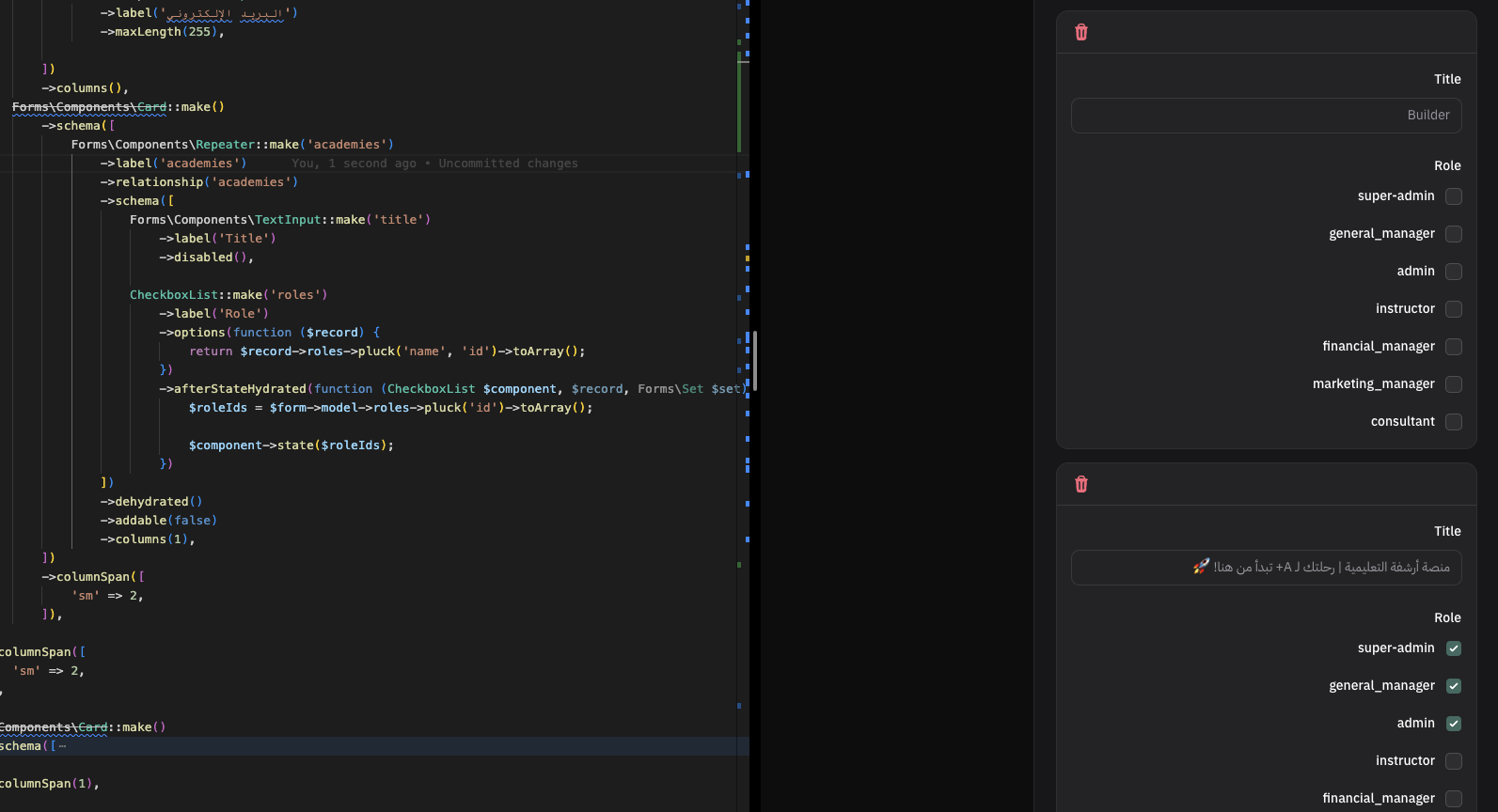
I need to use dehydrated here