162 Replies
can someone explain the fix here?
Console.ReadLine returns a string
You would need to convert it to an int first
how do i do that?
int.TryParse()
is the most proper wayAlso you don't need the if statement if you compare the same value
This
Followed closely by
int.Parse()
int.Parse is if you're sure the input is a number
TryParse If you want to double check
:SCfeet:
anything coming from a user should obviously not be trusted
int withDrawl = int.Parse(Console.ReadLine());
this?
Yes!!!
well yeah, but if I am a meanie and type "cheese" your program will crash
so thats where
int.TryParse
comes in:SCcheese:
your program suddenly has whats called a "fail state", or "unhappy flow"
there are two cases, the user might enter a good value, or a bad value
you need to handle that bad value properly
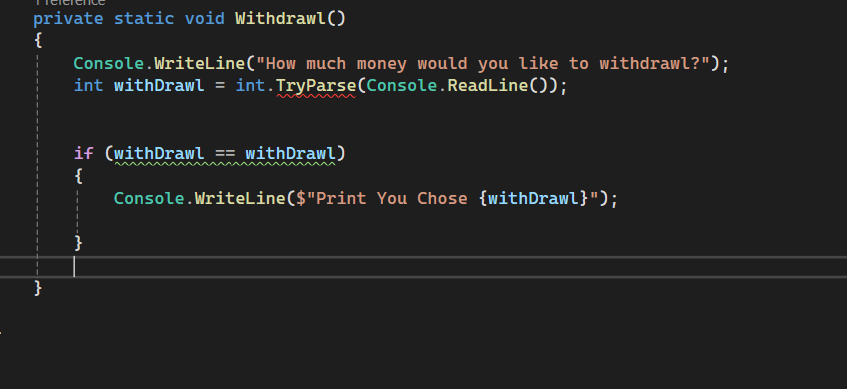
? how to do TryParse
$tryparse
When you don't know if a string is actually a number when handling user input, use
int.TryParse
(or variants, e.g. double.TryParse
)
TryParse
returns a bool
, where true
indicates successful parsing.
Remarks:
- Avoid int.Parse
if you do not know if the value parsed is definitely a number.
- Avoid Convert.ToInt32
entirely, this is an older method and Parse
should be preferred where you know the string can be parsed.
Read more hereyou seem to be struggling with the concept of return types in general. I highly recommend reading up on what a strict type system is and how to work with it
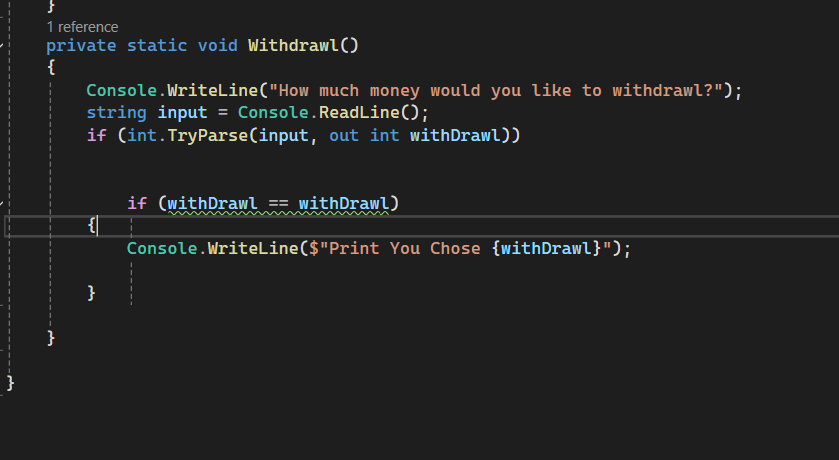
whats the purpose of that second if?
i just didnt delete it
if (a == a)
is a fairly silly comparisonYou can never be too sure.
Should be
if ((a == a) == true)
then :when::SCgetoutofmyhead:
I wonder how far this can go before it becomes unreadable
lets not
Lmfao
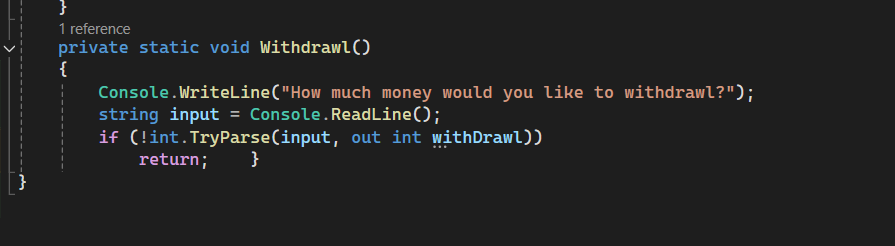
that'll work - but please fix your formatting š
can u show me proper formatting?
$prettycode
To format your code in Visual Studio, Visual Studio Code, Rider, use the following shortcut:
NOTE: the first key must be held while doing it.
https://cdn.discordapp.com/attachments/569261465463160900/899513918567890944/2021-10-18_01-26-35.gif
ctrl+k, ctrl+d
The IDE will do it for you
Is there a list for all those speed dial commands.
well yeah, the VS keyboard shortcut menu
Nono I mean for the bot
go to mod.gg
its there, called "tags"
Bet
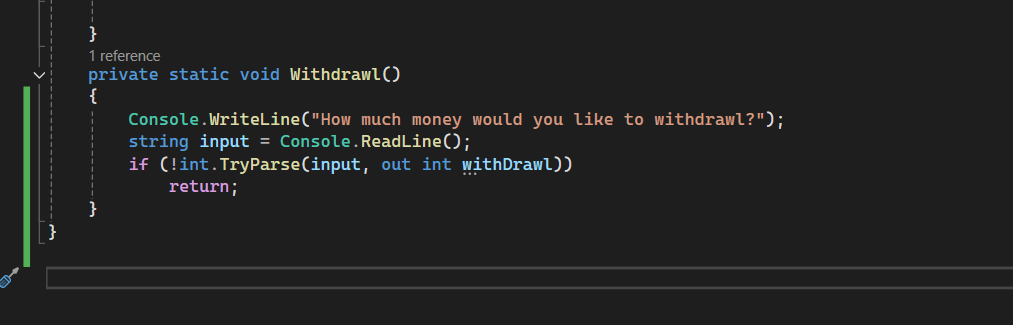
much better.
doesnt look much different
lmao
Would've looked better with braces
well, your methods closing brace is now properly aligned AND on its own row
which it wasnt before
so... thats a big improvement for readability
No Page access
Gotta log in with Discord IIRC
yep
Ah
should be a "log in" button in the top right
Yes yes
fair warning, there are 941 tags atm
so you have a bit of browsing to do
:SCshocked:
PasteCode
bank449494 - PasteCode.dev
PasteCode.dev is a website where you can store any code online for quick sharing. You can paste code and share code online for free.
Isn't there a tag for like rule 6 or smth specifically
heres code i got rn
We have a lot of useful and less-so tags lol
$chat
got some unused
using
statements. your IDE should be able to clean those up for youyeah i only need using system; and system.Threading; when i made my project it auto added them
soo lazy to remove
as said, the IDE can do it for you. Just click one of the gray ones and pick "remove unused usings"
ok
I'd recommend removing your
thread.sleep
s thou
artificial pauses are just annoyingokay
its so text can be read
better to have a "press any key to continue" then imho
what if 5 seconds wasnt long enough? what if Im ready to continue in 1 sec?
press any key works for both of those scenarios, thread.sleep doesnt
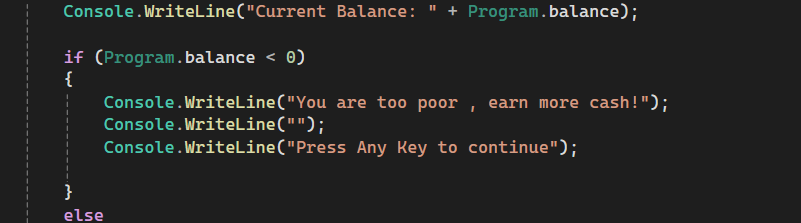
il add this to a few areas
What if the user isn't ready to continue at all after seeing their bank balance.
My brain isn't braining atm but I think this only takes effect when the balance is below 0
So like -1 and such
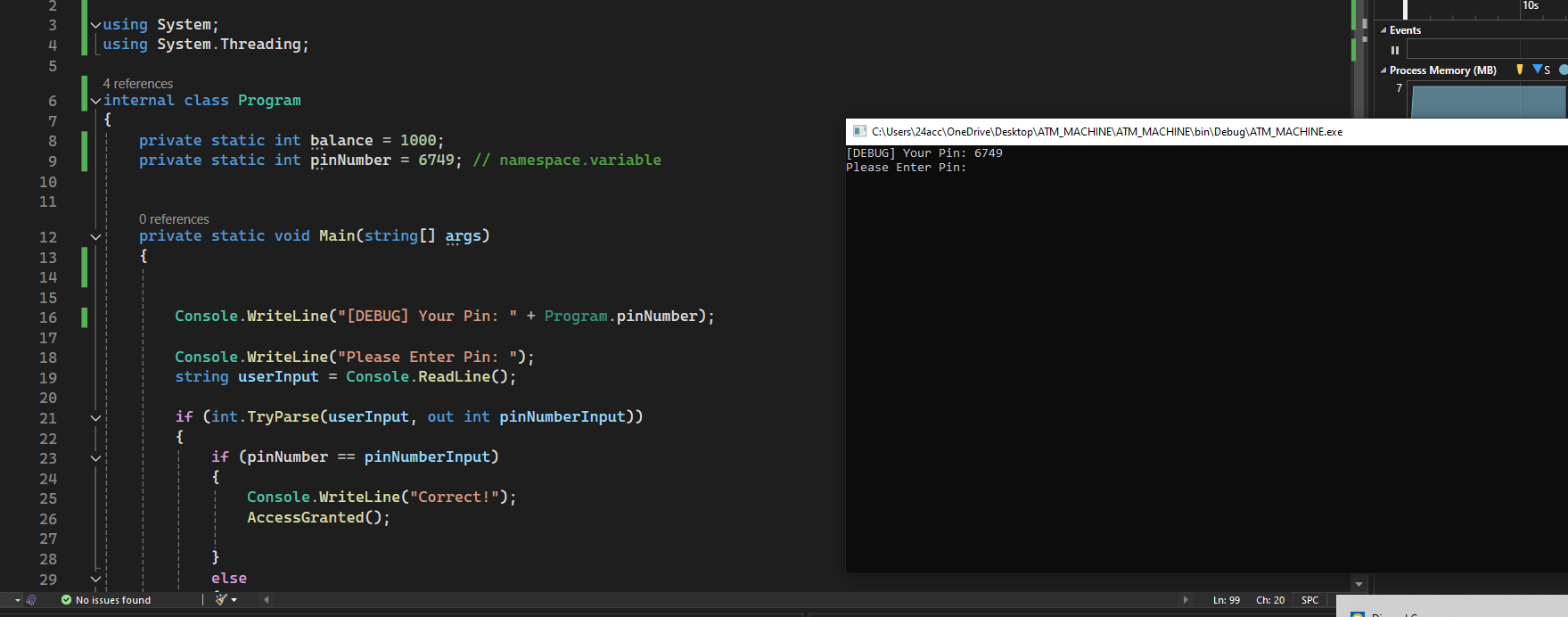
how do i make it where i text on same line
right after the :
it makes me type under
Use
.Write()
not .WriteLine()
I think you need to do some shenanigans with Console.Write since WriteLine will break the line
The latter appends a newline at the end of the string
okay good got that looking better, now how can i make it make mutliple ways of spelling in the string
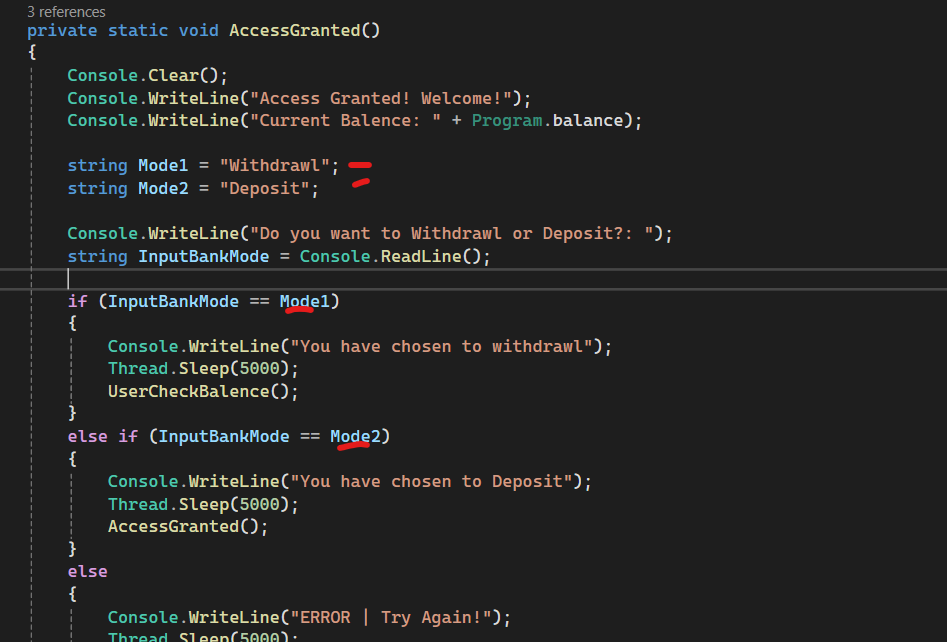
string Mode1 = ["word1" , "word2"]
like that?You could use a string comparison that ignores case
Or you could use
||
for logical or
Or you could use or
for pattern matching
* str.Equals("word", StringComparison.OrdinalIgnoreCase)
* str == "word" || str == "WORD"
* str is "word" or "WORD"
The first one will cover "word"
, "Word"
, "wOrd"
, "woRd"
, "WOrD"
, and all other permutations
found this way
Ah, that's what you mean
Then yeah, an array can store multiple values
the IDE has an area to see github examples which helps
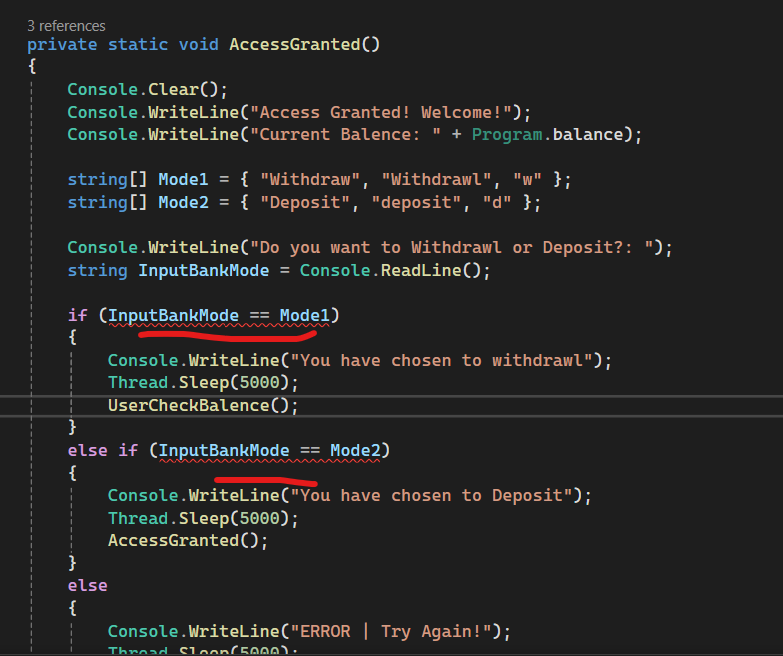
well now underlined
Well, yeah, can't compare an array of strings to a string, can we?
Because you try to compare a string to an array
You can check if an array
.Contains()
a given elementArray probably has a function called
Contains
or smth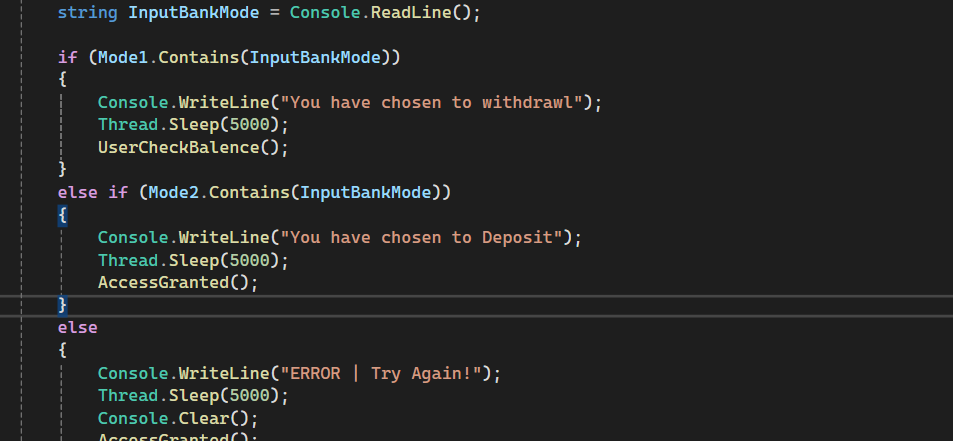
got it
btw, if you notice that you are doing a certain thing over and over, putting that code in its own method is a great idea. for example, printing a message and then expecting the user to return a value
i know im making this have alot of crap trying to force my self to memorize this stuff
thats fine.
practice is very important
Ye
I like how this auto goes to exe too
python could never
Python Bad
:soPortuguese:
tired of using that lang
useless
Yes!!!
Become CSharper
Make life easier.
languages are like tools. some tools are better for certain jobs
python is imho a great scripting language, but not a great choice for full on program development
i really wanted to learn c++
too complicated
:SCwhatiscatwant:
The issue is that it's being shoved down the throats of beginners and then they get overwhelmed by X amount of packages and eventually start to learn third party dependencies more than the actual language
agreed
Python is a very lovable language indeed but it's so horribly taught :SCgetoutofmyhead:
I'll never forget sitting down in the first programming class in college, after having tried some PHP and covering LOGO at school... and starting with fucking pointers :KEKW:
i only used python for Discord bots
What's LOGO :thinker:
yeah I'm pretty glad I already knew C# and PHP before I started with C in uni
fucking pointers.
and pointer arithmatic
You'd probably love Discord.NET or the other lib some find is a better choice but I don't know the name rn
we have a dedicated channel for discord bot development
#discord-dev
You have a turtle that leaves a trail, and you give it instructions like
FORWARD 6
ROTATE CLOCKWISE 70
Ah that thing
LIFT PEN
YeahWriting a discord bot in C# would be weird... love it
I personally am a huge proponent of the Remora discord library, but its... fairly technical
MODiX is open-source if you wanted a peek
We use Java in school, it's chill but we use Java 6 or smth and we use a weird ass editor
Luckily I got IntelliJ setup on the school laptop and my personal one
Eclipse or Netbeans?
let me guess, BlueJay?
It'll be much easier
We had blueJ before
Horrible
The current one is weirder
I don't remember the name
Java Editor is the name
Just that
BlueJay is... actually genious, but you shouldnt learn it and only it, since its so... different
ftr, bluejay lets you create instances of objects and call methods via the IDE gui, bypassing "Main" entirely
great for testing stuff, but horrible for learning how to build actual programs
Our current topic is SQL and databases which I'm kinda excited about since our last Java assignment I finished in the first lesson and had nothing else to do for like 4 weeks :SCgetoutofmyhead:
how do i call this?

wdym?
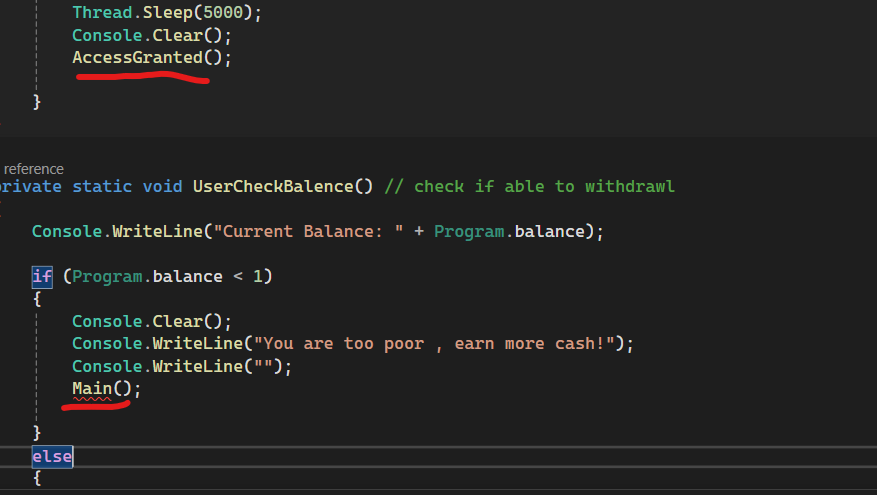
You don't manually call Main, ever
How should i go about restarting?
A loop, usually
with a loop
A
while(true)
for example
Or better, a
okay i see
what about colors for specfic text
Console.ForegroundColor
IIRCnonono
yes yes yes
like for example i only want the int green and other text on same line default
Huh?
Ah
Well
[green]500[/] moneys remaining in your [yellow]bank account[/]
thats NOT how you do it btw, but thats what you are trying to make, right?
Is how you'd do it vanilla
I'd write a helper method
Yeah
Or
Spectre.Console
like word blaze to be green it would be red
Then like what I wrote
Or like Pobiega's helper method way
Or with the
Spectre.Console
package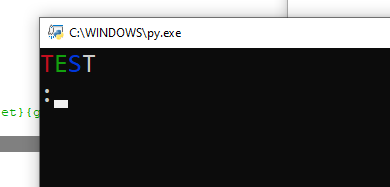
spectre actually supports the syntax I used above, with the colors inline in the string
but you can easily make your own version of it, as demonstrated above
Yes, I know what you mean
My answer stands
and I agree with you š
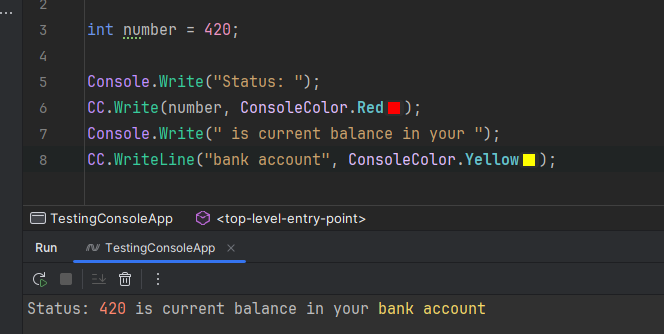
just to demonstrate how it could look with a helper

got it
don't forget to reset the colors
i did
ah right
still, thats now 3 rows that could be turned into 1 with a simple helper method
if you plan to use colors a lot, or just in more than one place, thats a lot of extra rows
I just wanted to add it for testing
but ill remeber that for later
doing t he withdrawl rn
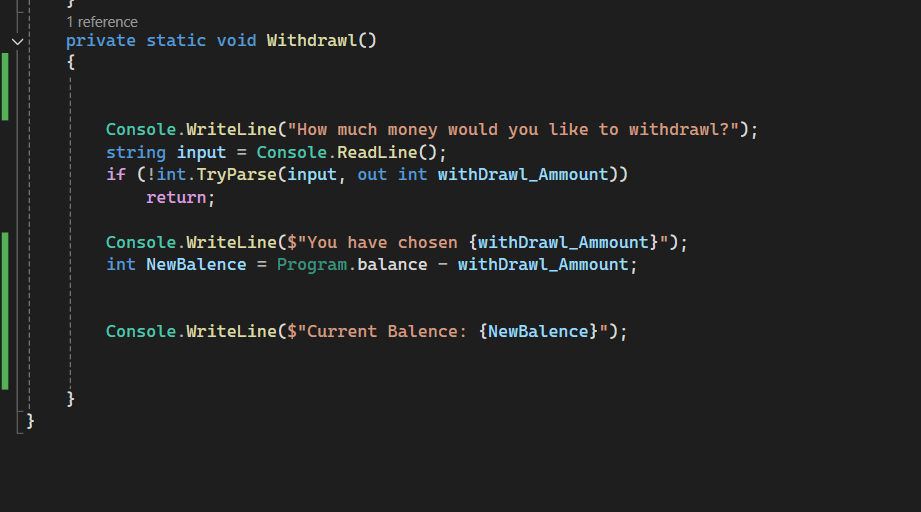
i think math is fine
idk if theres better ways
you are not saving the new balance there
and you can also withdraw more than you have
or even a negative number
okay
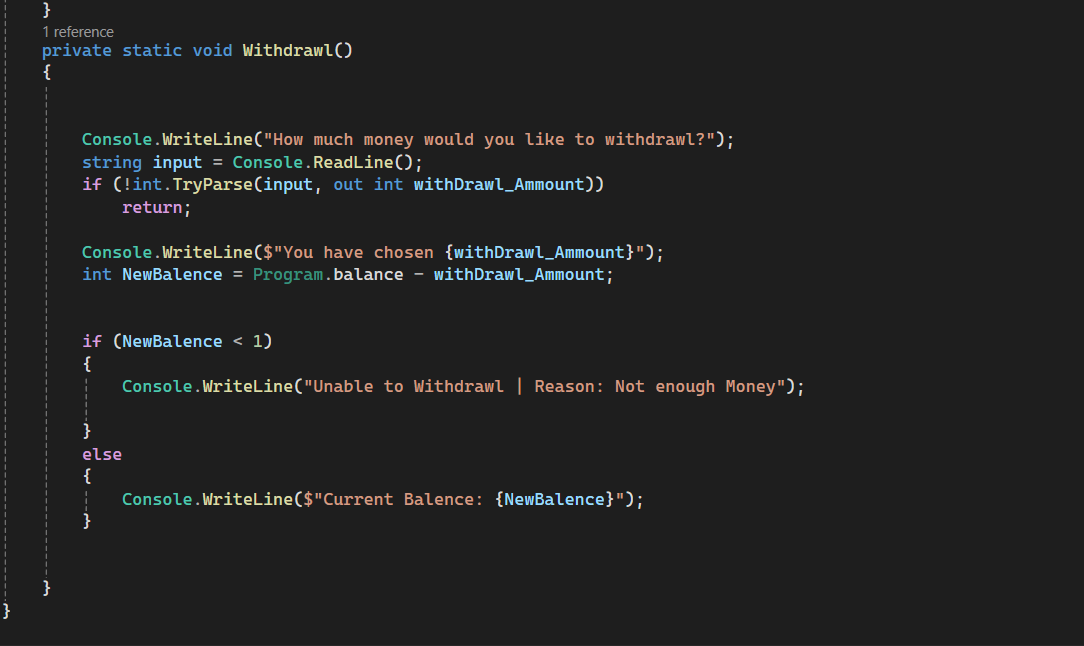
you need to handle both these cases
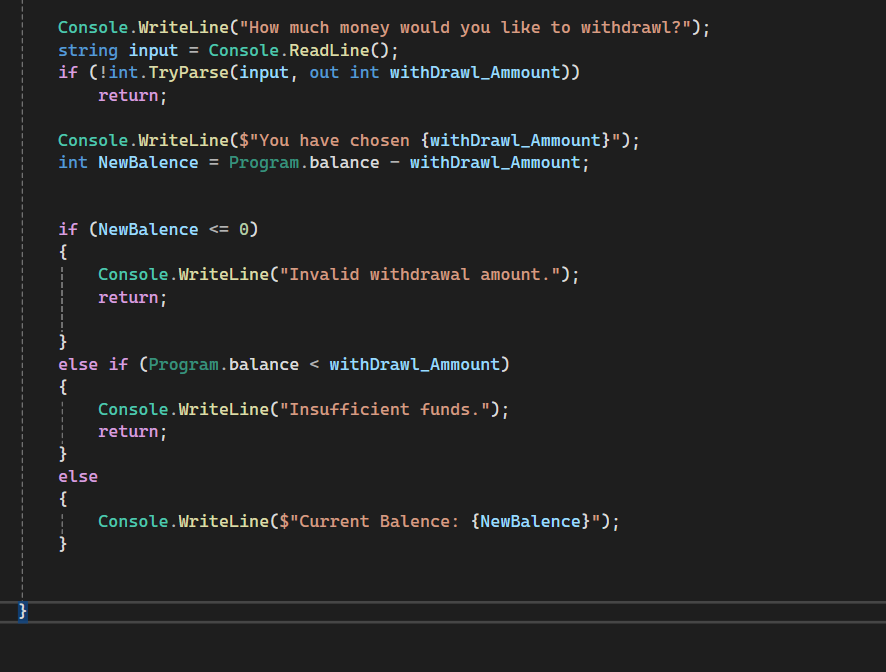
this work?
no, you are testing the same thing twice
you dont actually test for negative withdrawal
iām so hungover ima take a quick break from my pc