can't retrieve the cookies from the app
<script setup lang="ts">
import { ref } from 'vue'
import { useRouter } from 'vue-router'
import { useNuxtApp } from '#app'
import { useUserStore } from '~/stores/user'
const username = ref('')
const password = ref('')
const rememberMe = ref(false)
const errorMessage = ref('')
const userStore = useUserStore()
const router = useRouter()
const { $axios } = useNuxtApp()
const customAxios = $axios.create({
withCredentials: true,
});
const accessToken = useCookie('access_token');
const login = async () => {
userStore.isLoggedIn = true;
userStore.wait = true
try {
const response = await customAxios.post('auth/login', {
username: username.value,
password: password.value
})
console.log(response)
userStore.user = response.data
const cookie = useCookie('access_token')
if (cookie) {
console.log(cookie.value)
if (accessToken) {
console.log(accessToken)
router.push('/patients')
} else {
console.error('Access token not found in cookies')
}
}
} catch (error) {
userStore.errorType = 3
userStore.message = error.message
} finally {
setTimeout(() => {
userStore.wait = false
errorMessage.value = ''
}, 10000)
}
}
</script>
so im using nest for the beckend it is setting the access_token in cookies
but when i sign up in the front end i can see the cookies has bees set up
but i cant get the value
i get undifined
thanks in advanced
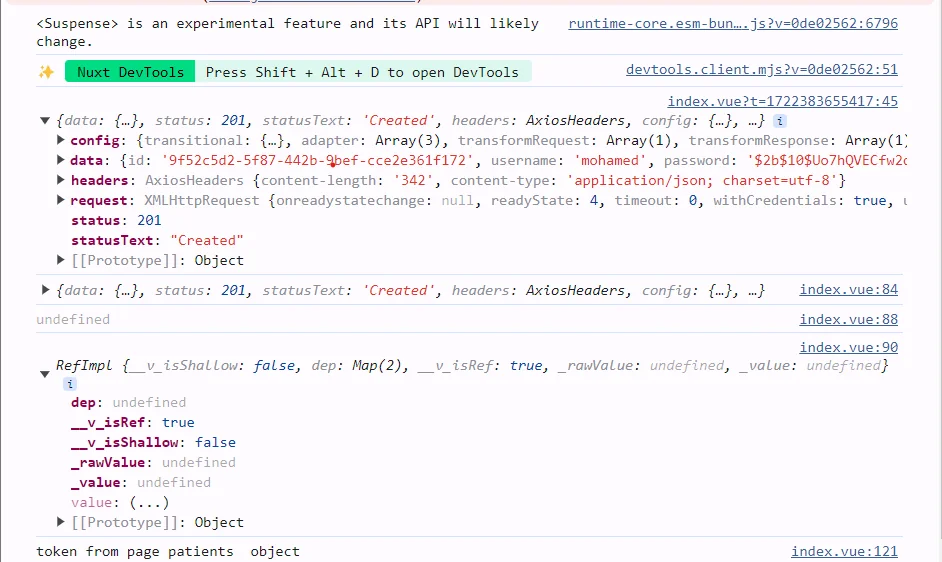
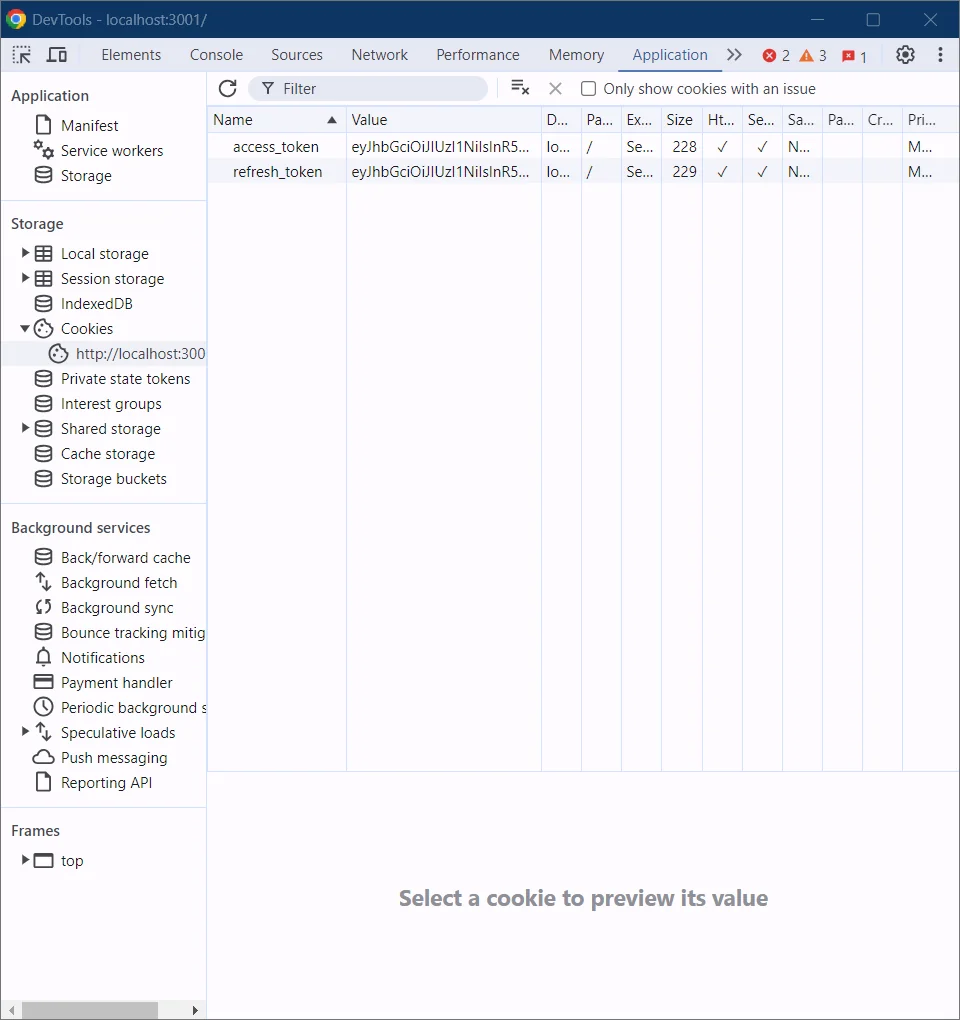
1 Reply
IIRC a client cannot read a HTTPOnly cookie