undefined on contexts that depend on each other
how can i fix this conflict?
SavedProvider
depends on useJsonData
, and JsonDataProvider
depends on useSaved
.
im getting undefined behaviour no matter the depth/order the providers are put in on the component tree14 Replies
Not really possible, you'd probably have to combine the contexts
Or introduce a higher context that receives the values and the other two read from that context, but that seems unnecessary
maybe i should implement the
saved
signal differently, but how 🤔Couldn't you move the writeable memo into the JsonDataProvider?
you mean to put the code for the memo in
JsonDataProvider
and also expose saved
and setSaved
from that context?
hmm should i just create one combined context and expose it via a object?
example
That's how I would do it
fair enough
think i will go with that then
also this will make less imports across my app which is event better tbh
wow nice one...
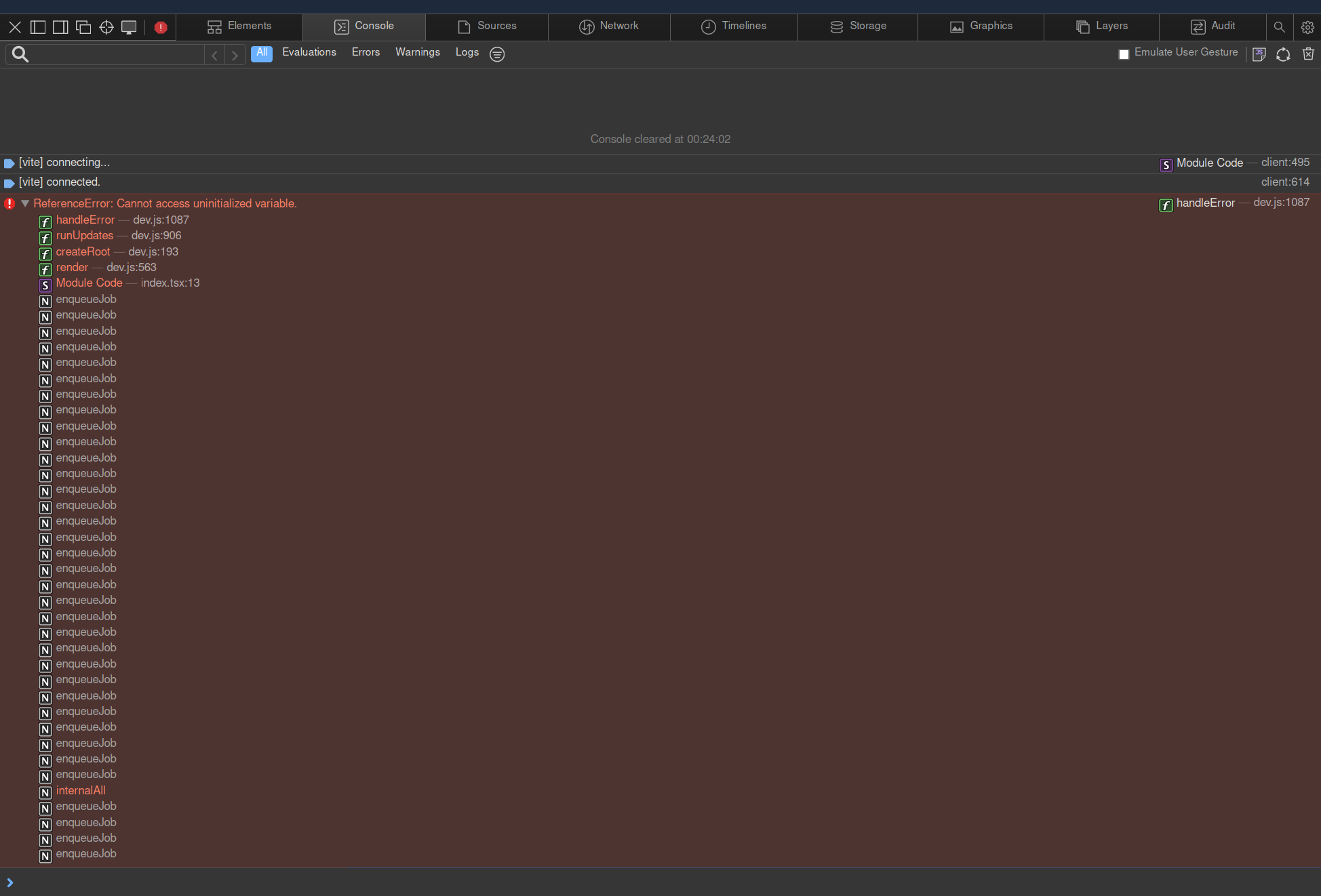
index.tsx:13 btw
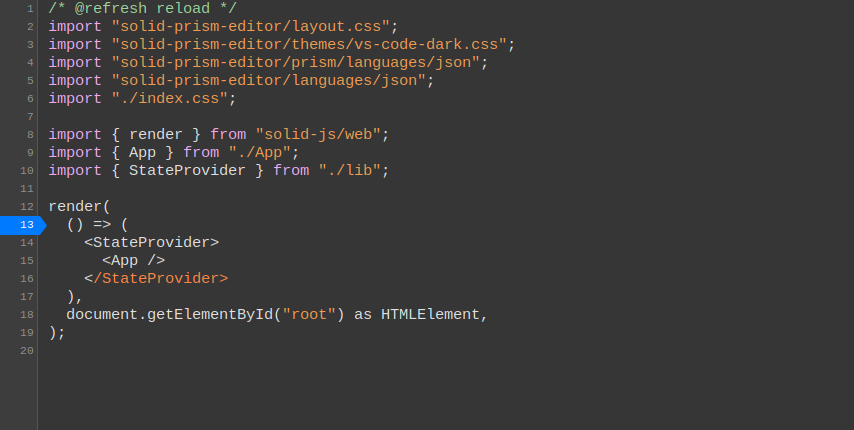
move the
createResource
up
above the writable memofixed. hmm how tf that work lmao
thank you
you were accessing jsonData in the writable memo before it was defined
idk why js/ts doesn't warn when that happens but I've run into the same thing
ahh yes i see
well, thats me finally done for the night lol. its 12:30 am💀
thanks 👍
np
yeah because it's inside a closure, we don't really know if it runs right away or next tick. it might be useful to have an eslint rule for this, before solid 2.0 lazy memo.