31 Replies
is there a way for state to persist across page reloads?
I'm trying to make a flags quiz app. it works but as soon as I reload the page. the data seems to go out of sync
Localstorage
Wrap
createStore
in makePersisted
https://primitives.solidjs.community/package/storage#makepersistedSolid Primitives
A library of high-quality primitives that extend SolidJS reactivity
it still changes when I reload the page.
when I reload the page it always goes to a certain flag
If you are using SSR you should probably switch to cookieStorage because localstorage and sessionstorage are not available on the server.
I'm having trouble rending my state as well:
I'm just trying to make a simple flag triva question but it's displaying the wrong flag when I reload the page
how is that even possible.
like the flag being displayed in
img
tag is not the same flag printed by the createEffect
I changed it a little bit. this works as expected. Now how can I just get it to stay the heck the same across page reloads? Are you persisting the signal data into storage?
nvm this is way easier than I though just doing it manually
I was trying to do it using makePersisted but I couldn't figure it out
should I just
onMount
for persisting it locally?You can, but changes made to the signal after the mount won't be persisted
so what is the alternative?
do the persisting in a
createEffect
that listens to the signalshould I add the items in
onMount
then update them in createEffect
?createEffect
will run on mount as wellso createEffect isn't running after each update to the signals. why not?
what do you mean?
createEffect
runs on mount and whenever a signal read inside of it changesif you look at the callback on my button you'll see I changed one of the signals but my createEffect is not being called.
I made sure to put log calls in each and they are only being called when I first load the page
Do you have any code you can show for your
createEffect
?
this right now works but the issue is I need to update the storage in the callback
your effect isn't listening to the signal
how do I do that?
oh I see what you're saying
You can do this
yes but now it's just persisting the initial values
Wym?
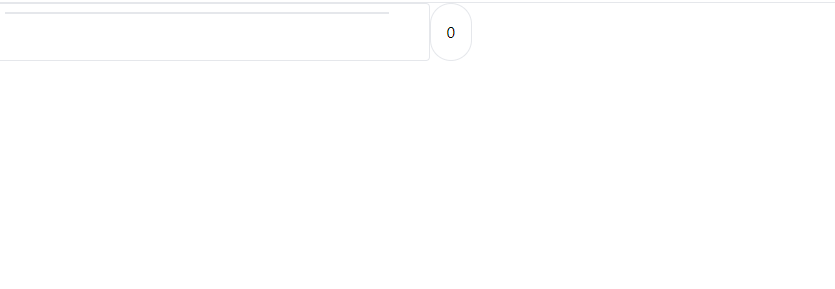
when createEffect is called it persists the initial values and so nothing is displayed
When do you want to persist it the values? The effect will call
setItem
whenever the signals changewell the logic in onMount should be called once, then for each change to the values we would persist it
the issue is createEffect is called at the beginning once as well
Actually here this will be better
Defer will stop it from running on mount
okay
thank you so much that worked
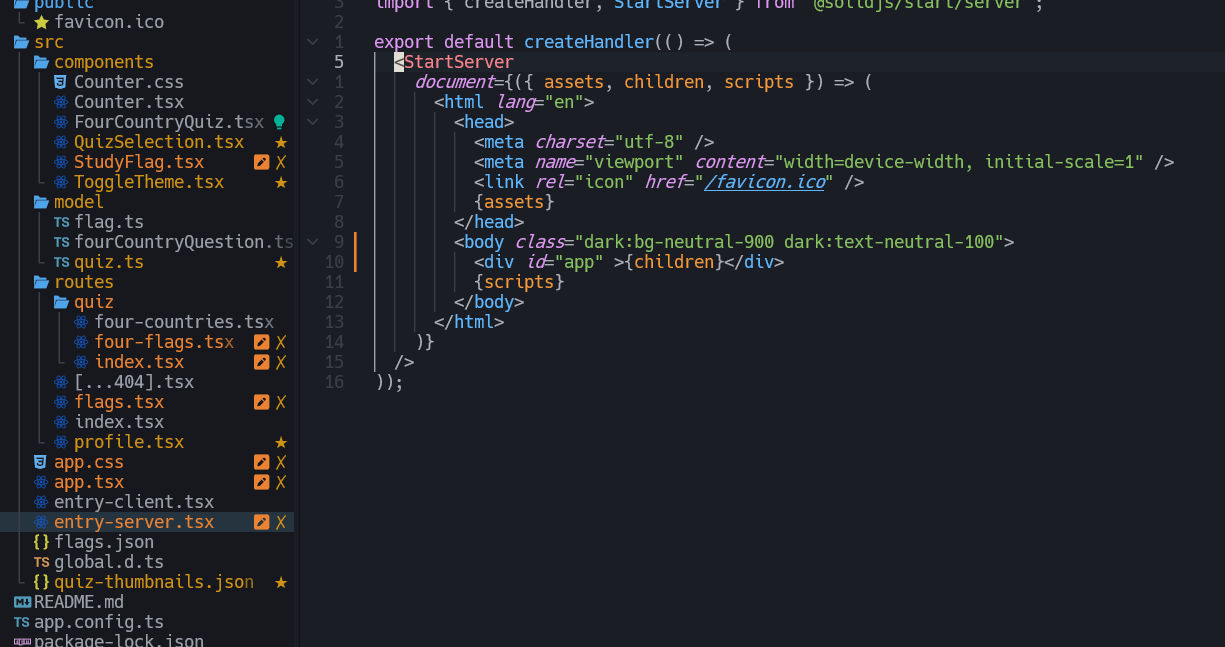
I see scripts assets and children where are scripts and assets located at?