46 Replies
seems pretty clear
whatever site you're making a request to isn't responding
the site im fetching from just returns a integer and nothing else.
no format or anything
Try to specify a useragent
Best read the docs of that API, see what it needs
lol no docs
Also, is
https://game-website/activePlayers
the actual URL you have there?is it possible for them to block my connection and therefore be getting this response type?
https://helmet-heroes-api.com/TotalOnline

Seems it can't even be pinged
Is that your game?
not mine, im just a player
That site is crazy bad lol.
It thinks I run in 4K when my monitor is just in 4K lol, I play in windowed 1/4th of the screen :kekw:
Curling works

anyway to put that into code?

HttpClient seems to work fine
Same for me
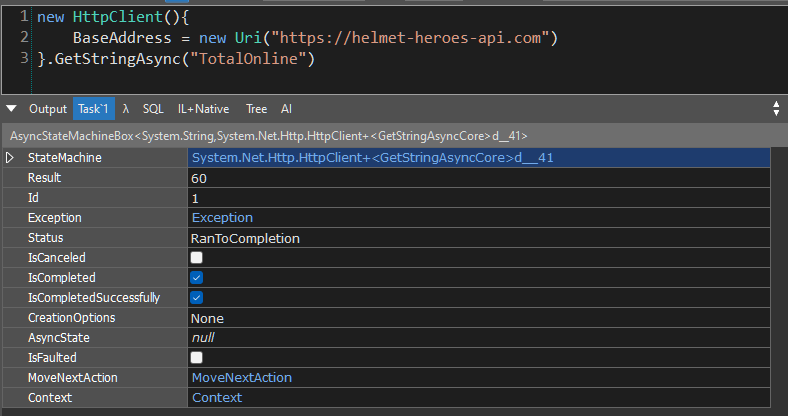
will prob use "getfromjsonasync"
Yeah, it'll make things easier for sure
i've been using "ReadAsStringAsync"
If the format is JSON, sure.
Definitely easier.
A plain number is valid JSON, so yeah
alr
im still getting this:
Your new code?
Idk what to say, it works perfectly for me
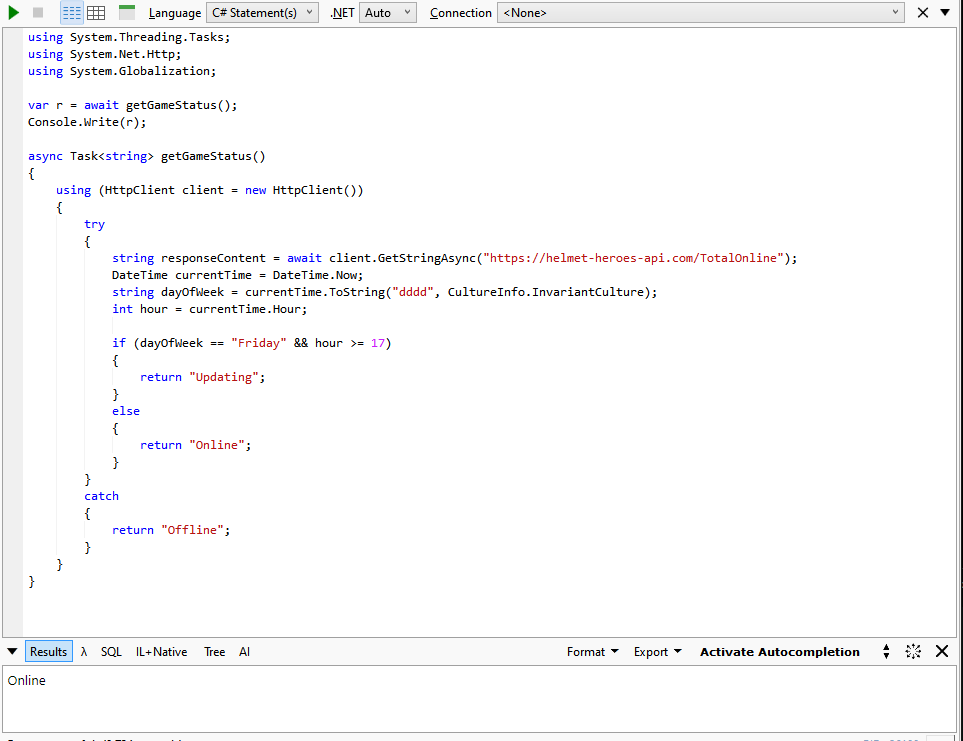
i have another call made for http client
Does that one work?
i tried putting debug statements before but they didnt even show up instead i got the error
i just relaized the error is going on the api "timeapi.io" and not the TotalOnline
is it possible for it to be blocking it?
Ah, damn, true lol

know any libs that has a clock that shows time for UTC and PH?
NodaTime should be able to easily convert time between timezones
https://nodatime.org/
NodaTime 3.1.11
Noda Time is a date and time API acting as an alternative to the built-in DateTime/DateTimeOffset etc types in .NET.
It's even in the sample code on their website lmao
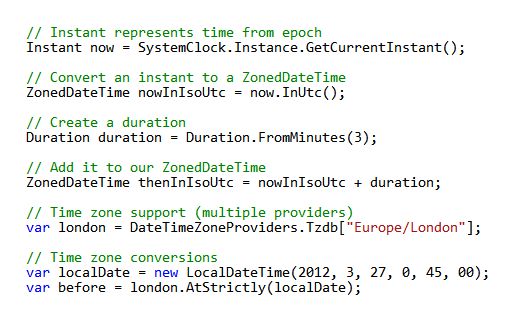
Yeah
alr let me rewrite my other .cs file and see if it still causes an issue
now its returning this:
still trying to figure out why the text isnt showing up

should be like this

Ah, it's, like, WPF or something?
yes
Async code with UI frameworks can be... iffy
any suggestions?
Chances are you have an
async void
or something somewhere that's causing this issue
'Fraid not, I don't have much experience with making UI appsrip
i solved the issue i forgot to bind it in the
file.xaml.cs