Sendgrid Error
const info = await emailSender.send({
from: {
name: name,
email: "[email protected]",
},
to: "[email protected]",
subject: subject,
text:
Some text
,
html: Some text
,
});
I am trying to send mail, I authenticate my Sendgrid account, added DNS configuration and everything and getting this error:
Access to XMLHttpRequest at 'https://api.sendgrid.com/v3/mail/send' from origin 'https://requestphotoedit.com' has been blocked by CORS policy: Response to preflight request doesn't pass access control check: The 'Access-Control-Allow-Origin' header has a value 'https://sendgrid.api-docs.io' that is not equal to the supplied origin.
When I check stackoverflow, it says I cannot send mail over Javascript code? https://stackoverflow.com/questions/54797626/react-unable-to-send-email-with-sendgrid
Help me plzStack Overflow
React unable to send email with SendGrid
I am using react to send emails:
const sgMail = require('@sendgrid/mail');
sgMail.setApiKey(process.env.REACT_APP_SENDGRID);
const msg = {
to: '[email protected]',
from: '[email protected]',
24 Replies
Hi @skyblade2954. I'm kapa.ai, your friendly support bot. I will try to answer your question by searching through the knowledge base. :wave:
----------------------
The error you're encountering is related to Cross-Origin Resource Sharing (CORS) policy. This is a security measure implemented by browsers to restrict how resources are shared between different origins (domains, protocols, or ports).
In your case, it seems like you're trying to send an email directly from your client-side JavaScript code using SendGrid's API. This is generally not recommended due to security reasons, as it would expose your SendGrid API key on the client side.
Instead, you should send the email from your server. In the context of Wasp, you can use the
emailSender
API provided by Wasp. Here is an example of how to use it:
(Source: Wasp Docs (https://wasp-lang.dev/docs/advanced/email#javascript-api))
If you're still encountering issues, it might be due to the configuration of your SendGrid account or the way you're making the API call. In that case, you might want to check the official SendGrid documentation or their support for more specific guidance.
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------
Sorry @skyblade2954, I seem to be having a bit of trouble. Don't worry though, I've pinged my support and they will try to fix me up! :technologist:
Perhaps try again in 15mins?I did what it is in the tutorial.
However it gives this error... What's wrong?
import { emailSender } from 'wasp/server/email';
export const sendEmail = async (name, email, subject, phone, body) => {
try {
const info = await emailSender.send({
from: {
name: name,
email: email,
},
to: '[email protected]',
subject: subject,
text:
Phone: ${phone}\n\nMessage: ${body}
,
html: <p>Phone: ${phone}</p><p>Message: ${body}</p>
,
});
return { success: true, info };
} catch (error) {
return { success: false, error };
}
};
@kapa.aiLet me check the knowledge base for you. One moment! :books:
Sorry @skyblade2954, I seem to be having a bit of trouble. Don't worry though, I've pinged my support and they will try to fix me up! :technologist:
Perhaps try again in 15mins?
@kapa.ai
Let me check the knowledge base for you. One moment! :books:
Sorry @skyblade2954, I seem to be having a bit of trouble. Don't worry though, I've pinged my support and they will try to fix me up! :technologist:
Perhaps try again in 15mins?
@Wasp Expert
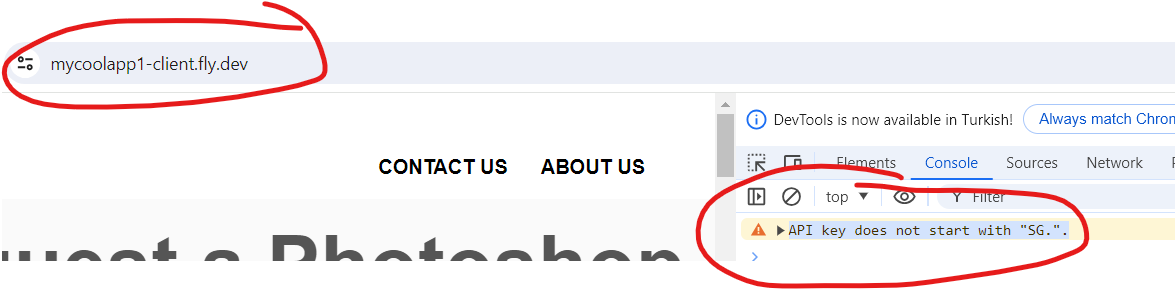
@skyblade2954 :
1. You should be sending those emails from your server code (actions or queries), not from your client code (react). It seems to me you already fixed taht.
2. I don't see the error you get, you pasted code above but not the error with it.
3. The warning/error in the console says that API key for sendgrid is not correct. It should start with "SG.", but yours doesn't. Probably because it is empty or incorrect / set to dummy value. During local develpment, you will want to set your key in .env.server . In production, you will want to set it as env var / secret on your cloud, for the server.
1. "You should be sending those emails from your server code (actions or queries), not from your client code (react). It seems to me you already fixed taht."
I am not sure where I am sending the mails. If it is in client or server. I created actions folder under src folder, and created a email.js file. I put my code under that folder:
export const sendEmail = async (name, email, subject, phone, body) => {
try {
const info = await emailSender.send({
from: {
name: name,
email: email,
},
to: '[email protected]',
subject: subject,
text: Phone: ${phone}\n\nMessage: ${body},
html: <p>Phone: ${phone}</p><p>Message: ${body}</p>,
});
return { success: true, info };
} catch (error) {
return { success: false, error };
}
};
I am calling this function from another file under src. So probably there is a chance that I am calling email send function from client side.
2. "I don't see the error you get, you pasted code above but not the error with it."
It is the same problem as in the first question.
3. Why do I get a warning in the client side? Why does client side trying to find SendGrid API key? It shouldn't be required from Client side. @martinsos
1. Thanks for more info -> yeah you should make sure you call that sendEmail function from server code, so from queries / actions.
3. It shouldn't, you are right, which indicates you are indeed by accident calling email sending from the client side! So fixing (1) should take care of this.
I think I should be using actions, I just started with the advance tutorial. So didn't understood that I need to add an action.
Yes exactly, it should be an action! A brainfart from my side regarding mentioning queries: it should be an action indeed.
I probably passed the first error, I am using "wasp deploy fly deploy" to deploy my project to server and test it. Do you think I can test it locally? Or with out building everytime?
Because "wasp deploy fly deploy" takes a lot of time.
Yes of courseyou can test locally! If you want to do it proper, you should develop and test it locally, then once you are ready for deploying test it in staging environment (kind of like production but for testing), and then finally push to production. You can skip the staging part if it is a smaller project in early stag and you want to move faster and are not too worried about having production potentially broken for a bit.
How? Because each time I run "wasp deploy fly deploy". How can I do it?
Don’t we all mind it broken for a bit?
bruh. How can I deploy code without building every part of it? Because each time I run "wasp deploy fly deploy" it takes a lot of time.
Deployment == building apriori, you cannot deploy without building
So there is no way to test sendgrid mail system with out deploying? Or can I test mail system by activly sending mails in my localhost?
Well that is not what I said and that was not your question.
You can test things locally
If you want to test sendgrid locally, have a look at their documentation, I don’t know how to personally
Ok I could be able to test it locally, everything is fine now.
Generaly yes, but in practice it can vary, for example for usemage.ai we don't have a staging environemnt, because it is not overly complex and there aren't many reasons why it would break in production. And even if it does, we can revert it if needed and we don't loose much if it is broken for a short time. So really depends on the project, how mission critical is it for the users, what are their expectations, and how costly is it for you to have it broken for a bit vs investing time into having a staging environment.
Yes of course 🙂 I was just joking, I think dudes at CrowdStrike would appreciate the joke
Hah yes indeed 😄