Is there a more proper way to set value across components?
I've tried an experiment of setting value across components.
The codes in the shot screen works, but it should be a better way to do this right?
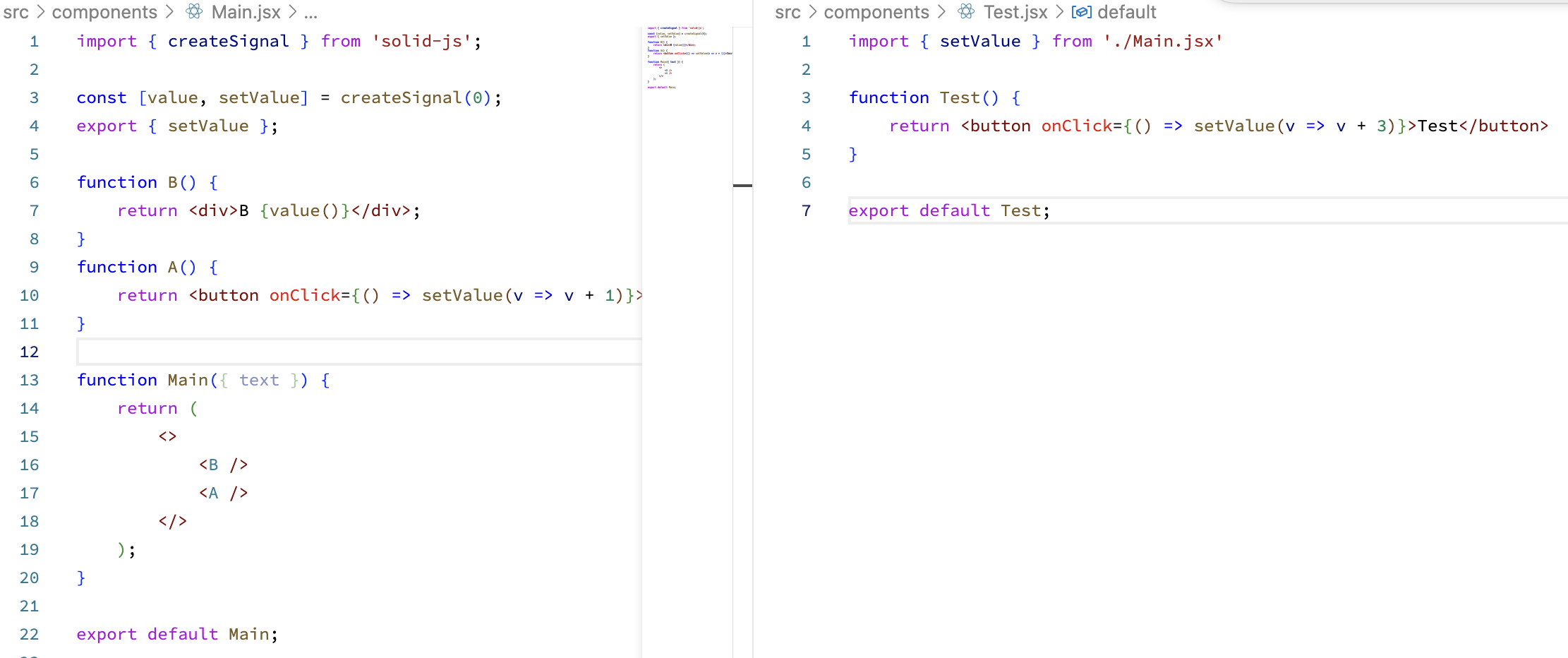
5 Replies
No, using the setter in multiple locations is the way to go. If you want, you can encapsulate the functionality in a context, but you can also keep it global.
Thank you
I would be extremely reluctant to hand out a raw setter like that.
The “owner ” of reactive state should constrain the possible ways in which that state can be modified. The definition of those mutators should be collocated with the state itself and exported (if absolutely necessary) instead of the setter.
https://playground.solidjs.com/anonymous/e26301b5-e5dc-4d69-9304-10022a0b11e1
3. Read/Write segregation.
Solid Playground
Quickly discover what the solid compiler will generate from your JSX template
SolidJS
Solid is a purely reactive library. It was designed from the ground up with a reactive core. It's influenced by reactive principles developed by previous libraries.
Under most circumstances I would consider this a “code smell”:
i.e. needing access to both the accessor and mutators at the same time.
Compare to:
https://playground.solidjs.com/anonymous/40c07288-1536-4f99-9f6f-283506f87667
Solid Playground
Quickly discover what the solid compiler will generate from your JSX template
@peerreynders thank you for the advice