✅ EF Core Relationship
I have 3 different models call Owner, Products and Warranty.
The relationship between them is that Products can be created independently, so when a user purchase a product Owner table will have foreign key ProductsId inside it. During product purchase a new Warranty is created so OwnerId will be a foreign key in Warranty table.
How do I declare this in model and the relationship at ApplicationDbContext? I'm having trouble due to wrongly created model and unable to submit the form from my Angular application.
Model become more complex after implementing navigation property which now makes me unable to create a Owner
26 Replies
?
So owner should have
List<Product>
and List<Warranty>
Product should have List<Owner>
and List<Warranty>
Warranty should have Owner
and Product
Yes but the problem with that is during my API Post call, it will complain the list of Owner cannot be empty inside Products.
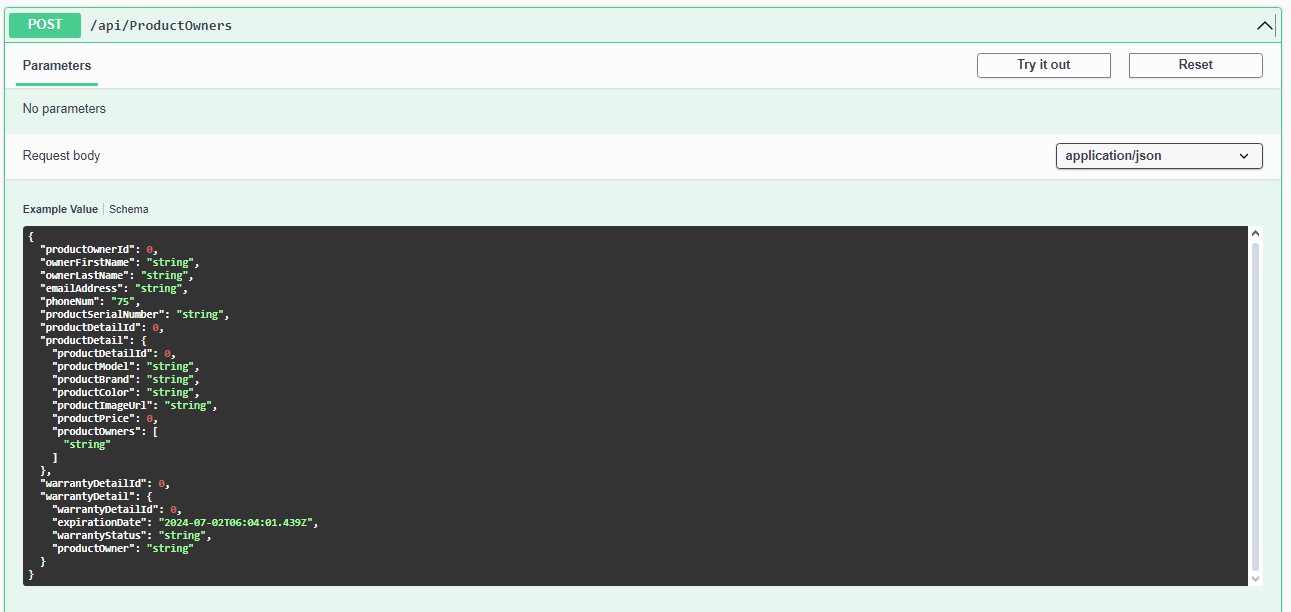
What's doing the validation?
I've handle in my controller to create a new unique serial number and create a new Warranty during the POST API call
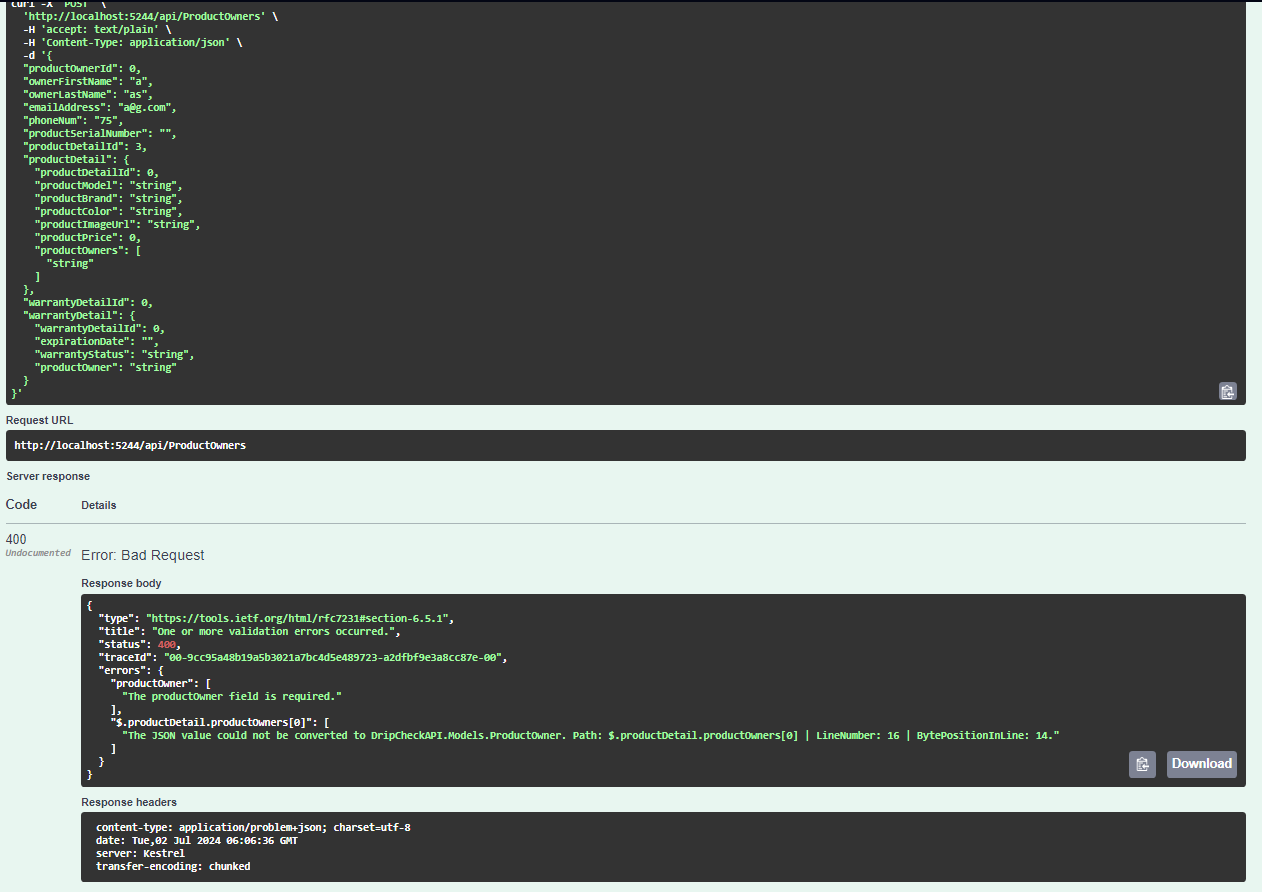

I dont quite get it sorry. but I paste the response call that I got the error from. In my model I've created a list of Owners. but during Post API if the owner is not created yet how would I get the details to put in the list?
I mean code
What in code is validating that the list must not be empty?
This productOwner inside Warranty model. Sorry not the list ones
So it's not the product that's an issue?
It's warranty?
Yes, when a owner is created automatically in Warranty a new record is created in controller. But since this is related to navigation property I declared for the one-to-one relationship
I'm having trouble understanding
You mean when a warranty is created, you also create an owner?
When an owner is created a warranty then will be created.
My ERD
I think if i only implement the foreign-key there will be no issue, but now with navigation property it got me stuck. First time dealing with navigation property
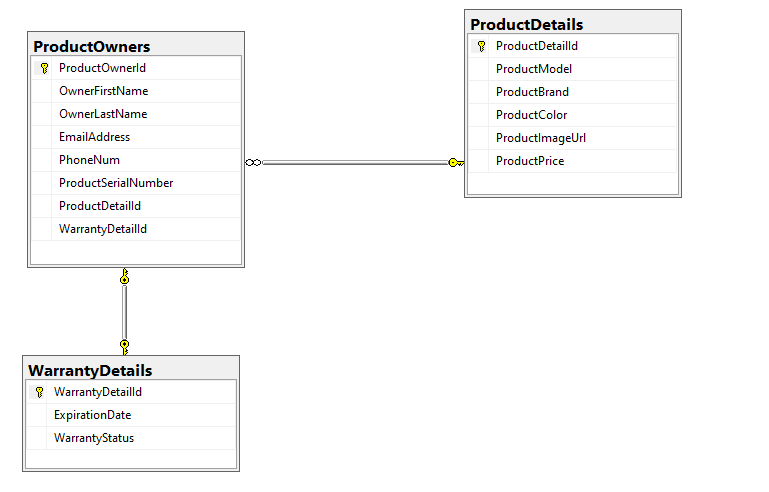
So an owner cannot exist without warranty?
In case this helps :/ or should I post the code else where
Yes, because when owner purchase a product it should come with a warranty
I'd start by using a DTO for the API instead of the database entity
Since it's complaining that what you send to the API is incomplete... just use a DTO without the things that are unnecessary
Correct me if I'm wrong so if a use DTO , my API call request body can be modified? I was in the assumption that all the columns in a table/entity should be made available in JSON Request body during an API call
Only the things you want to send should be available in the request
You wouldn't want the user to be able to set their own password hash
Or their ban duration
Understood ... :NotedHmm: so it should be a good practice to always use DTO so we can modify the API request body..
For example in my db i have an entity with columns of brand/model/color.. assuming the brand is created by default i should not showcase it in my request body, I create a DTO only for model/color ...
Correct? haha
It's always a good idea to use DTOs period
API should receive and return DTOs
Database models should never leave the app's boundary
Ok got it, will update here if I able to restructure it successfully. Thanks for the help :feelscomfyman:
@ZZZZZZZZZZZZZZZZZZZZZZZZZ Thankss man, restructure the code and created DTO. Now time to fix the rest of the methods with DTO too :sadcat:
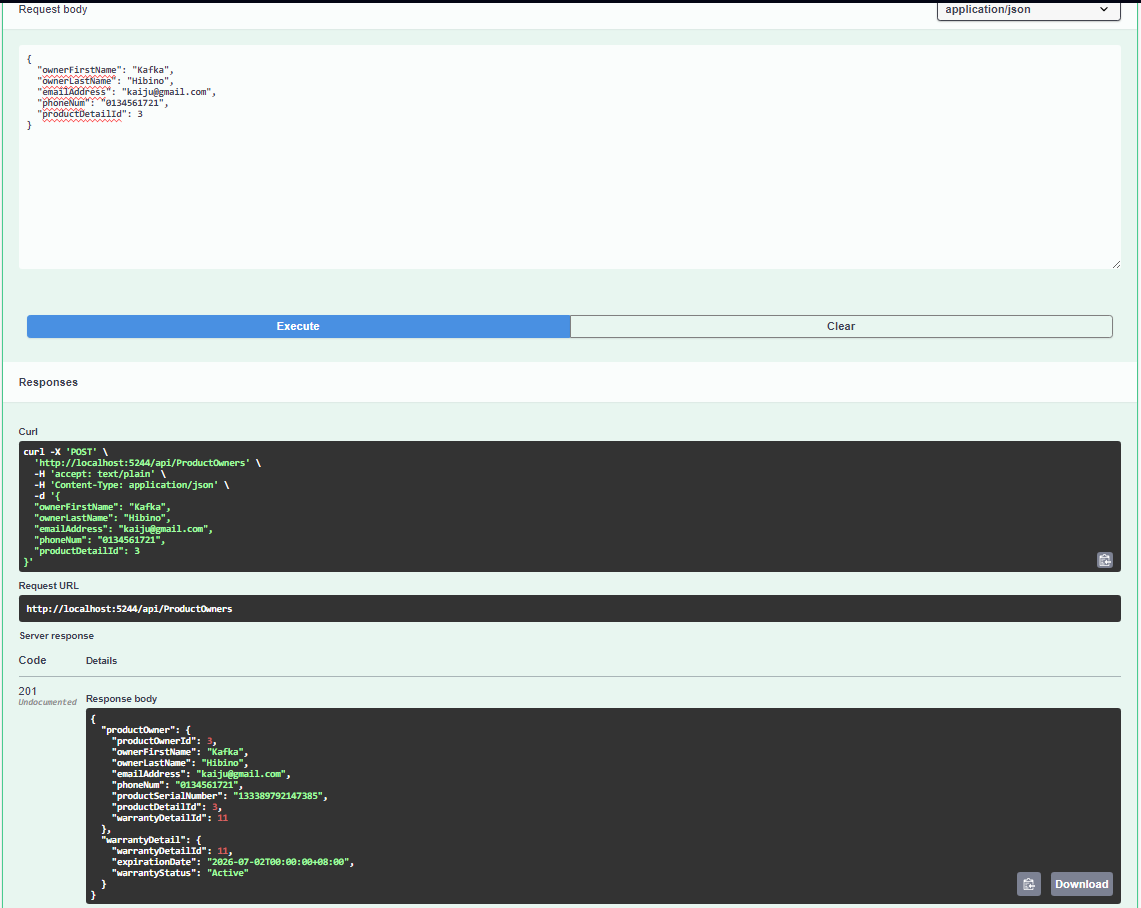
Nice
Anime reference cause saw your Youtube channel haha. Have a great day man. Arigato!
Anytime :Ok:
Unknown User•5mo ago
Message Not Public
Sign In & Join Server To View
If you have no further questions, please use /close to mark the forum thread as answered