$inferSelect and $inferInsert doesn't return table types
Hey There, Im Using Koa.js having issues with inserting as the types arent correctly referenced from the table.
tsconfig.json:
{
"compilerOptions": {
"target": "ESNext",
"module": "commonjs",
"lib": ["ESNext"],
"outDir": "dist",
"rootDir": "src",
"noImplicitAny": false,
"experimentalDecorators": true,
"emitDecoratorMetadata": true,
"esModuleInterop": true,
"skipLibCheck": false,
"forceConsistentCasingInFileNames": true,
"moduleResolution": "node",
"allowSyntheticDefaultImports": true,
"strict": true
},
"include": ["src/**/*.ts", ".drizzle.config.ts"],
"exclude": ["node_modules", "dist"]
}
drizzle.config.ts:
import { defineConfig } from "drizzle-kit";
export default defineConfig({
dialect: "postgresql",
schema: "./src/database/schema",
out: "./drizzle",
dbCredentials: {
url: process.env.DATABASE_URL as unknown as string,
},
});
Drizzle instantiation:
import postgres from "postgres";
import { PostgresJsDatabase, drizzle } from "drizzle-orm/postgres-js";
import dotenv from "dotenv";
dotenv.config();
import * as schema from "./schema";
declare global {
var database: PostgresJsDatabase<typeof schema> | undefined;
}
let database: PostgresJsDatabase<typeof schema>;
const DATABASE_URL = process.env.DATABASE_URL as unknown as string;
if (process.env.NODE_ENV === "production") {
database = drizzle(postgres(DATABASE_URL), { schema });
} else {
if (!global.database) {
global.database = drizzle(postgres(DATABASE_URL), {
schema,
logger: true,
});
}
database = global.database;
}
export default database;
Here is my table definition:
export const agencies = pgTable("agencies", {
id: serial("id"),
// id: varchar("id", { length: 32 })
// .$defaultFn(() => generateId("user"))
// .primaryKey(),
name: text("name").notNull(),
createdAt: timestamp("created_at").defaultNow().notNull(),
updatedAt: timestamp("updated_at").default(sql
current_timestamp),
deletedAt: timestamp("deleted_at"),
});
export type Agency = typeof agencies.$inferSelect;
export type NewAgency = typeof agencies.$inferInsert;
Agency type outputs to:
type Agency = any
NewAgency type outputs to:
type NewAgency = {
name: any;
id: any;
createdAt: any;
updatedAt: any;
deletedAt: any;
}
here is the code in my persistency layer:
export async function createNewAgency(values: NewAgency) {
console.log(values);
try {
return await database.insert(agencies).values({
name: values.name,
});
} catch (error) {
console.log(error);
}
}
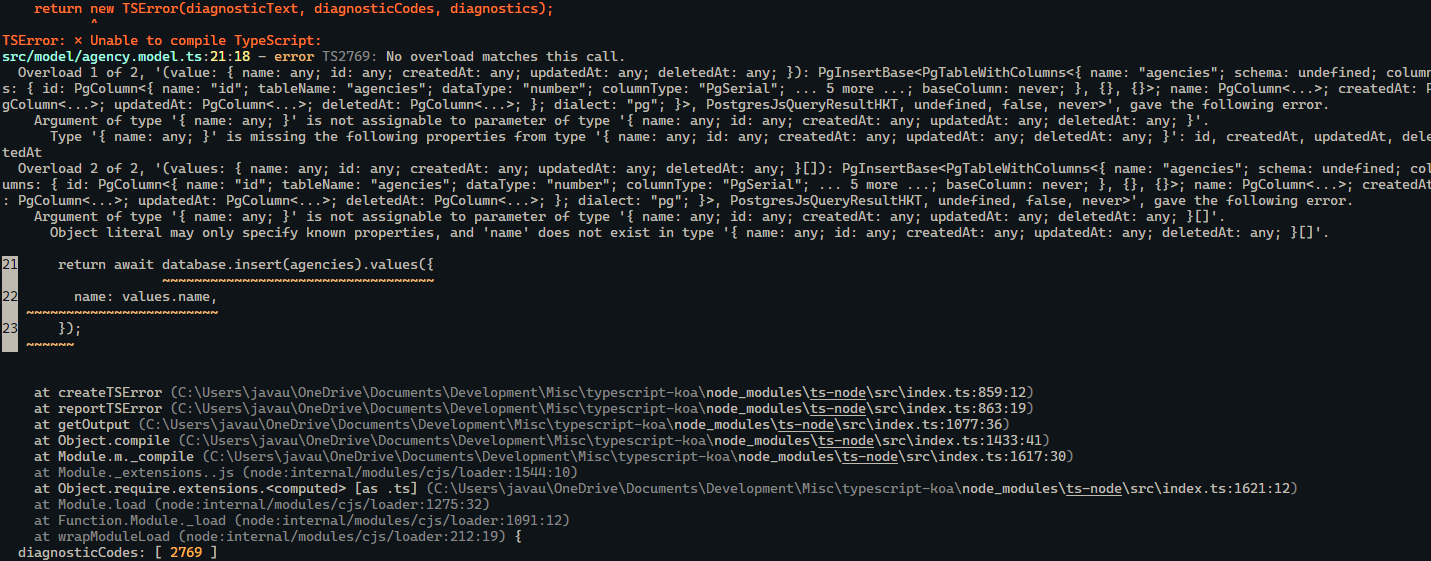
1 Reply
Fixed: Two things to check for
Make sure your typescript version is updated to latestt if possible
also, if there is a silent type error within the schema definition then it will not give the correct type
the culprit:
parentId: varchar("parent_id", { length: 30 }).references(
() => agencies.id,
{
onDelete: "cascade",
}
),
changed to: parentId: varchar("parent_id", { length: 30 }).references(
(): any => agencies.id,
{
onDelete: "cascade",
}
),