How to make full static generation
Hello, I'm trying to build a blog site using a JamStack like architecture.
I want to deploy it to Cloudflare Pages as a completely static site, including the API responses.
I wrote app.config.ts as follows and used the fetch method to get data from the API.
However, when I build and run the site, API requests using the values from process.env are made during the page load.
I want to save the API responses at build time, similar to the full static generation in Nuxt.js or Next.js, so that no API requests are needed after deployment.
How can I achieve this?
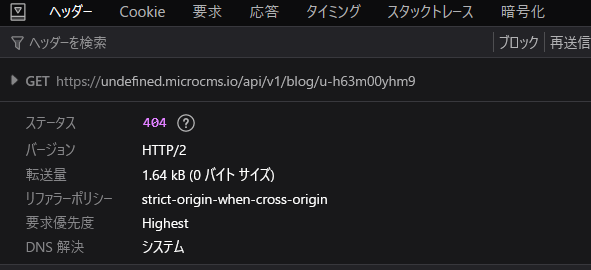
6 Replies
How do you call the fetcher function? Try wrap the function call in createAsync or use solid router's load function
Haven't tried SSG yet but i think the intended approach here is to use solidjs provided method
But if you are asking how to predefined "id", then idk
I forgot to mention the function call and the params. I am calling the fetch function as shown in the code below.
I use FileRoutes and get
I use FileRoutes and get
id
from path params.
By crawlLinks: true,
option in app.config.ts, those pages seems prerendered properly.
But I access these page the first time, I get the aforementioned error
is the post fully rendered/hydrated on the generated files? if yes, then i think the last thing you need is to use isServer to prevent the fetch from running in the client
idk the implication though, will solid runtime purge the initially rendered html because post resource will be empty on client...
trial and error haha
try this https://docs.solidjs.com/reference/rendering/is-server
The generated HTML file contains the complete content of the CMS...
However the client will still try to perform a fetch. (I found that reloading the page shows the content ....)
Thank you so much! I will try it !
if its not working as expected, then try to use route load function instead https://docs.solidjs.com/solid-router/reference/load-functions/load
Logically speaking, load function should be the closest thing you want since it will make page rendering idempotent and simple, just like in nextjs, remix, or gatsby. But again, i haven't tried SSG yet, so yeah
correction: load function is not available when using filerouter 😦
Of course they are: https://docs.solidjs.com/solid-start/building-your-application/data-loading
In route.tsx: