What's wrong with my LINQ expression?
Hi! I'm trying to get data using LINQ expressions below. I use Sqlite
But I'm getting exception
32 Replies
Try splitting your where?
into
It should work from what I can tell, maybe a quirk of SQLite
The closure includes a
DateTimeOffset
variable, whose operator >
the expression compiler can likely not translate to sql , it's a limitation of the system.Stack Overflow
How to Use Dates in Where Clause in EF Core?
I need to filter my queries by dates but I don't care in this case about time portion of it that is stored in SQL Database.
I first tried to something like
var now = DateTime.Now.Date;
Where(x =&...
They're using a
DateTime
instance, so maybe try doing that instead of DateTimeOffset
Notice that database-bound operations are limited in this manner, but process-bound (enumerated) operations are not. I.e. you can use arbitrary linq operations after you enumerate your query out of the database into memory via, e.g., ToListAsync()
.You can implement custom functions as per this article:
https://learn.microsoft.com/en-us/ef/core/querying/user-defined-function-mapping
User-defined function mapping - EF Core
Mapping user-defined functions to database functions
sqlite doesnt have a date and time types
if you want to compare based on that you likely need to add a converter
there's a few options
DateTimeOffsetToBinaryConverter
or something custom... but probably no need for custom
its just sqlite limitation. it would work fine in e.g. postgres
and no need for this
add a converter and you can keep those operations db side
How will the conversion enable using the
>
operator though?exactly it will
SQLite Database Provider - Limitations - EF Core
Limitations of the Entity Framework Core SQLite database provider as compared to other providers
datetime solution is... 😬 i would ignore datetime ever exists in the language
its a can of worms
I mean the convert to utc via converter seems fine to me
sure
but rather keep DTO
$datetimelie
please please please get into the habit of using DateTimeOffset instead of DateTime in your code everywhere you possibly can. DateTimeOffset contains the UTC offset with the data so is lossless. DateTime is very very weird in how it stores data. DateTime only knows UTC and "local" time. But your local time zone can change! Laptops easily move across state lines, even while an app is currently running. (So you are in California running an app, put some DateTime instance in memory, hop on a plane to New York, then resume your app 3 time zones ahead. What on earth will be contained within the DateTime instance?) But wait, this was a lie. DateTime actually has 3 ways it keeps track of time: UTC, local (which can change while an app is running), and unspecified (unknown, essentially). (Unknown = I don't know if it's UTC or local, the app will figure it out later and call ToUniversal or ToLocal before calling ToString or any comparison routine.) But wait, this was a lie. DateTime actually has a secret fourth way of storing data that's not exposed in the standard API surface! It's truly an abomination of a type. All of this nonsense is irrelevant if we just pretend DateTime doesn't exist and we instead use DateTimeOffset everywhere.- GrabYourPitchforks (start: https://discord.com/channels/143867839282020352/143867839282020352/988353756108312607)
I tried to fix it with DateTimeOffsetToBinaryConverter (onModelCreating) but it doesn't work. I can switch to postgresql, there's no problem
did you create migration after
sqlite is perfectly fine.. if it suits your needs
yes, I added code:
to the
protected override void OnModelCreating(ModelBuilder builder)
and created a migration and updated databaseI think it's correct
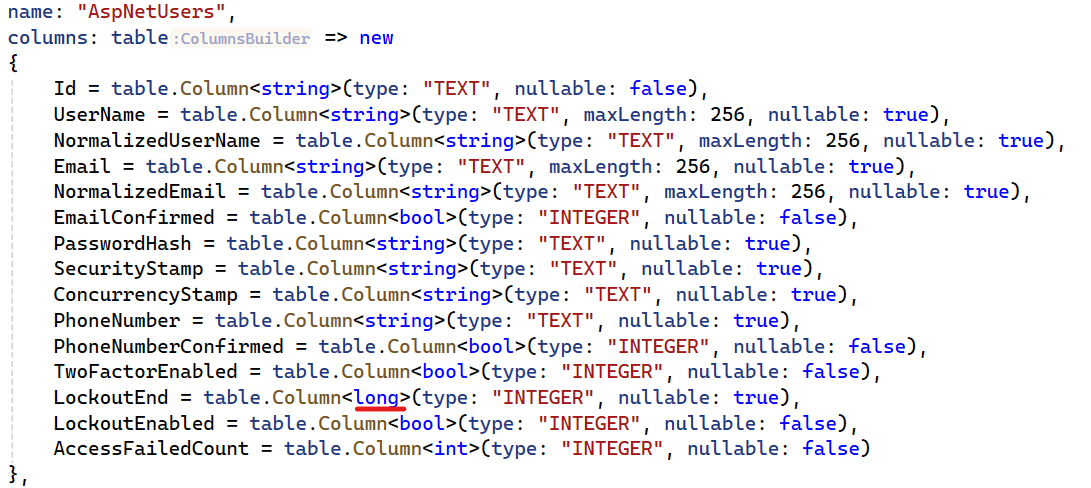
actually
1sec
do it like here https://learn.microsoft.com/en-us/ef/core/modeling/value-conversions?tabs=data-annotations#bulk-configuring-a-value-converter
configureconventions
should I add it instead of previous code?
yea
applied migrations?
yes
try DateTimeOffset.UtcNow instead of .Now just to be sure
actually
show the code if you changed something
it should be like this except UtcNow instead of Now
dont do DateTimeOffset.UtcNow inside .where
it cant translate to sql
that's was the problem, I changed
.Where(u => u.LockoutEnd != null && u.LockoutEnd > DateTimeOffset.UtcNow)
to var utcNow = DateTimeOffset.UtcNow
and .Where(u => u.LockoutEnd != null && u.LockoutEnd > utcNow)
and it works finecool
thank you!
np
also $close
If you have no further questions, please use /close to mark the forum thread as answered
i'll try to close it again later
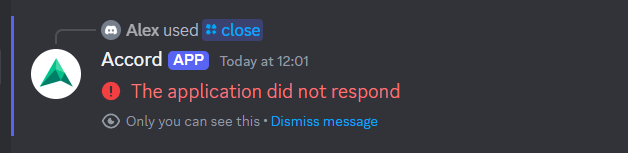
😆 ok then bot broke