✅ Variable calling from other classes
I've got a question, so imagine I've got 3 classes, and I was trying to make a method to show the information from the 1st class(Info.cs) to 2nd class(Menu.cs) through a 3rd class(MiddleClass.cs), would that be somehow possible?
43 Replies
Sure
The two classes can expose a public property
And you can access it from their instances
Angius
REPL Result: Success
Console Output
Compile: 526.359ms | Execution: 43.836ms | React with ❌ to remove this embed.
I think I might have explained wrong here
so my issue is
I have 2 infos classes (examples only) and let's say you have alot of objects on info1.cs, and in info2.cs u got the information about those objetcs
could you search on info1.cs and get the info2.cs connected to it through a method?
Sure, you can pass instances of one class to a method
And do whatever with them
If you have a lot of objects, I assume it's a list or an array or some other collection
In that case yes, you can search for the specific instance
LINQ
.Where()
and/or .FirstOrDefault()
will be useful herewould it be a bother if I sent the example where I'm having this issue?
Sure, go ahead
it's in another irl language, but it showed to be no bother to another of the helpers here
ok
GitHub
Issue/TrabalhoFinal_23-24/TrabalhoFinal_23-24 at main · JoValadares...
Contribute to JoValadares/Issue development by creating an account on GitHub.
so I'm trying to get this method to show the localização of it's creator (NoSensor's location) and it's estadoAtivo (true or false, also in NoSensor) through Sensor.cs Id
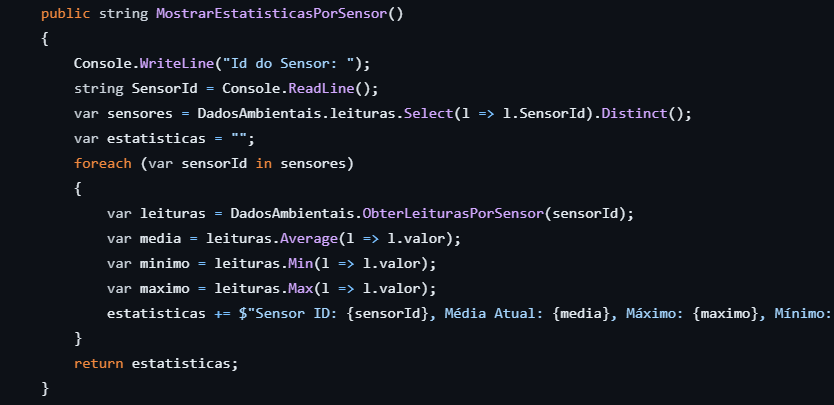
SistemaMonitorizacaoAmbiental
has the noSensores
property that contains multiple NoSensor
s
NoSensor
has sensores
, which is a list of Sensor
s
That has estatoAdivo
So you can loop over noSensores
, then over sensores
Or use LINQa foreach u say?
I really don't know what LINQ is
For example, yes
but then how'd do the foreach?
what would I search in Nosensor
Sensores
basically
so right now I have it like this
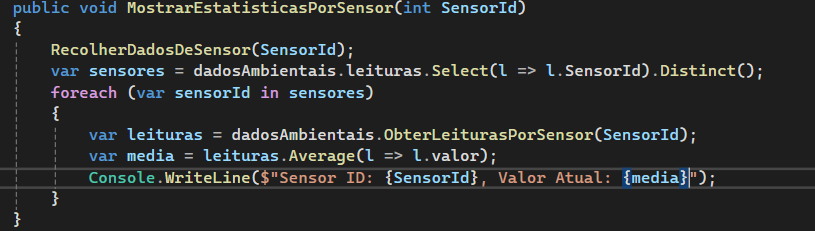
and right now it's showing the id and value of the sensor I just searched
how would I add a foreach here that'd make the localização and estadoAtivo of the NoSensor that this Sensor belongs to appear on the aftermath
Assuming
sensorId
is actually an instance of Sensor
, and not its ID like it says
Get that data from therealso for some reason when using the foreach here, instead of showing it one time, it'd show the same thing thrice
and when I took it off it worked out
Well, if there are three
sensores
...C# mixed in with a language you still have issues with can be such a hard achievement
I'm sorry if I don't get it but what does that mean?
If there are three elements in the
sensores
list, the loop will run three times, so it will print to console three timesI see, so the problem was that the console write line was inside the foreach
not that the foreach itself was wrong
The problem was you expected to get a single sensor, seemingly
But you got three
At no point did you say "I want just one sensor"
it was showing only one sensor, but repeating the same one 3 times
I've been trying the foreach and still can't get a hold of how to do it in a way that it'd show these variables correspondent to the Id I'm searching for
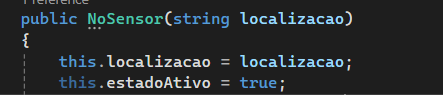
Don't select just the ID, get the entire sensor. Then, get those properties from that sensor
Assuming
dadosAmbientais.leituras
is a list of sensors?that's where they are read from if that's what you're asking yea
would this not be enough?

Is it?
apparently not, since I can't seem to reach even estadoAtivo, which is a Sensor variable
Does this not work, from the
MonstrarEstaticadasPorSensor()
method?
It should be able to list all sensors from all nosensorsthe thing is
that method
should be able to go to a nosensor, and check if the Id that the user put in exists, and then show the information
and for now it's working
it's showing back the id and the ValorAtual of the sensor the user asks for
but I want it to show more than just the id and valor
Then get more
that's the issue, how do I call them to the method?
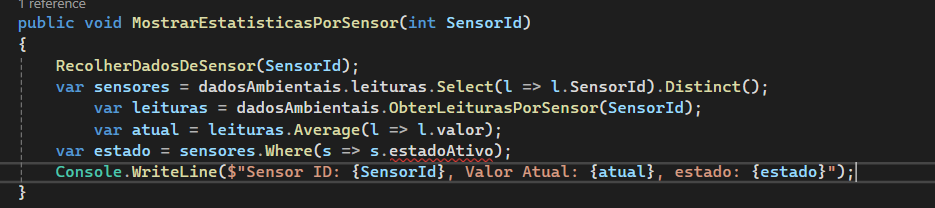
Get the nosensor the user requests
From that nosensor get all sensors
Right now your sensores is a list of just the IDs
yea but I'm not asking the user for nosensores, I'm asking the user for the sensor
But sensors are inside of nosensors
yes
This class has a list of nosensors
Each nosensor has a list of sensors
yes
So you will need to get all the sensors from all the nosensors
And get the one you need
Something like this
Or with LINQ,
I can definitely see it working
I just can't implement it
never mind I got it working