Import action make field nullable does not work
I want to store fields in db even if they are not valid or empty as null, but I can't get this to work.
I have tried to cast the field to null but it always says "must be valid date".
Help is much appreciated! 🙂
Solution:Jump to solution
Thanks solved it like so
```
protected function beforeValidate(): void
{...
24 Replies
You need to set your column for date to be nullable in the db.
it is already
$table->timestamp('follow_up')->nullable();
email has nothing to do with a valid date? which I suspect is follow_up
Sorry I was copying the wrong line of code. The problem is still the same. Updated the post above
I have tried to cast the field to null but it always says "must be valid date".Is that an exception or a validation message?
This is a validation message I see in the document at the end of an row
No matter what I always get validation errors, struggling for days now.
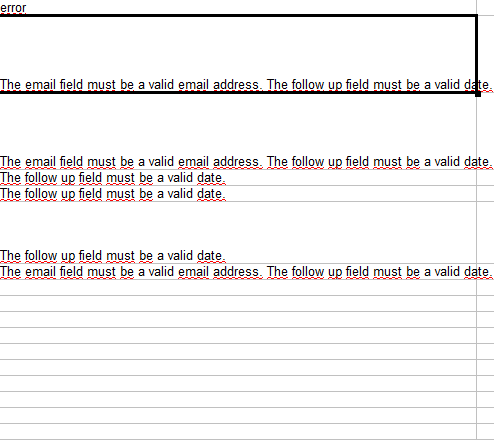
Provide your test CSV
Can't provide the whole file, but I want not valid data to be stored as null
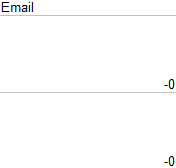
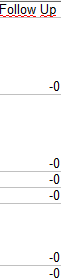
You need to remove not valid data then and just cast it.
So you mean I should only provide valid data in my files?
No, you can cast it https://filamentphp.com/docs/3.x/actions/prebuilt-actions/import#casting-state
which adjusts that it will be, so casting to null if invalid will work if you allow nulls in that column.
Tried that already, but no success
And what is the state? is the state null? If it iss then your not allow to store nulls.
Remove the rules too
Did that already
How to change that?
try {
$date = new \DateTime($value);
$newState = $date->getTimestamp();
} catch (\Exception $e) {
$newState = null;
}
dd($newState);
ok
does nothing
Interesting, try logging it instead. The importer could be catching the exception
Logging on the try and the catch
Ok
I get nothing in my log
same here
Is there someone else who has an idea how to handle this? Please really need this
maybe using a beforeValidate?
$this->data['follow_up'] = $this->data['follow_up'] ? Carbon::parse($this->data['follow_up']) : null
https://filamentphp.com/docs/3.x/actions/prebuilt-actions/import#lifecycle-hooksThanks I will try this 🙂
If it doesn't work, provide a mini repo on Github to reproduce this issue.. I can take a look
Oh wow thanks for your effort
Will check it later no time at the moment
Solution
Thanks solved it like so