How do I recursively wrap a `JSX.Element` with an `Array<FlowComponent>`
I think turning it into this is fine. But keeping the reactivity is a little challenging.
13 Replies
I'm trying to accomplish something similar to this:
https://www.reddit.com/r/solidjs/comments/14m5bde/cant_figure_out_why_i_cant_stack_wrapper/
You could try following how it's done for the
MultiProvider
in solid-primitives
https://github.com/solidjs-community/solid-primitives/blob/8e6089531dea15947d9c4671d764fe861413f2e5/packages/context/src/index.ts#L102GitHub
solid-primitives/packages/context/src/index.ts at 8e6089531dea15947...
A library of high-quality primitives that extend SolidJS reactivity. - solidjs-community/solid-primitives
MultiProvider
doesn't take into account that the values can change, so you might need to wrap with createMemo
or use <>{render(0)}</>
Thanks @REEEEE ! It seemed to work but I'm getting hydration issues. (Essentially the backend rendered my component differently, and the frontend also rendered it differently). Maybe because for my case, I'm rendering UI as well (other than context).
Wondering if @mdynnl 's solution would work. Can you elaborate on using createMemo here?
Also,
render(0)
, do you mean the fn(0)
used in the MultiProvider
example?yeah, i was looking at an old thread related to inertia layouts and that slipped in
Btw for context, I'm trying to code Vike's SSR for rendering cumulative layouts atm.
Basically, there's a
layouts: FlowComponent[]
and a children: JSX.Element
.
I'm trying to wrap children with a bunch of layouts essentially.
I tried doing your solution but no luckThis is what I wrote so far
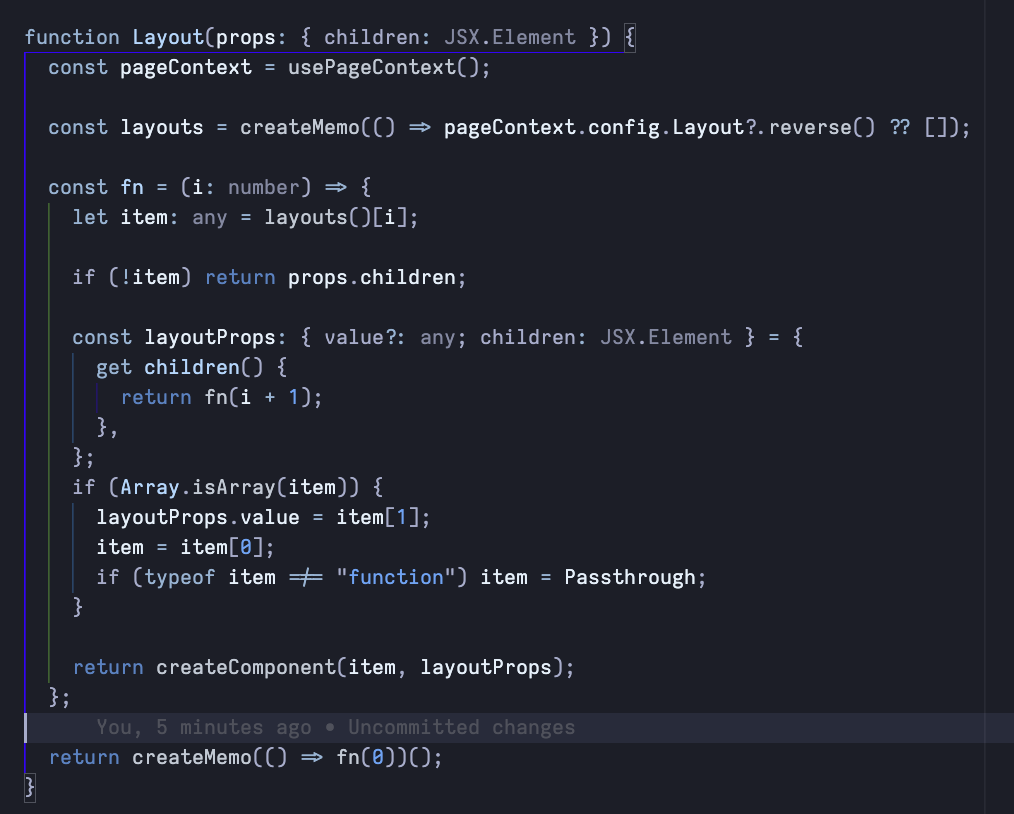
i think soid-js need better hydration strategy
can't have adjacent fragment layouts
Interesting! Thanks for this.
it needs some elements for hydration marker
the logic acutally comes from
context: https://discord.com/channels/722131463138705510/1108166822286602361/1108504704201277451
MultiProvider
context: https://discord.com/channels/722131463138705510/1108166822286602361/1108504704201277451
Ah man.. Just tried it, unfortunately still doesn't work. I also don't have fragments for the layouts.
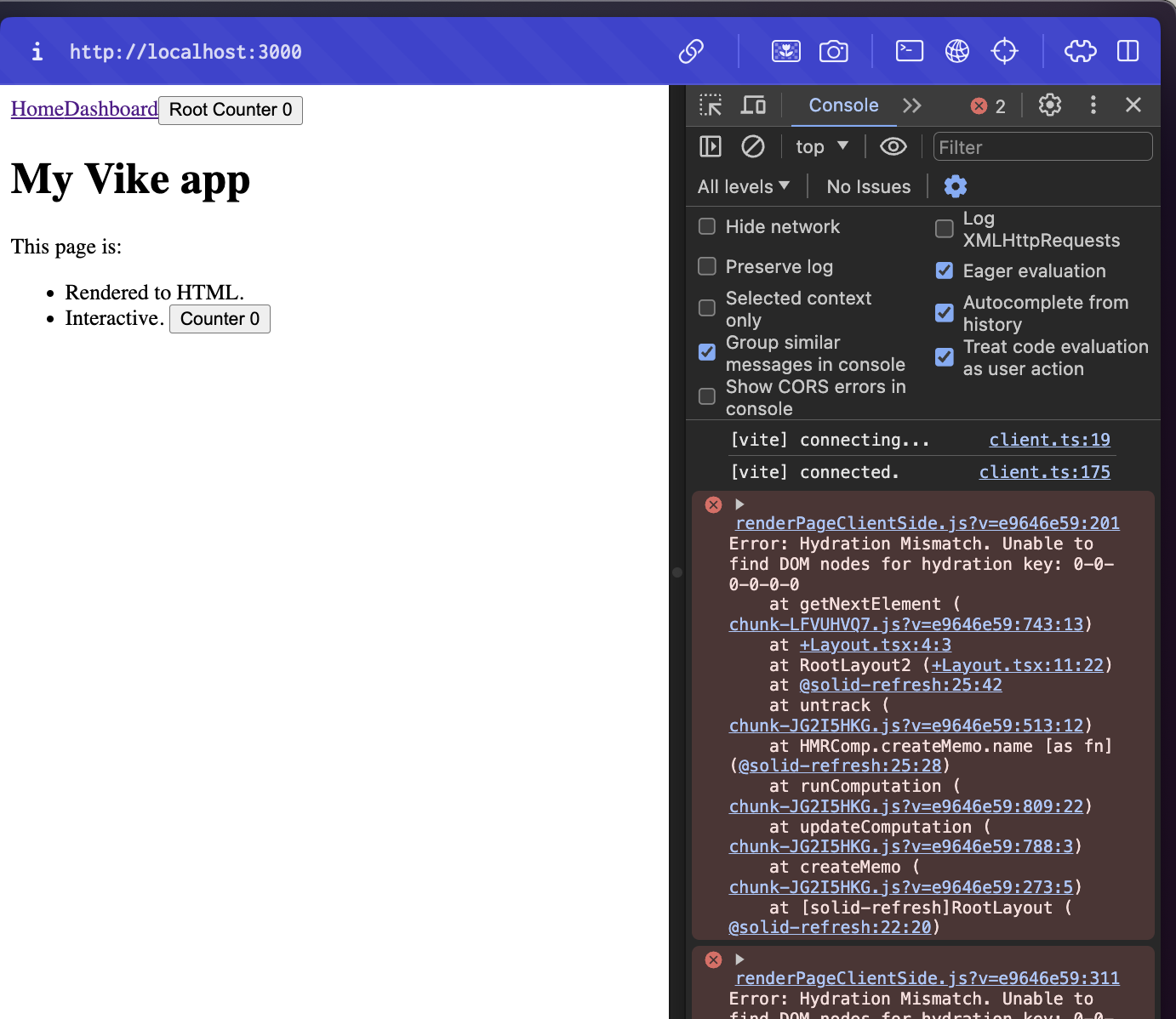
Thanks bud. Was able to solve it thanks to the links!