How to fix a simple (hopefully) error?
I am doing a leetcode solution, and I have this so far:
I am getting this error
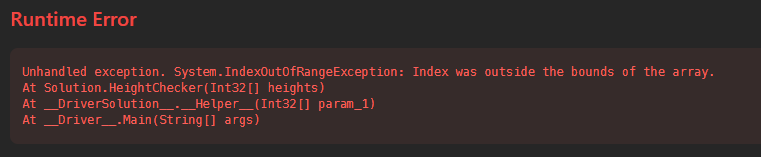
37 Replies
I'm a beginner so if anything here is bad practice please let me know
index out of range means you tried to access an array element that doesn't exist
valid array indexes are from 0 to 1 less than the length of the array
also, arrays are fixed size so if you're trying to put elements into an empty array it won't work
so how should i do it instead
make the array large enough to hold the data you want to put in it, or use a
List
which automatically expands to add itemsso would making correctOrder a list fix that error?
if you change to a list and call Add, that would be one solution
or just make the array big enough to hold all the heights like
var correctOrder = new int[heights.Length];
I switched it to a list
looks like a similar if not the same error

and call Add
you have to do that to put new items in a listcan add put it in a specific spot
you could use
Insert
for thatIll try that real quick'
Still the same error
correctOrder[heights[iter-1]]
there will not be anything here, the list is emptyshould i just make correctOrder the same as heights but in list form in the beginning
is that possible
if your "correct order" is a known fixed size there's no reason to use the list
then why'd you tell me to
it sounds like what you want is https://discord.com/channels/143867839282020352/1249757566041325718/1249759651784822947
Jimmacle
or just make the array big enough to hold all the heights like
var correctOrder = new int[heights.Length];
Quoted by
<@901546879530172498> from #How to fix a simple (hopefully) error? (click here)
React with ❌ to remove this embed.
i'm giving you multiple options, not the answer
which option is the best then
that's up to you, i have no idea what the actual problem being solved is
i'm just going based off of a code snippet
lists are generally for collections that change size, arrays can be used for collections that are always the same size
so array would make more sense
but i dont understand where i am getting a negative index
foreach(int i in heights)
seems off
this will give you the values in heights, not the indexes
are you sure you don't want a for loop?i need all the values, then i am sorting them smallest to biggest
then comparing the new sorted array to the old one to see how many are wrong
should i use a for loop?
updated code, similar error

if you need the index, use a for loop
your code doesn't make sense because you're using the actual height value as an index into the array
i need the values, no?
i
is not storing an index in your code, it's storing a height
then you're trying to use it as an index into the arrays, which is probably where your bad index is coming fromswitched it and im still getting that same error ^
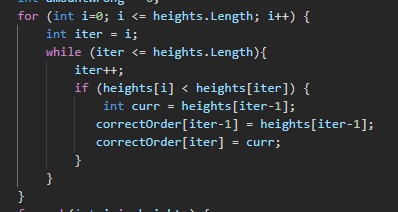
i <= heights.Length
remember what i said about valid indexes
heights.Length
would be 1 past the end of the arrayokay that makes sense
so heights.Length-1 then
or just
i < heights.Length
same error still
then you have more places where you're making mistakes
can you run this code in an actual IDE with a debugger?
it will tell you exactly which line is causing the exception
yeah give me a second
You have 2 of that checks, did you change both?
And it reminds me of bubble sort, isnt it?
Can you show full code?