Repeater have problem
Hi
I have create depot delivery form.
After I send form I have error (photo). How I can resolve this? Products is a relationship, I have table
delivery_products
for this products.21 Replies
And mutate data
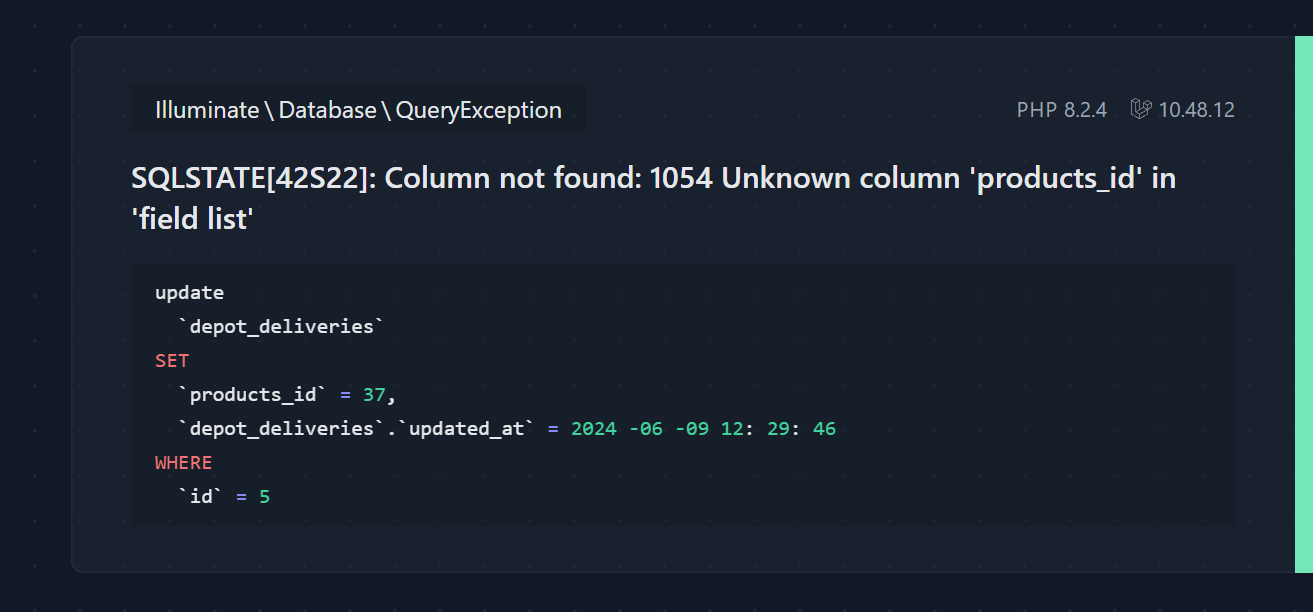
If you are trying to do this https://demo.filamentphp.com/shop/orders/create
I recommend check the demo project: https://github.com/filamentphp/demo
GitHub
GitHub - filamentphp/demo: Source code for the demo.filamentphp.com...
Source code for the demo.filamentphp.com website. Contribute to filamentphp/demo development by creating an account on GitHub.
Omg
That was too easy
Thanks bro ❤️
Whats wrong?
This
Doesn't work. Without where() works, with where doesnt work 😦
what is the value from
depot_id
? Is it live?->reactive()
isn't necessary if you are using live on V3
if you change depot_id, the query should work in the product_id..I remove
->reactive()
and this still not working
what is the value from depot_id?
Value is id, label is name
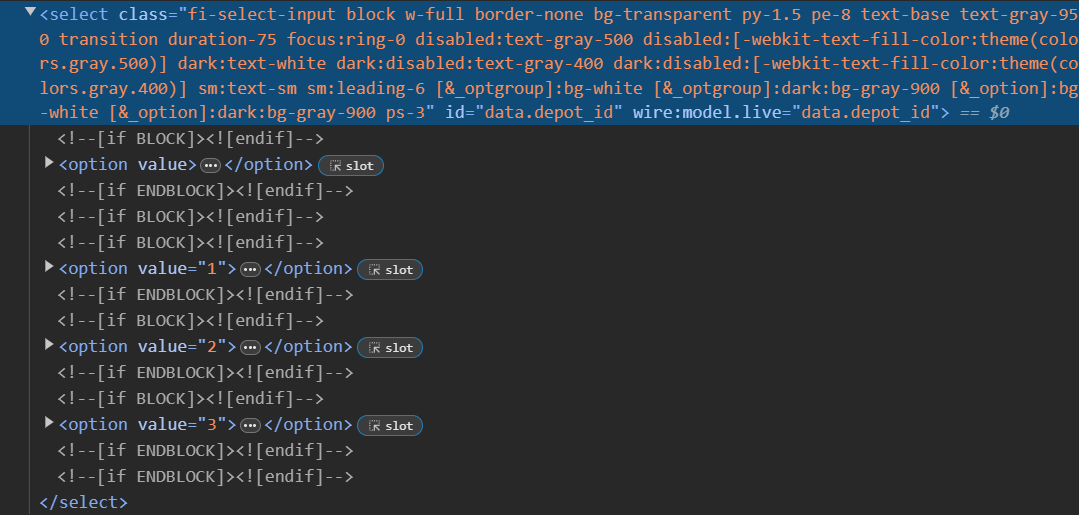
use this instead of options
I understand that
->whereDepotId()
is a scope?short way of
->where('depot_id', $get('depot_id'))
Still not working
hum, not sure why
Could you share the whole code you are using?
Github or only this resource and models?
are you able to share the project on github?
I have it shared, but privately. Is it a problem if you gave me your email address?
no problem
Sorry, I didn't notice that you are using a repeater.
This should be
$get('../../depot_id')
https://filamentphp.com/docs/3.x/forms/fields/repeater#using-get-to-access-parent-field-values
✌️