Computed properties from useState inside a composable is called multiple times
Hi, Im currently doing some state sharing between components with composable. From this video https://www.youtube.com/watch?v=mv0WcBABcIk, the usage is like
and the state should be shared across the 2 components.
However the issue comes when I try to implement computed and watch properties inside these hooks
In this case, the computed and watch properties will be executed n times for every doSomething() call, where n is the number of components that have useSomething() mounted
Is there a way to properly implement this behaviour without triggering computed a bunch of times?
Alexander Lichter
YouTube
Why you should use useState()
VUEJS AMSTERDAM DISCOUNT (20%): i-really-like-alexander-lichter
π Sending state from the server to the client is tricky but necessary when using SSR. And global state management is not the easiest part of an app either. Nuxt's useState composable solves both of these issues - but how? You'll learn in this weeks video!
Key points:
β©οΈ How to se...

19 Replies
Your computed seems to be async (fetchResults is, no?)
Agree, async computed is probably very bad. But aside from the async function, if I were to replace it with console log it still seems to execute multiple times along side watch each time the dependency changes
I can create a code sandbox to demonstrate what I meant
It seems like the code sandbox is broken with vue/nuxt right now, https://github.com/codesandbox/codesandbox-client/issues/8451
GitHub
Vue purported vnode error in CodeSandbox Β· Issue #8451 Β· codesandbo...
π bug report Preflight Checklist [x ] I have read the Contributing Guidelines for this project. [x ] I agree to follow the Code of Conduct that this project adheres to. [x ] I have searched the iss...
try stackblitz instead π
ShuviSchwarze
StackBlitz
Nuxt - Starter (forked) - StackBlitz
Create a new Nuxt project, module, layer or start from a theme with our collection of starters.
I couldnt get console.log to work so I just have the sideeffect as a state count instead
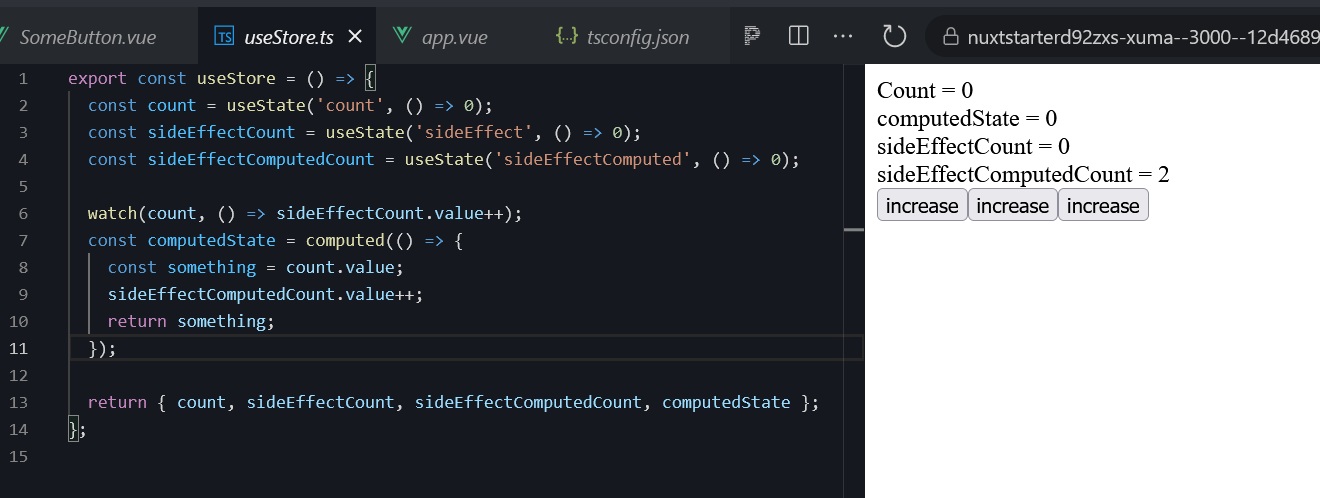
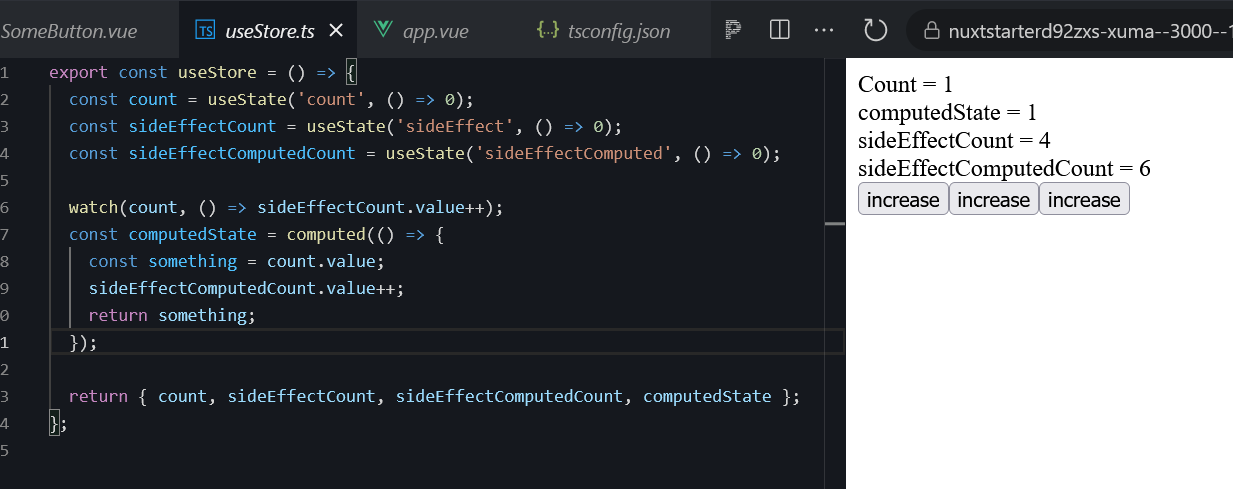
Some button has the hook mounted, so in stotal the watch effect is mounted 4 times
@manniL / TheAlexLichter sorry for bumping this, but I ran into another behaviour in having side effects in computed, this time interacting with pinia store
https://stackblitz.com/edit/nuxt-starter-izzlz4?file=README.md
ShuviSchwarze
StackBlitz
Nuxt - Starter (forked) - StackBlitz
Create a new Nuxt project, module, layer or start from a theme with our collection of starters.
somehow computed is reacting to changes from count in useCounterStore
Is there any explanation to why this is the behavior? It seems like computed is prone to rerunning too often. I know having side effects in computed is a bad practice, but this firing behavior would affect cases where computed function is expensive too
FYI computeds also shouldnβt have side effects
Better to watch that computed
As there is no guarantee how often itβll be executed/recalculated
but watch in this case is also re-executing multiple times as seen with the initial minimal reproduction
https://stackblitz.com/edit/nuxt-starter-d92zxs?file=useStore.ts,app.vue
I dont think either watch or computed are reliable inside a custom hook if you have any side effect at all
StackBlitz
Nuxt - Starter (forked) - StackBlitz
Create a new Nuxt project, module, layer or start from a theme with our collection of starters.
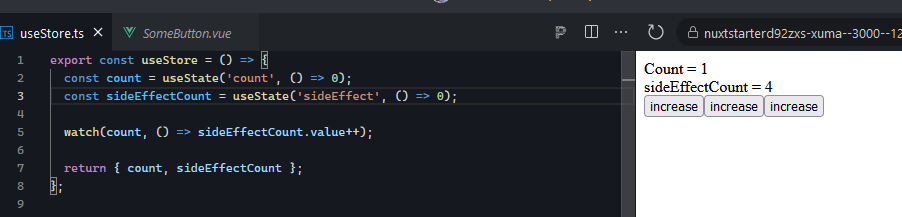
Using watch in pinia with the same pattern seems to produce the appropriate behaviour
no no, it is correct
as you create a watcher per button when initiating the composable
(for each
SomeButton
)Yes, but isnt setting state with this pattern not ideal? I dont think it is appropriate for the use case
Do you suggest using any other pattern or just stick with pinia?
Unfortunately "it depends" - basically on what you want to do
pinia is totally fine π
I guess for these kind of watch Ill just use a pinia store then, though I do think it is a bit of a pitfall that computed state is running so often.
Thank you for your timeπ
no problem at all!