14 Replies
heres the code
Please review how JavaScript arrow functions work: https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Functions/Arrow_functions
Currently your
registerChatInputCommand
method isn't returning anything so the error is that you're providing undefined
where string
was expected, which is for name
because you're not actually setting it. You need to either remove the wrapping { }
or add a return
keyword.
One of these 2:
MDN Web Docs
Arrow function expressions - JavaScript | MDN
An arrow function expression is a compact alternative to a traditional function expression, with some semantic differences and deliberate limitations in usage:
Sidenote that you really dont need a help command when using slash commands btw because the list of commands is integrated in the Discord client when the user types a single
/
the dude im coding this for wants
alright :monkashrug:
wait what's wrong with my arrow function
i took my messaage command i made for this and used chatgpt to convert it to a slash command
@Boomeravna also how can i make my code register the slash command only in a single guild
I literally told you. Your syntax is wrong right now.
please read my message again
i have fixed it.

IT IS RETURNING
sorry caps
Sapphire Framework
Registering Chat Input Commands | Sapphire
To register a Chat Input Command (also known as a Slash Command) with Discord, you need to acquire an application
It is returning the name which is string isn't it


now it registered this shit twice
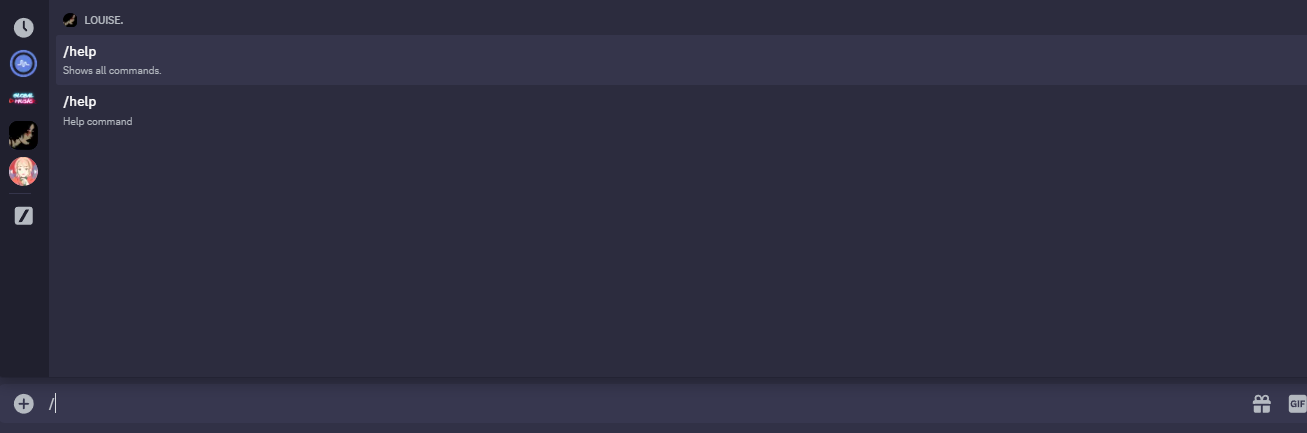
you didnt have that
return
keyword beforeremove one or both then re-register. Because the changing data Sapphire was unable to detect similarity. Refer to these docs to learn more:
- https://sapphirejs.dev/docs/Guide/commands/application-commands/application-command-registry/registering-chat-input-commands#behaviorwhennotidentical
- https://sapphirejs.dev/docs/Guide/commands/application-commands/application-command-registry/registering-chat-input-commands#idhints
- https://sapphirejs.dev/docs/Guide/commands/application-commands/application-command-registry/advanced/setting-global-behavior-when-not-identical