Do something when a submission ends if there's more than 1 action
Hey everyone. For showing user feedback (like a toast) when an action was done I was checking the
action.result
inside a createEffect
where action
is the returned value from useSubmission(myAction)
This has been working fine but breaks the moment I use more than one useSubmission
in the same component. All effects run on success even if just one action was fired (in my case showing multiple toasts)
What's the pattern for handling this?10 Replies
Would be easier if you shared some code you already have.
If your
useSubmission
isn't context signal I don't see why would it trigger other signals.
I guess you have something like this?
Here's the example in playground
https://playground.solidjs.com/anonymous/728692bc-1edc-429f-a48e-dfa2e78cafc2
In console you get only single action result on button click
Solid Playground
Quickly discover what the solid compiler will generate from your JSX template
@Maciek50322 Thanks for your response! Note I'm talking about
useSubmission
exported by solid router, not a custom hook. Your example is pretty much the case, except with two useSubmission using a form and a formAction
on a button to trigger different server actions.
Ok, I never really used solid router, but looking at source code I see that it uses
useRouter
(which uses context), which holds single signal for all sumbissions. I guess it then triggers all submissions' results when one of them changes. I also see that it can get some custom filter, that depends on sumbmission's input (idk what that really means, but maybe you can try work something out with that, take a look here https://github.com/solidjs/solid-router/blob/main/src/data/action.ts#L21 )
Seeing how it's done it was probably designated to have 1 submission per pageGitHub
solid-router/src/data/action.ts at main · solidjs/solid-router
A universal router for Solid inspired by Ember and React Router - solidjs/solid-router
As a workaround you can always check yourself if the result changed
Can you console.log
myServerAction1.url
and myServerAction2.url
?
The behaviour suggests that they are identical.Thank you@peerreynders sorry for the late response!
They are functions in the same file, but they are separate and the url does seem different
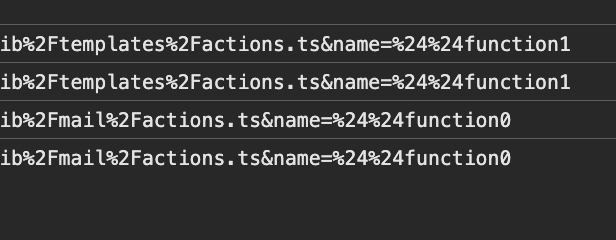
As it turns out, this is just a variation on the theme already established earlier
The difference here is that you trigger on the
true -> false
transition of pending
, so undefined
or identical successive results aren't a problem; they will still trigger properly with each "new" result.
This version explains a bit more of what is going on.
@peerreynders thanks!