Cloud Flare Context is not defined when using Session
Im getting this message every time i try to call a server action that calls useSession hook
it only happens on CloudFlare preset builds. On preset cloudflare-modules
the error is
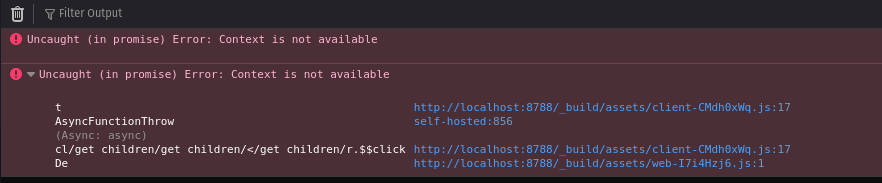
2 Replies
Just a heads up; given that you say that this occurs inside an action (i.e. the response hasn't been created yet, so it couldn't have started streaming) it should not be relevant but FYI …
useSession
will always create (i.e. try to update) a session if there isn't one already which won't work if the response has already started streaming (this typically happens during SSR, not actions).
The action itself may be helpful to provide "context" to whoever may be more knowledgeable about Cloudflare and its idiosyncrasies.
Rubberduck out.Rubber duck debugging
In software engineering, rubber duck debugging (or rubberducking) is a method of debugging code by articulating a problem in spoken or written natural language. The name is a reference to a story in the book The Pragmatic Programmer in which a programmer would carry around a rubber duck and debug their code by forcing themselves to explain it, l...
I refactored the code a bit, based on the "with-auth" template
it is probably a Cloud Flare Wrangler issue since on node-server preset it works as expected
the erros happens when trying to call login or logout
here it is some code