Application !Closing
Good day folk, first time Svelte app tinkerer here. I am having a little bit of an issue getting this app to close after either button is pressed. Any ideas where I am failing?
<script>
import { createEventDispatcher } from 'svelte';
import { ApplicationShell } from '#runtime/svelte/component/core';
import { FatesDescentRoll } from '../FatesDescentRoll.js';
export let actorId;
export let type;
export let config;
export let performRoll = FatesDescentRoll.performRoll;
export let elementRoot;
let severities = [
{ id: 'minimal', text: 'Minimal (DC 8)' },
{ id: 'moderate', text: 'Moderate (DC 12)' },
{ id: 'serious', text: 'Serious (DC 16)' },
{ id: 'extreme', text: 'Extreme (DC 20)' }
];
let selectedSeverity = severities[0];
let useCustomDC = false;
let customDC = 0;
let useCustomLoss = false;
let loss = 0;
const dispatch = createEventDispatcher();
function handleRoll() {
const lossInput = useCustomLoss ? loss : { 'minimal': '1d4', 'moderate': '1d6', 'serious': '1d8', 'extreme': '1d10' }[selectedSeverity.id];
performRoll(actorId, selectedSeverity.id, customDC, type, lossInput, config, useCustomDC, useCustomLoss);
closeApp();
}
function handleCancel() {
const method = type === 'save' ? 'rollAbilitySave' : 'rollAbilityTest';
const rollOptions = {
chatMessage: true,
fastForward: true,
fromDialog: true,
advantage: config?.advantage || false,
disadvantage: config?.disadvantage || false
};
const actor = game.actors.get(actorId);
if (actor) {
actor[method]("san", rollOptions);
}
closeApp();
}
function closeApp() {
dispatch('close');
}
const dialogTitle = type === 'save' ? 'Sanity Save' : 'Sanity Check';
</script>
<svelte:options accessors={true}/>
<ApplicationShell bind:elementRoot>
<main>
<h2><i class="fas fa-dice"></i> {dialogTitle} <i class="fas fa-dice"></i></h2>
<form on:submit|preventDefault={handleRoll}>
<label>
Severity:
<select bind:value={selectedSeverity} on:change={() => { customDC = 0; loss = 0; }}>
{#each severities as severity}
<option value={severity}>{severity.text}</option>
{/each}
</select>
</label>
<label>
Custom DC: <input type="checkbox" bind:checked={useCustomDC} />
<input type="text" bind:value={customDC} placeholder="DC" disabled={!useCustomDC} />
</label>
<label>
Loss: <input type="checkbox" bind:checked={useCustomLoss} />
<input type="text" bind:value={loss} placeholder="Loss" disabled={!useCustomLoss} />
</label>
<button disabled={selectedSeverity === null} type="submit">Roll</button>
<button type="button" on:click={handleCancel}>Cancel</button>
</form>
<p>Selected severity: {selectedSeverity ? selectedSeverity.text : '[waiting...]'}</p>
</main>
</ApplicationShell>
<style>
main {
background: #222;
color: #f8f8f8;
padding: 5px;
border-radius: 8px;
display: flex;
flex-direction: column;
align-items: center;
}
form {
display: flex;
flex-direction: column;
align-items: flex-start;
}
label {
display: block;
margin: 10px 0;
font-size: 1.4em;
}
input[type='text'], input[type='checkbox'], select {
margin-left: 10px;
background: #333;
color: #f8f8f8;
border: 1px solid #444;
padding: 2px;
border-radius: 4px;
font-size: 12px;
}
input[type='text'] {
width: 100px;
}
button {
background: #f8f8f8;
color: #222;
border: none;
padding: 10px 20px;
border-radius: 4px;
font-weight: bold;
font-size: 16px;
margin: 10px 0;
cursor: pointer;
}
button:hover {
background: #555;
}
</style>
<script>
import { createEventDispatcher } from 'svelte';
import { ApplicationShell } from '#runtime/svelte/component/core';
import { FatesDescentRoll } from '../FatesDescentRoll.js';
export let actorId;
export let type;
export let config;
export let performRoll = FatesDescentRoll.performRoll;
export let elementRoot;
let severities = [
{ id: 'minimal', text: 'Minimal (DC 8)' },
{ id: 'moderate', text: 'Moderate (DC 12)' },
{ id: 'serious', text: 'Serious (DC 16)' },
{ id: 'extreme', text: 'Extreme (DC 20)' }
];
let selectedSeverity = severities[0];
let useCustomDC = false;
let customDC = 0;
let useCustomLoss = false;
let loss = 0;
const dispatch = createEventDispatcher();
function handleRoll() {
const lossInput = useCustomLoss ? loss : { 'minimal': '1d4', 'moderate': '1d6', 'serious': '1d8', 'extreme': '1d10' }[selectedSeverity.id];
performRoll(actorId, selectedSeverity.id, customDC, type, lossInput, config, useCustomDC, useCustomLoss);
closeApp();
}
function handleCancel() {
const method = type === 'save' ? 'rollAbilitySave' : 'rollAbilityTest';
const rollOptions = {
chatMessage: true,
fastForward: true,
fromDialog: true,
advantage: config?.advantage || false,
disadvantage: config?.disadvantage || false
};
const actor = game.actors.get(actorId);
if (actor) {
actor[method]("san", rollOptions);
}
closeApp();
}
function closeApp() {
dispatch('close');
}
const dialogTitle = type === 'save' ? 'Sanity Save' : 'Sanity Check';
</script>
<svelte:options accessors={true}/>
<ApplicationShell bind:elementRoot>
<main>
<h2><i class="fas fa-dice"></i> {dialogTitle} <i class="fas fa-dice"></i></h2>
<form on:submit|preventDefault={handleRoll}>
<label>
Severity:
<select bind:value={selectedSeverity} on:change={() => { customDC = 0; loss = 0; }}>
{#each severities as severity}
<option value={severity}>{severity.text}</option>
{/each}
</select>
</label>
<label>
Custom DC: <input type="checkbox" bind:checked={useCustomDC} />
<input type="text" bind:value={customDC} placeholder="DC" disabled={!useCustomDC} />
</label>
<label>
Loss: <input type="checkbox" bind:checked={useCustomLoss} />
<input type="text" bind:value={loss} placeholder="Loss" disabled={!useCustomLoss} />
</label>
<button disabled={selectedSeverity === null} type="submit">Roll</button>
<button type="button" on:click={handleCancel}>Cancel</button>
</form>
<p>Selected severity: {selectedSeverity ? selectedSeverity.text : '[waiting...]'}</p>
</main>
</ApplicationShell>
<style>
main {
background: #222;
color: #f8f8f8;
padding: 5px;
border-radius: 8px;
display: flex;
flex-direction: column;
align-items: center;
}
form {
display: flex;
flex-direction: column;
align-items: flex-start;
}
label {
display: block;
margin: 10px 0;
font-size: 1.4em;
}
input[type='text'], input[type='checkbox'], select {
margin-left: 10px;
background: #333;
color: #f8f8f8;
border: 1px solid #444;
padding: 2px;
border-radius: 4px;
font-size: 12px;
}
input[type='text'] {
width: 100px;
}
button {
background: #f8f8f8;
color: #222;
border: none;
padding: 10px 20px;
border-radius: 4px;
font-weight: bold;
font-size: 16px;
margin: 10px 0;
cursor: pointer;
}
button:hover {
background: #555;
}
</style>
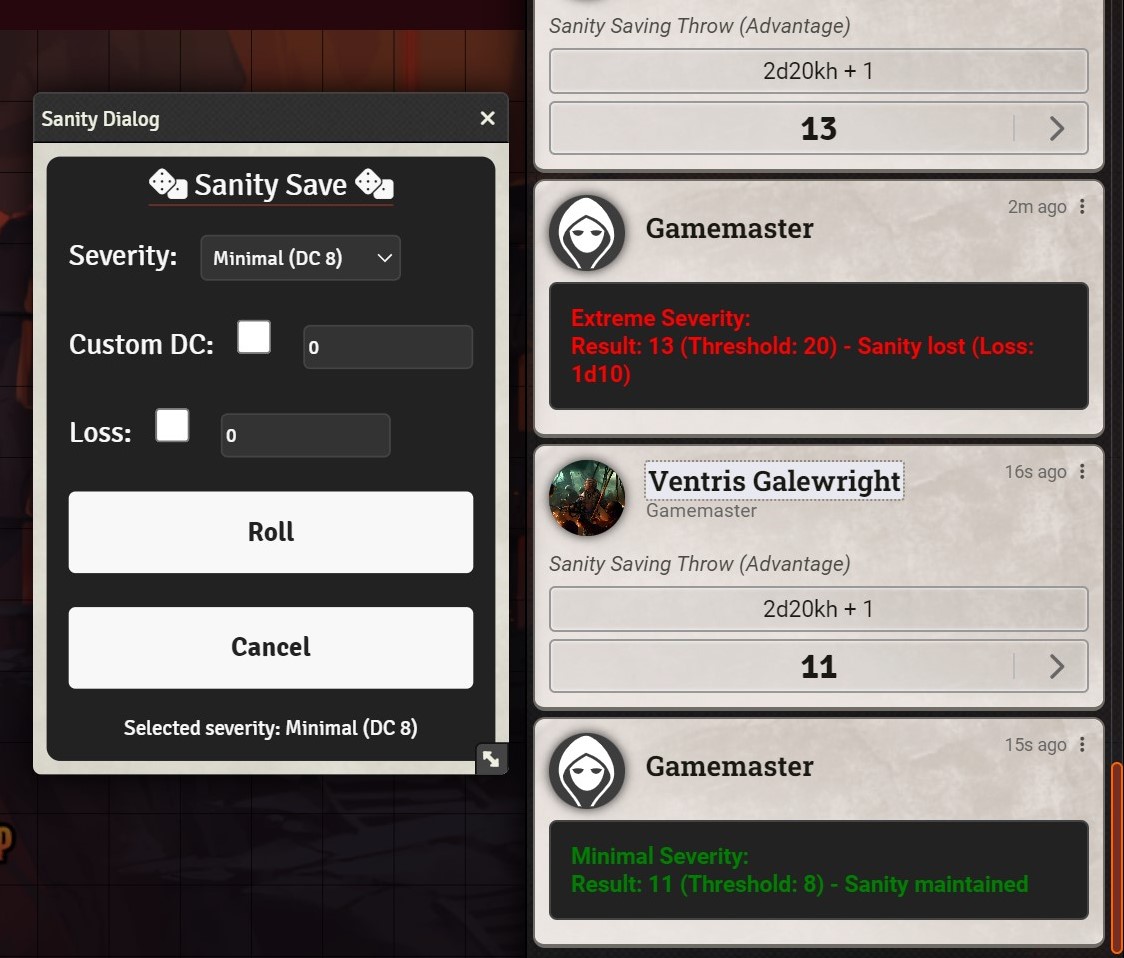
6 Replies
Good initial progress. With TRL there is a Svelte context available that has external references. To get the outer SvelteApplication based JS class instance you can use the
You can remove the the use of
#external
context.
import { getContext } from 'svelte';
const { application } = getContext('#external');
// When you want to close the app invoke the close method.
function handleCancel() {
// Do stuff
application.close();
}
import { getContext } from 'svelte';
const { application } = getContext('#external');
// When you want to close the app invoke the close method.
function handleCancel() {
// Do stuff
application.close();
}
createEventDispatcher
and the dispatch of close
.So like this
Well let me get rid of the unused method too
Yep that did it
Taking note of that getContext
<script>
import { getContext } from 'svelte';
import { ApplicationShell } from '#runtime/svelte/component/core';
import { FatesDescentRoll } from '../FatesDescentRoll.js';
export let actorId;
export let type;
export let config;
export let performRoll = FatesDescentRoll.performRoll;
export let elementRoot;
const { application } = getContext('#external');
let severities = [
{ id: 'minimal', text: 'Minimal (DC 8)' },
{ id: 'moderate', text: 'Moderate (DC 12)' },
{ id: 'serious', text: 'Serious (DC 16)' },
{ id: 'extreme', text: 'Extreme (DC 20)' }
];
let selectedSeverity = severities[0];
let useCustomDC = false;
let customDC = 0;
let useCustomLoss = false;
let loss = 0;
function handleRoll() {
const lossInput = useCustomLoss ? loss : { 'minimal': '1d4', 'moderate': '1d6', 'serious': '1d8', 'extreme': '1d10' }[selectedSeverity.id];
performRoll(actorId, selectedSeverity.id, customDC, type, lossInput, config, useCustomDC, useCustomLoss);
application.close();
}
function handleCancel() {
const method = type === 'save' ? 'rollAbilitySave' : 'rollAbilityTest';
const rollOptions = {
chatMessage: true,
fastForward: true,
fromDialog: true,
advantage: config?.advantage || false,
disadvantage: config?.disadvantage || false
};
const actor = game.actors.get(actorId);
if (actor) {
actor[method]("san", rollOptions);
}
application.close();
}
function closeApp() {
dispatch('close');
}
const dialogTitle = type === 'save' ? 'Sanity Save' : 'Sanity Check';
</script>
<svelte:options accessors={true}/>
<ApplicationShell bind:elementRoot>
<main>
<h2><i class="fas fa-dice"></i> {dialogTitle} <i class="fas fa-dice"></i></h2>
<form on:submit|preventDefault={handleRoll}>
<label>
Severity:
<select bind:value={selectedSeverity} on:change={() => { customDC = 0; loss = 0; }}>
{#each severities as severity}
<option value={severity}>{severity.text}</option>
{/each}
</select>
</label>
<label>
Custom DC: <input type="checkbox" bind:checked={useCustomDC} />
<input type="text" bind:value={customDC} placeholder="DC" disabled={!useCustomDC} />
</label>
<label>
Loss: <input type="checkbox" bind:checked={useCustomLoss} />
<input type="text" bind:value={loss} placeholder="Loss" disabled={!useCustomLoss} />
</label>
<button disabled={selectedSeverity === null} type="submit">Roll</button>
<button type="button" on:click={handleCancel}>Cancel</button>
</form>
<p>Selected severity: {selectedSeverity ? selectedSeverity.text : '[waiting...]'}</p>
</main>
</ApplicationShell>
<style>
main {
background: #222;
color: #f8f8f8;
padding: 5px;
border-radius: 8px;
display: flex;
flex-direction: column;
align-items: center;
}
form {
display: flex;
flex-direction: column;
align-items: flex-start;
}
label {
display: block;
margin: 10px 0;
font-size: 1.4em;
}
input[type='text'], input[type='checkbox'], select {
margin-left: 10px;
background: #333;
color: #f8f8f8;
border: 1px solid #444;
padding: 2px;
border-radius: 4px;
font-size: 12px;
}
input[type='text'] {
width: 100px;
}
button {
background: #f8f8f8;
color: #222;
border: none;
padding: 10px 20px;
border-radius: 4px;
font-weight: bold;
font-size: 16px;
margin: 10px 0;
cursor: pointer;
}
button:hover {
background: #555;
}
</style>
<script>
import { getContext } from 'svelte';
import { ApplicationShell } from '#runtime/svelte/component/core';
import { FatesDescentRoll } from '../FatesDescentRoll.js';
export let actorId;
export let type;
export let config;
export let performRoll = FatesDescentRoll.performRoll;
export let elementRoot;
const { application } = getContext('#external');
let severities = [
{ id: 'minimal', text: 'Minimal (DC 8)' },
{ id: 'moderate', text: 'Moderate (DC 12)' },
{ id: 'serious', text: 'Serious (DC 16)' },
{ id: 'extreme', text: 'Extreme (DC 20)' }
];
let selectedSeverity = severities[0];
let useCustomDC = false;
let customDC = 0;
let useCustomLoss = false;
let loss = 0;
function handleRoll() {
const lossInput = useCustomLoss ? loss : { 'minimal': '1d4', 'moderate': '1d6', 'serious': '1d8', 'extreme': '1d10' }[selectedSeverity.id];
performRoll(actorId, selectedSeverity.id, customDC, type, lossInput, config, useCustomDC, useCustomLoss);
application.close();
}
function handleCancel() {
const method = type === 'save' ? 'rollAbilitySave' : 'rollAbilityTest';
const rollOptions = {
chatMessage: true,
fastForward: true,
fromDialog: true,
advantage: config?.advantage || false,
disadvantage: config?.disadvantage || false
};
const actor = game.actors.get(actorId);
if (actor) {
actor[method]("san", rollOptions);
}
application.close();
}
function closeApp() {
dispatch('close');
}
const dialogTitle = type === 'save' ? 'Sanity Save' : 'Sanity Check';
</script>
<svelte:options accessors={true}/>
<ApplicationShell bind:elementRoot>
<main>
<h2><i class="fas fa-dice"></i> {dialogTitle} <i class="fas fa-dice"></i></h2>
<form on:submit|preventDefault={handleRoll}>
<label>
Severity:
<select bind:value={selectedSeverity} on:change={() => { customDC = 0; loss = 0; }}>
{#each severities as severity}
<option value={severity}>{severity.text}</option>
{/each}
</select>
</label>
<label>
Custom DC: <input type="checkbox" bind:checked={useCustomDC} />
<input type="text" bind:value={customDC} placeholder="DC" disabled={!useCustomDC} />
</label>
<label>
Loss: <input type="checkbox" bind:checked={useCustomLoss} />
<input type="text" bind:value={loss} placeholder="Loss" disabled={!useCustomLoss} />
</label>
<button disabled={selectedSeverity === null} type="submit">Roll</button>
<button type="button" on:click={handleCancel}>Cancel</button>
</form>
<p>Selected severity: {selectedSeverity ? selectedSeverity.text : '[waiting...]'}</p>
</main>
</ApplicationShell>
<style>
main {
background: #222;
color: #f8f8f8;
padding: 5px;
border-radius: 8px;
display: flex;
flex-direction: column;
align-items: center;
}
form {
display: flex;
flex-direction: column;
align-items: flex-start;
}
label {
display: block;
margin: 10px 0;
font-size: 1.4em;
}
input[type='text'], input[type='checkbox'], select {
margin-left: 10px;
background: #333;
color: #f8f8f8;
border: 1px solid #444;
padding: 2px;
border-radius: 4px;
font-size: 12px;
}
input[type='text'] {
width: 100px;
}
button {
background: #f8f8f8;
color: #222;
border: none;
padding: 10px 20px;
border-radius: 4px;
font-weight: bold;
font-size: 16px;
margin: 10px 0;
cursor: pointer;
}
button:hover {
background: #555;
}
</style>
Also with your dialog you can do the following to remove the padding from
This alters inline styles. You can also of course set a CSS class to your dialog through the main
.window-content
via passing inline styles for the content area via a prop to ApplicationShell
.
// In script.
const stylesContent = { padding: '0' };
// In template.
<ApplicationShell bind:elementRoot {stylesContent}>
// In script.
const stylesContent = { padding: '0' };
// In template.
<ApplicationShell bind:elementRoot {stylesContent}>
SvelteApplication
class as it is an Application
and then target in static styles to remove the padding. This can be easier if you have many apps where you want to change the styles.Well I want to give it that lovely glass appearance like your esm testing module main app has, but I have another piece of functionality I need to incorporate. I first have to set some of these inputs on a Horizontal plain and prefix the Actor name onto because my intention is to just update the one App with all inbound roll requests if the App is already open. i was going to wait to do final stylings until i had that arrangement done.
Though I am going to toss that in now to see how it looks
Yeah that's already better
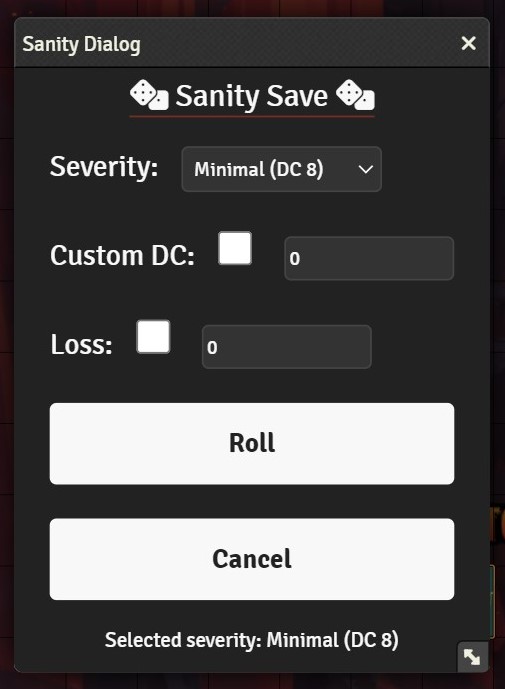
The main testing app doesn't have a
.window-content
background. You can try setting background: 'none'
in stylesContent
as well. Then you can also remove the background from your content component.