Why does calling the PrintFullName() method require casting it as ((Employee)PTE).PrintFullName() ?
Hi, I have two classes where one inherits from the other. Here's the code. My question is: why do I need to cast the method PrintFullName() when calling it as ((Employee)PTE).PrintFullName()? Why can't it be cast directly like (Employee)PTE.PrintFullName()?
11 Replies
Because the latter tries to cast the result of
PrintFullName
to Employee
i.e. a string
to an Employee
and string cant be converted to an employee
why do u use
new
?
you can override this method in "normal" wayso in here we are just type casting PTE that its type is PartTimeEmployee to an employee then we call the
PrintFullName()
method fro that object correct
but if we are doing it like that (Employee)PTE.PrintFullName()
this mehod is returning a string so in tha case we are convering a sring to employee
hi i am just readin about the mehod hiding in c# so i read that its recommended to use the new kewordin the drived class method but it i did`t add the new i will have a warring
you should not use
new
caz this hides your impl. You should avoid this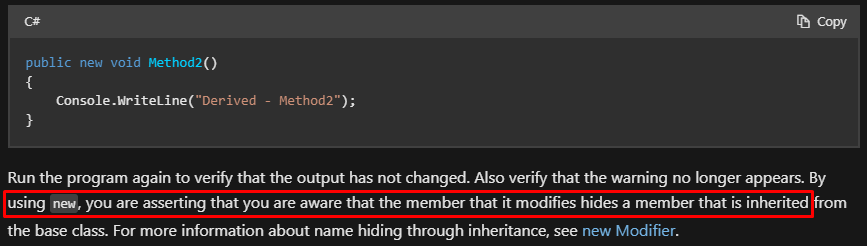
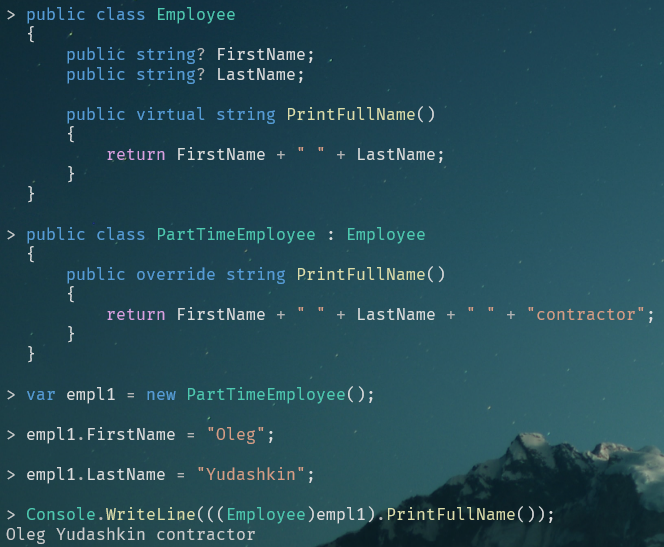
use
override
keywordi agree you can use it if you have the intention to hide the base class method
i didnt come to method overriding yet i know that its cleaner approch
@kurumi i mean i didt studied it yet
you can read more info here: https://learn.microsoft.com/en-us/dotnet/csharp/programming-guide/classes-and-structs/knowing-when-to-use-override-and-new-keywords
Knowing When to Use Override and New Keywords - C#
Use the new and override keywords in C# to specify how methods with the same name in a base and derived class interact.
correct
thank you
thank you very mush