My NextAuth session callback fetches the database too often
So.. I have this web app built with nextjs, the auth is done with next-auth (authjs). I have a session callback in which I set a few user properties such as default_team_id, verified, and id.
I am facing too many queries. More than 10k queries in a week of development. The session queries the database on every router refresh or navigation.
I appreciate any help. 🙏
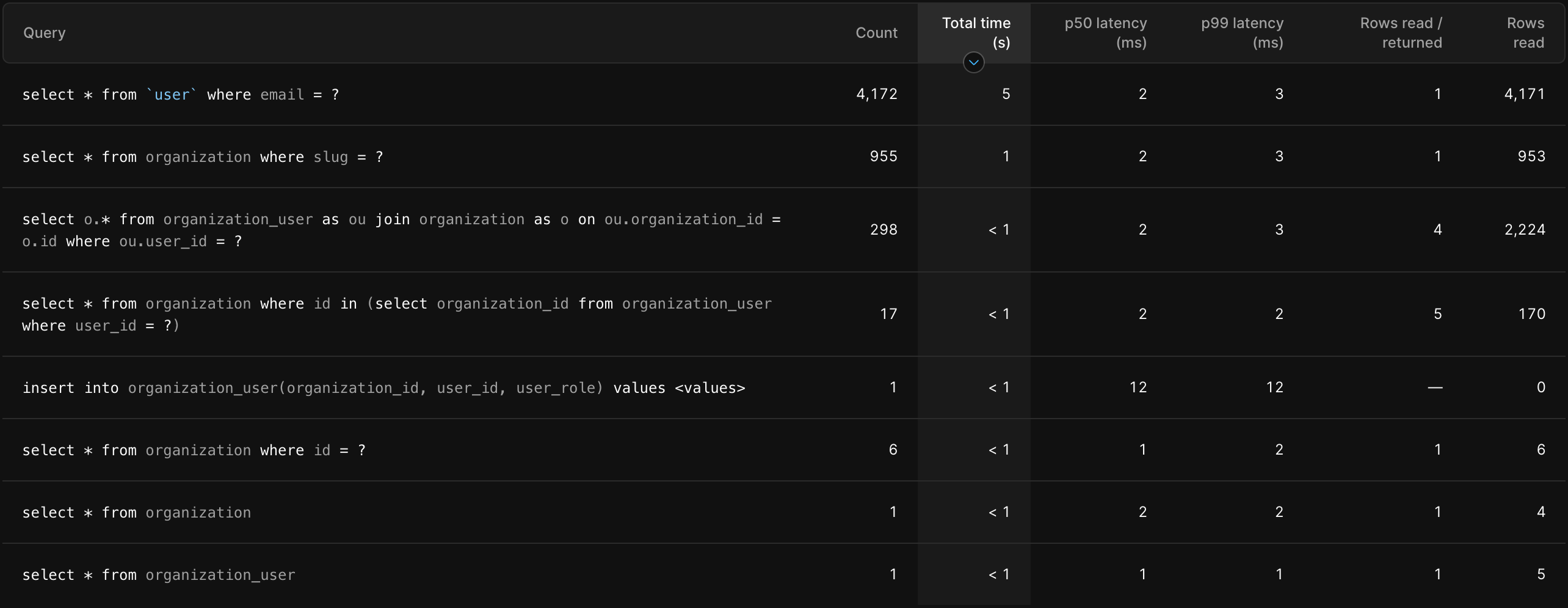
0 Replies