Query returning extra records that do not match
Wasp version: 0.13.2
Platform: WSL
Apologies if this is a dumb question, but I have been tearing my hair out for the past 2 hours trying to figure this out.
I have an entity called
PostVote
with 2 records in it. I have the following query which is meant to retrieve all votes that a user has made for a specific post:
However, when I call this query, I get the result shown in the attached image. No matter what post id I give it, it just returns all of the records in the PostVote
table, not just the ones with the postId
that I am looking for. Here is the code where it is being called:
And for reference this is the PostVote schema:
I can provide additional context if need be, please help me out if you can (trying to debug this is costing me my sanity)! Thank you π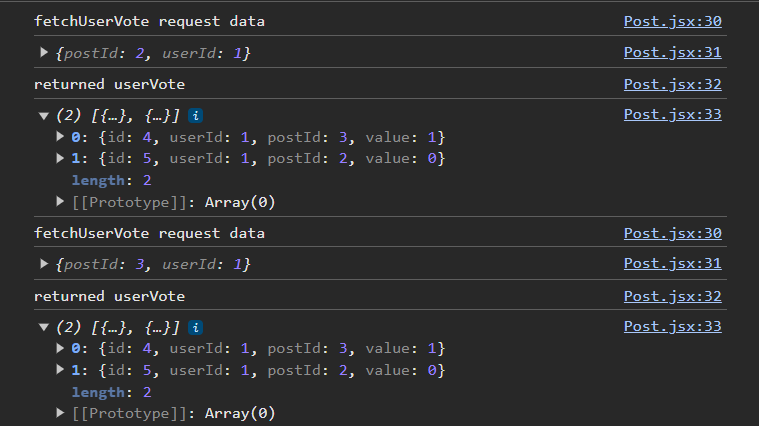
4 Replies
Hi @marto. I'm kapa.ai, your friendly support bot. I will try to answer your question by searching through the knowledge base. :wave:
----------------------
It seems like there might be an issue with the way you're structuring your
where
clause in the findMany
function. In Prisma, when you want to combine conditions with an AND operator, you don't need to use AND
explicitly. You can just list the conditions in the where
object.
Try changing your getUserPostVote
function to this:
This should return only the PostVote
records where both the postId
and userId
match the arguments passed to the function.
If you're still having issues, it might be helpful to check the Wasp documentation on implementing queries (https://wasp-lang.dev/docs/data-model/operations/queries#implementing-queries). It provides a detailed explanation on how to structure your queries and what the args
and context
parameters are used for.
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------
Sorry @marto, I seem to be having a bit of trouble. Don't worry though, I've pinged my support and they will try to fix me up! :technologist:
Perhaps try again in 15mins?This answer helped me simplify my code but did not solve the problem
I've tested out the answer the bot gave locally and it worked for me. That's the correct way to specify two conditions in Prisma.
Are you sure you don't have some state in React that messes with your requests to the backend?
Log the params you receive on the backend and see if they are what you think they are π
Yeah, pepper that query code on the server with some console.logs, and let's see how many times it is called, with which arguments, and what those prisma calls actually return!