Table Header Actions: How to access data?
I have a RelationManager table, and I am trying to add a quick payment action on it which would create a new PaymentRecord
Trying to figure out how to access PhoneMembership info
->headerActions([
Tables\Actions\Action::make('quick-payment')->label('Quick Payment')
->action(function (PhoneMembership $record, array $data) {
dd($data);
})
->color('success'),
Tables\Actions\CreateAction::make()->label('Add Manual Transaction')->slideOver()->modalWidth('lg'),
])
->headerActions([
Tables\Actions\Action::make('quick-payment')->label('Quick Payment')
->action(function (PhoneMembership $record, array $data) {
dd($data);
})
->color('success'),
Tables\Actions\CreateAction::make()->label('Add Manual Transaction')->slideOver()->modalWidth('lg'),
])
$record->phoneMembershipRecords()->create([
'date' => now(),
'type' => EType::CREDIT,
'value' => $data['value'],
'memo' => 'Quick payment',
]);
$record->phoneMembershipRecords()->create([
'date' => now(),
'type' => EType::CREDIT,
'value' => $data['value'],
'memo' => 'Quick payment',
]);
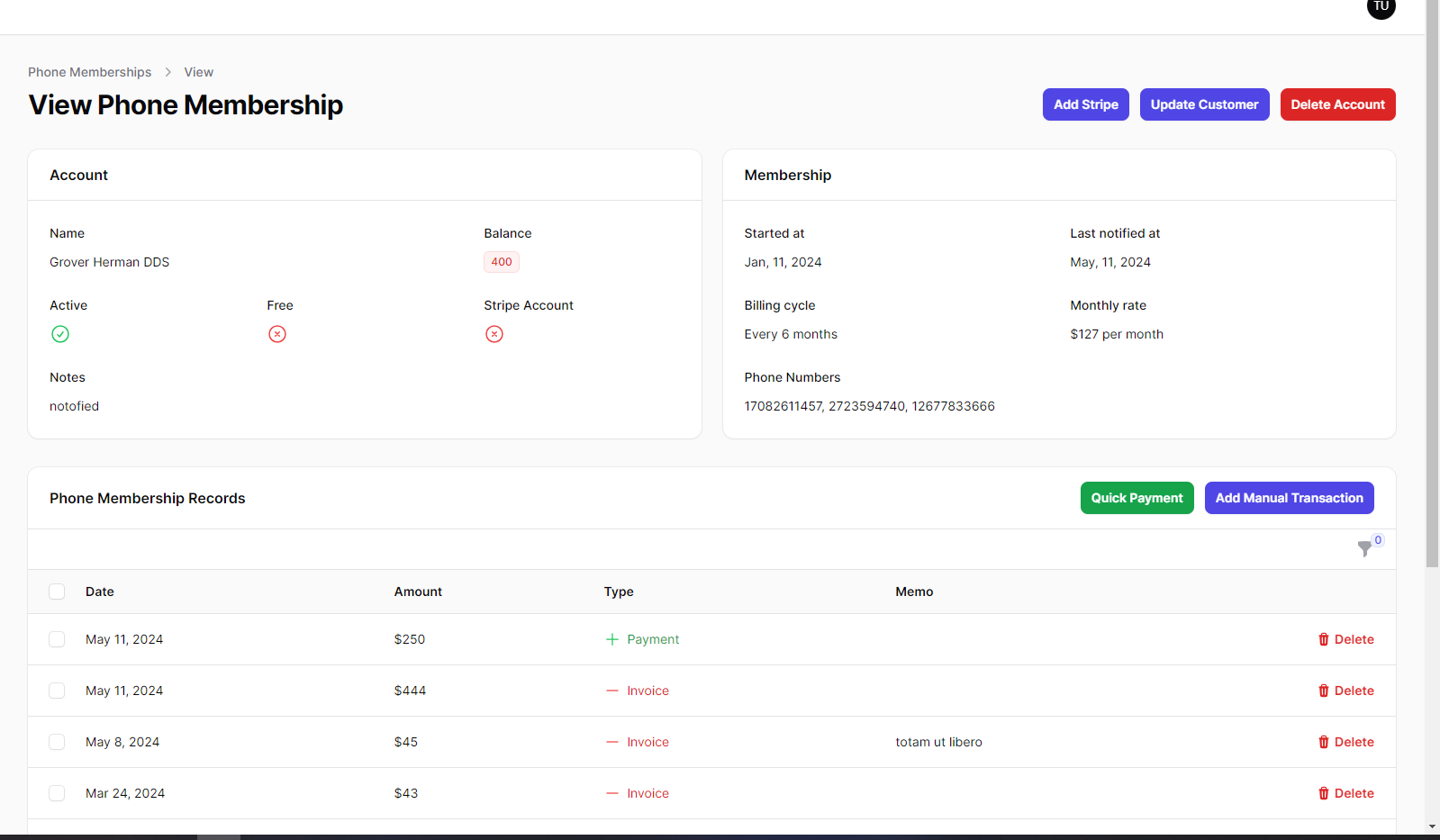
Solution:Jump to solution
```php
Tables\Actions\Action::make('quick-payment')->label('Quick Payment')
->action(function () {
$this->ownerRecord->phoneMembershipRecords()->create([
'date' => now(),...
10 Replies
Im looking at https://filamentphp.com/docs/3.x/actions/advanced and the prebuilt actions, and i cant find an example that will let me dd and see the info
Data is for the form but you don’t have one? What data are you expecting? You already have the record?
im on
This is my relation manager
Trying to find a way to access the parent model somehow 🤔
ViewPhoneMembership
page and it has a relations manager called PhoneMembershipRecordsRelationManager
I am trying to access the PhoneMembership->id, price, and billing cycle so that I can create a transaction w/o the form
public function up(): void
{
Schema::create('phone_memberships', function (Blueprint $table) {
$table->id();
$table->string('name');
$table->json('phones')->nullable();
$table->longText('notes')->nullable();
$table->boolean('is_free')->default(false);
$table->boolean('is_active')->default(true);
$table->boolean('is_subscriber')->default(false);
$table->timestamp('started_at')->nullable();
$table->timestamp('last_notified_at')->nullable();
$table->unsignedBigInteger('monthly_rate')->nullable();
$table->unsignedBigInteger('billing_cycle')->nullable();
$table->softDeletes();
$table->timestamps();
});
}
public function up(): void
{
Schema::create('phone_memberships', function (Blueprint $table) {
$table->id();
$table->string('name');
$table->json('phones')->nullable();
$table->longText('notes')->nullable();
$table->boolean('is_free')->default(false);
$table->boolean('is_active')->default(true);
$table->boolean('is_subscriber')->default(false);
$table->timestamp('started_at')->nullable();
$table->timestamp('last_notified_at')->nullable();
$table->unsignedBigInteger('monthly_rate')->nullable();
$table->unsignedBigInteger('billing_cycle')->nullable();
$table->softDeletes();
$table->timestamps();
});
}
public function up(): void
{
Schema::create('phone_membership_records', function (Blueprint $table) {
$table->id();
$table->foreignId('phone_membership_id')->constrained()->onDelete('cascade');
$table->dateTime('date');
$table->unsignedBigInteger('type')->default(1);
$table->unsignedBigInteger('value');
$table->text('memo')->nullable();
$table->softDeletes();
$table->timestamps();
});
}
public function up(): void
{
Schema::create('phone_membership_records', function (Blueprint $table) {
$table->id();
$table->foreignId('phone_membership_id')->constrained()->onDelete('cascade');
$table->dateTime('date');
$table->unsignedBigInteger('type')->default(1);
$table->unsignedBigInteger('value');
$table->text('memo')->nullable();
$table->softDeletes();
$table->timestamps();
});
}
<?php
namespace App\Filament\Resources\PhoneMembershipResource\RelationManagers;
use Filament\Forms;
use App\Enums\EType;
use Filament\Tables;
use Filament\Forms\Form;
use Filament\Tables\Table;
use App\Models\PhoneMembership;
use Filament\Support\Enums\MaxWidth;
use App\Models\PhoneMembershipRecord;
use Illuminate\Database\Eloquent\Builder;
use Illuminate\Database\Eloquent\SoftDeletingScope;
use Filament\Resources\RelationManagers\RelationManager;
class PhoneMembershipRecordsRelationManager extends RelationManager
{
protected static string $relationship = 'phoneMembershipRecords';
// public static function canViewForRecord(\Illuminate\Database\Eloquent\Model $ownerRecord, string $pageClass): bool
// {
// return $ownerRecord->is_subscriber == false;
// }
public function form(Form $form): Form
{
return $form
->schema([
Forms\Components\DatePicker::make('date')
->helperText('The date of the transaction')
->default(now())
->required()
->columnSpanFull(),
Forms\Components\Radio::make('type')
->options(EType::class)
->helperText('Type of transaction, if its a payment or invoice.')
->default(EType::CREDIT)
->required()
->columns(2)
->columnSpanFull(),
Forms\Components\TextInput::make(name: 'value')
->helperText('Amount paid or owed')
->prefix('$')
->required()
->columnSpanFull(),
Forms\Components\TextInput::make(name: 'memo')
->helperText('Optional transaction notes')
->columnSpanFull(),
]);
}
public function table(Table $table): Table
{
return $table
->recordTitleAttribute('date')
->defaultSort('date', 'desc')
->columns([
Tables\Columns\TextColumn::make('date')->dateTime('M j, Y'),
Tables\Columns\TextColumn::make('value')->label('Amount')->prefix('$'),
Tables\Columns\TextColumn::make('type')->label('Type'),
Tables\Columns\TextColumn::make('memo')->label('Memo'),
])
->filters([
Tables\Filters\TrashedFilter::make()
])
->headerActions([
// Tables\Actions\Action::make('quick-payment')->label('Quick Payment')
// ->action(function (PhoneMembership $record, array $data) {
// $record->phoneMembershipRecords()->create([
// 'date' => now(),
// 'type' => EType::CREDIT,
// 'value' => $data['value'],
// 'memo' => 'Quick payment',
// ]);
// })
// ->color('success'),
Tables\Actions\CreateAction::make()->label('Add Transaction')->slideOver()->modalWidth('lg'),
])
->actions([
// Tables\Actions\EditAction::make(),
Tables\Actions\DeleteAction::make(),
Tables\Actions\ForceDeleteAction::make(),
Tables\Actions\RestoreAction::make(),
])
->bulkActions([
Tables\Actions\BulkActionGroup::make([
Tables\Actions\DeleteBulkAction::make(),
Tables\Actions\RestoreBulkAction::make(),
Tables\Actions\ForceDeleteBulkAction::make(),
]),
])
->modifyQueryUsing(fn (Builder $query) => $query->withoutGlobalScopes([
SoftDeletingScope::class,
]));
}
}
<?php
namespace App\Filament\Resources\PhoneMembershipResource\RelationManagers;
use Filament\Forms;
use App\Enums\EType;
use Filament\Tables;
use Filament\Forms\Form;
use Filament\Tables\Table;
use App\Models\PhoneMembership;
use Filament\Support\Enums\MaxWidth;
use App\Models\PhoneMembershipRecord;
use Illuminate\Database\Eloquent\Builder;
use Illuminate\Database\Eloquent\SoftDeletingScope;
use Filament\Resources\RelationManagers\RelationManager;
class PhoneMembershipRecordsRelationManager extends RelationManager
{
protected static string $relationship = 'phoneMembershipRecords';
// public static function canViewForRecord(\Illuminate\Database\Eloquent\Model $ownerRecord, string $pageClass): bool
// {
// return $ownerRecord->is_subscriber == false;
// }
public function form(Form $form): Form
{
return $form
->schema([
Forms\Components\DatePicker::make('date')
->helperText('The date of the transaction')
->default(now())
->required()
->columnSpanFull(),
Forms\Components\Radio::make('type')
->options(EType::class)
->helperText('Type of transaction, if its a payment or invoice.')
->default(EType::CREDIT)
->required()
->columns(2)
->columnSpanFull(),
Forms\Components\TextInput::make(name: 'value')
->helperText('Amount paid or owed')
->prefix('$')
->required()
->columnSpanFull(),
Forms\Components\TextInput::make(name: 'memo')
->helperText('Optional transaction notes')
->columnSpanFull(),
]);
}
public function table(Table $table): Table
{
return $table
->recordTitleAttribute('date')
->defaultSort('date', 'desc')
->columns([
Tables\Columns\TextColumn::make('date')->dateTime('M j, Y'),
Tables\Columns\TextColumn::make('value')->label('Amount')->prefix('$'),
Tables\Columns\TextColumn::make('type')->label('Type'),
Tables\Columns\TextColumn::make('memo')->label('Memo'),
])
->filters([
Tables\Filters\TrashedFilter::make()
])
->headerActions([
// Tables\Actions\Action::make('quick-payment')->label('Quick Payment')
// ->action(function (PhoneMembership $record, array $data) {
// $record->phoneMembershipRecords()->create([
// 'date' => now(),
// 'type' => EType::CREDIT,
// 'value' => $data['value'],
// 'memo' => 'Quick payment',
// ]);
// })
// ->color('success'),
Tables\Actions\CreateAction::make()->label('Add Transaction')->slideOver()->modalWidth('lg'),
])
->actions([
// Tables\Actions\EditAction::make(),
Tables\Actions\DeleteAction::make(),
Tables\Actions\ForceDeleteAction::make(),
Tables\Actions\RestoreAction::make(),
])
->bulkActions([
Tables\Actions\BulkActionGroup::make([
Tables\Actions\DeleteBulkAction::make(),
Tables\Actions\RestoreBulkAction::make(),
Tables\Actions\ForceDeleteBulkAction::make(),
]),
])
->modifyQueryUsing(fn (Builder $query) => $query->withoutGlobalScopes([
SoftDeletingScope::class,
]));
}
}
https://filamentphp.com/docs/3.x/panels/resources/relation-managers#unconventional-inverse-relationship-names
I gues its called
->inverseRelationship('section')
except I want to use that info to prefil the formHmm thought I was onto something with the attach actions, but stuck in same spot
I found it
dd($this->ownerRecord);
dd($this->ownerRecord);
->headerActions([
Tables\Actions\Action::make('quick-payment')->label('Quick Payment')
->action(function () {
dd($this->ownerRecord);
// $record->phoneMembershipRecords()->create([
// 'date' => now(),
// 'type' => EType::CREDIT,
// 'value' => $data['value'],
// 'memo' => 'Quick payment',
// ]);
})
->headerActions([
Tables\Actions\Action::make('quick-payment')->label('Quick Payment')
->action(function () {
dd($this->ownerRecord);
// $record->phoneMembershipRecords()->create([
// 'date' => now(),
// 'type' => EType::CREDIT,
// 'value' => $data['value'],
// 'memo' => 'Quick payment',
// ]);
})
Solution
Tables\Actions\Action::make('quick-payment')->label('Quick Payment')
->action(function () {
$this->ownerRecord->phoneMembershipRecords()->create([
'date' => now(),
'type' => EType::CREDIT,
'value' => $this->ownerRecord->billing_cycle * $this->ownerRecord->monthly_rate,
'memo' => 'Quick Payment',
]);
})
Tables\Actions\Action::make('quick-payment')->label('Quick Payment')
->action(function () {
$this->ownerRecord->phoneMembershipRecords()->create([
'date' => now(),
'type' => EType::CREDIT,
'value' => $this->ownerRecord->billing_cycle * $this->ownerRecord->monthly_rate,
'memo' => 'Quick Payment',
]);
})
so happy got it working haha