JwtBearerAuthentication
Guys, I'm so damn tired. I can't figure out why my code isn't working, been sitting with it for 12 hours now. I want to create a jwtBearerAuthentication and use it with SwaggerGen(). However, I don't get a header with the Bearer token in the request when I use Swagger (no errors), and when I use Postman to validate this request, I get an authorization header, but the HttpContext.User.Claims will be null. There are no errors and that puts me in a quandary.
49 Replies
$code
To post C# code type the following:
```cs
// code here
```
Get an example by typing
$codegif
in chat
For longer snippets, use: https://paste.mod.gg/
.
You may need to configure your authentication
something like
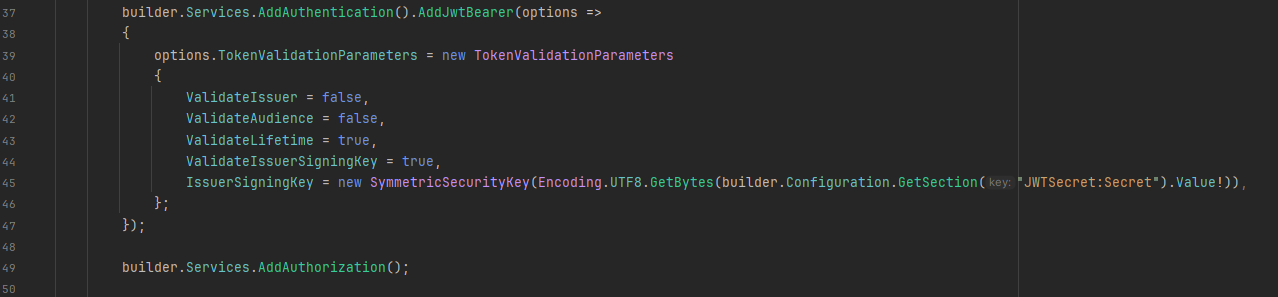
It's not going to work?
what?
I added service with Authentication here
with using JwtBearer
and that isn't doing what the snippet I linked shows
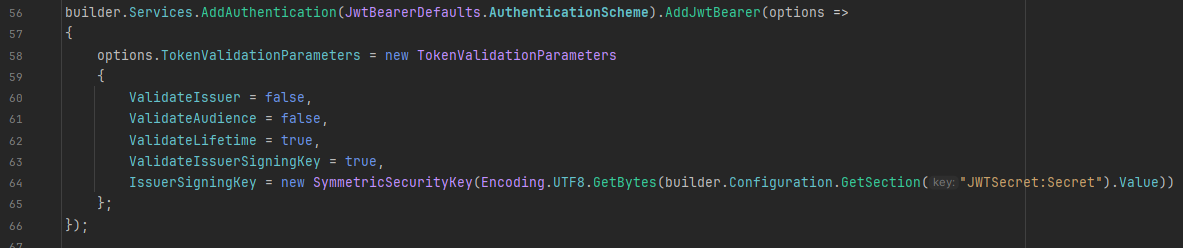
something like that?
give it a try and see what happens
Bearer header exists but HttpContext.User.Claims is null

Well, how are you generating your tokens?
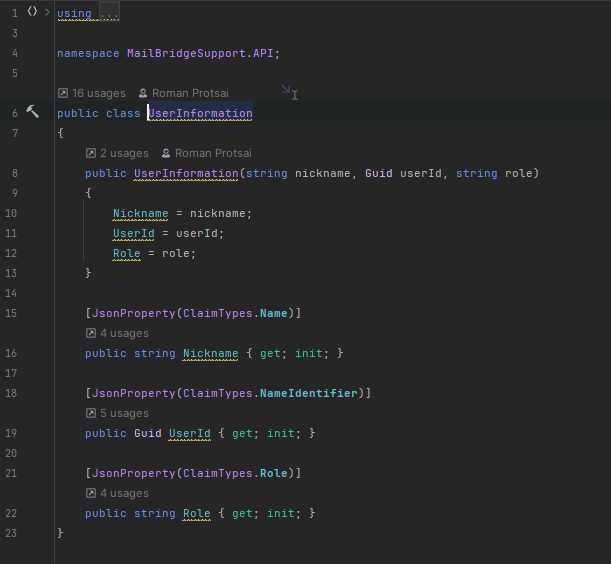
I used User from IdentityUser with Guid maybe it influences in some way
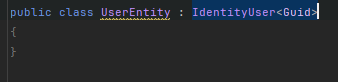
what do you think about it?
What is JwtBuilder from? Don't recognize it
granted I could be using an older version of asp.net core
I'm using .net 8
What do you mean?
I've written JwtHelper to generate this jwtTokens
Ah, I worked directly with
JwtSecurityToken
since it is part of identity (as far as I know)
JwtBuilder
looks to be from https://github.com/jwt-dotnet/jwthttps://github.com/jwt-dotnet/jwt?tab=readme-ov-file#register-authentication-handler-to-validate-jwt
GitHub
GitHub - jwt-dotnet/jwt: Jwt.Net, a JWT (JSON Web Token) implementa...
Jwt.Net, a JWT (JSON Web Token) implementation for .NET - jwt-dotnet/jwt
you should follow their documentation on using the library with asp
but I've used it
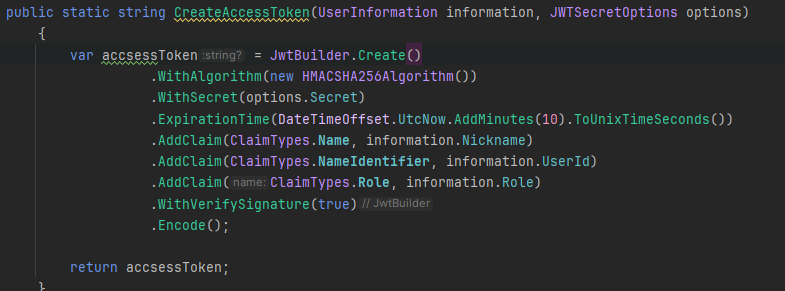
JwtBuilder
Or do you mean something else?
GitHub
GitHub - jwt-dotnet/jwt: Jwt.Net, a JWT (JSON Web Token) implementa...
Jwt.Net, a JWT (JSON Web Token) implementation for .NET - jwt-dotnet/jwt

probably the nuget package for the repo I linked
yea, and I've already used it in my project. I wrote this code 1 day ago
you can see it on this screenshot
And it doesn't work
It is very stange, because there no errors
And I don't know how to debbug it
Well, it doesn't look like you're using all of the configuration options required to make it work with ASP.net. Look at the readme and compare their
ConfigureServices
with yours.Do you mean that I should paste this code to my project? But there is no such commands even for AddJwt. I only have AddJwtBearer

the readme tells you the other dependencies you need
very interesting. I cannot install JWT.Extensions.DependencyInjection in case this error

but JWT.Extentions.AspNetCore I've installed
the best funiest thing is that I should have same versions but I cannot make it because the smallest version is 6 альфа
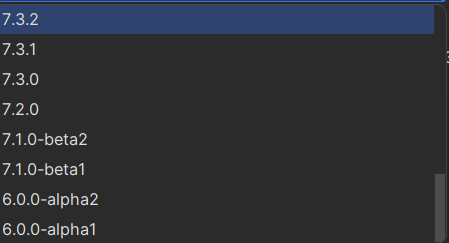
just roll back to the latest released version?
something like that?
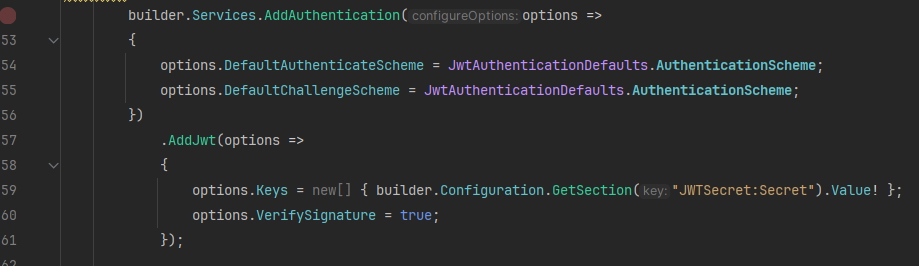
Wouldn't know, never used their library before. Give it a try and see if it works.
nothing changed))
// the non-generic version AddJwt() requires registering an instance of IAlgorithmFactory manually
could be part of your problem, you should really pay attention to what's in that readmeokay
can you show how did you use it?
this is what I use to configure jwt in my application
although I still have a user service that is responsible for generating the tokens
can you show it too, please?
JWTBearer and JWT NuGenPackets work in my previous project, but still don't work in my project I'm working on now
I thought that it does't work because I don't confirm email foreach user but I'd turned off this functions in configuration and it didn't work
Maybe it's because I configured it wrong somehow.
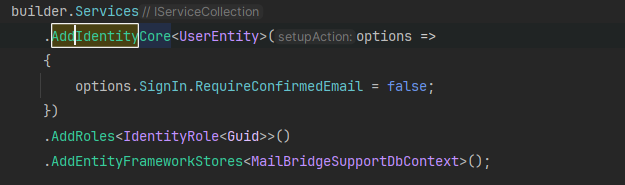
https://stackoverflow.com/questions/55361533/addidentity-vs-addidentitycore
Could be, but I've never used
AddIdentityCore
so I'm not sure what it's missing compared to just AddIdentity
Stack Overflow
AddIdentity vs AddIdentityCore
In ASP.NET Core, you can add various services for identification: AddDefaultIdentity, AddIdentity and AddIdentityCore.
What's the difference between AddIdentity and AddIdentityCore?
Is this endpoint for authorized only?
If not, it ignores token validation and stuff
I dont reallu remember exact function, but if your endpoint allows anonymous you have to call something HttpContext.Authenticate... to authorize and get your user info